Avoiding Pitfalls: Querying DynamoDB with Java Simplified
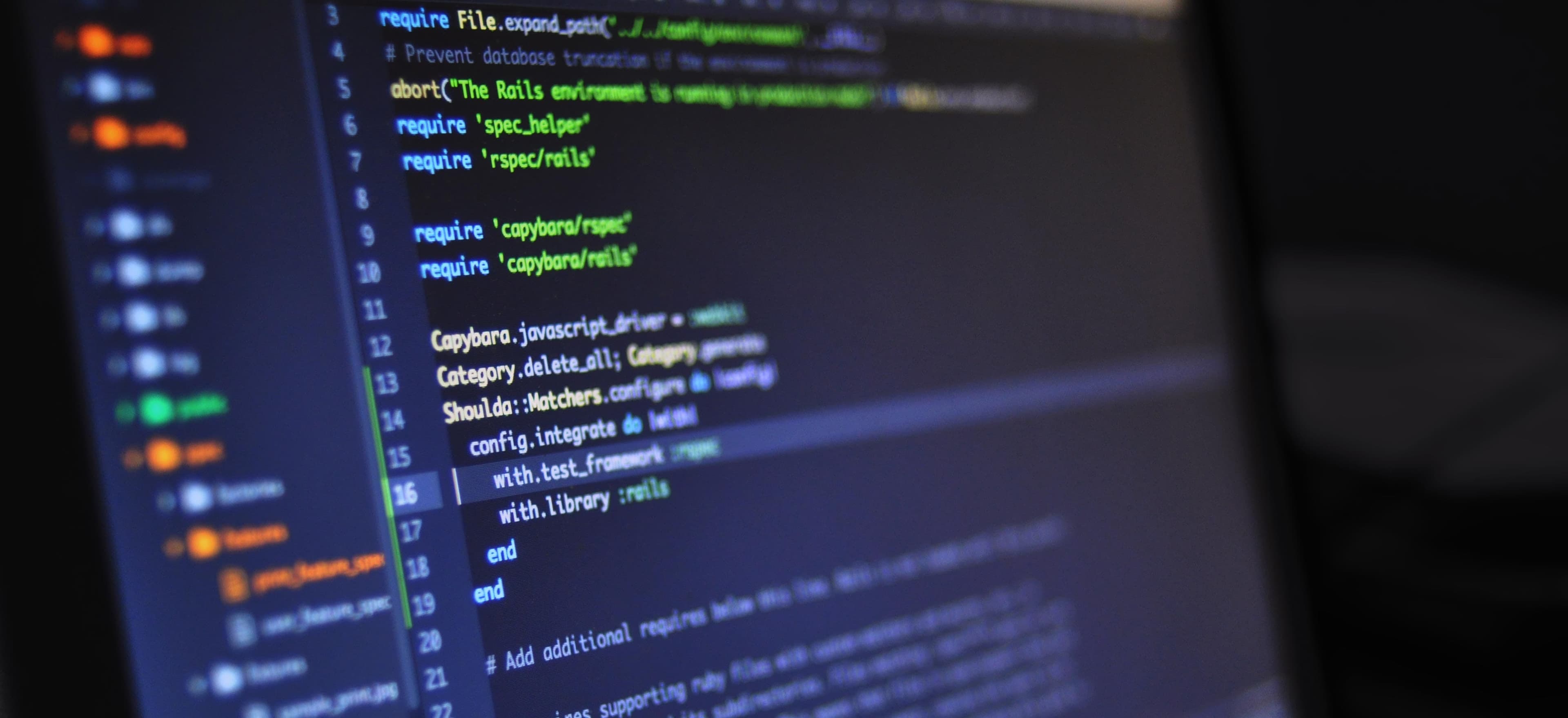
- Published on
Avoiding Pitfalls: Querying DynamoDB with Java Simplified
Navigating the intricacies of querying Amazon DynamoDB with Java can feel akin to untangling a complex web. However, with the right approach and understanding, it transforms into a seamless and efficient process. This blog post aims to demystify the process of querying DynamoDB using Java, ensuring you sidestep common pitfalls and harness the full power of this NoSQL database service. We'll provide code snippets to illustrate points clearly, accompanied by discussions on 'why' certain approaches are beneficial.
Understanding DynamoDB and Its Java SDK
Amazon DynamoDB, a fully managed NoSQL database service, offers fast and predictable performance with seamless scalability. It allows for the storage and retrieval of any amount of data, serving as a perfect fit for web-scale applications. The Java SDK for DynamoDB simplifies interacting with the database in Java applications, but it requires a nuanced understanding to avoid common mistakes.
First, familiarize yourself with the DynamoDB documentation (Amazon DynamoDB documentation) to grasp its core concepts, including tables, items, attributes, primary keys, indexes, and the various types of queries and scans.
Setting Up Your Java Environment
Before diving into querying, ensure your Java environment is set up correctly. This includes having the AWS SDK for Java correctly configured in your project. You can add it to your Maven project by including the following dependency in your pom.xml
:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-dynamodb</artifactId>
<version>LATEST_VERSION</version>
</dependency>
Replace LATEST_VERSION
with the latest version number of the SDK.
Diving into Querying
Querying in DynamoDB is primarily done using two methods: query
and scan
. While scan
reads every item in a table or a secondary index, query
performs a more efficient and targeted search by using a specific key value. For optimal performance and cost-effectiveness, prefer queries over scans whenever possible.
Crafting a Simple Query
Consider a scenario where you have a Users
table with a primary key named userId
. To fetch a user with a specific userId
, your query would look something like this:
AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build();
DynamoDB dynamoDB = new DynamoDB(client);
Table table = dynamoDB.getTable("Users");
QuerySpec spec = new QuerySpec()
.withKeyConditionExpression("userId = :v_id")
.withValueMap(new ValueMap()
.withString(":v_id", "12345"));
ItemCollection<QueryOutcome> items = table.query(spec);
for (Item item : items) {
System.out.println(item.toJSONPretty());
}
This code snippet highlights the use of QuerySpec
to define the query conditions. We specify that we're looking for items where userId
matches a specific value, which makes our query efficient and targeted.
Avoiding Common Pitfalls
When querying DynamoDB, there are several common pitfalls to be aware of:
-
Over-reliance on Scan Operations: As mentioned earlier, scans are less efficient than queries because they read every item in the table. Always look for opportunities to use queries instead.
-
Not Using Indexes Effectively: Secondary indexes can significantly speed up queries. However, they need to be designed thoughtfully, considering your query patterns. (Choosing the Right DynamoDB Partition Key).
-
Ignoring Pagination: DynamoDB queries return a maximum of 1 MB of data in a single operation. For larger datasets, implement pagination to handle the complete result set.
-
Overlooking Query Limitations: DynamoDB has specific limits, such as the 1 MB size limit for query results and the limit on the number of items that can be updated in a single request. Be sure to design your applications with these constraints in mind.
Enhancing Queries with Secondary Indexes
Secondary indexes are a powerful feature that allows for more flexible querying. There are two types of secondary indexes in DynamoDB: Global Secondary Indexes (GSI) and Local Secondary Indexes (LSI). Here's how you can leverage a GSI in a query:
Index index = table.getIndex("SomeGlobalSecondaryIndex");
QuerySpec spec = new QuerySpec()
.withKeyConditionExpression("someGSIKey = :v_key")
.withValueMap(new ValueMap().withString(":v_key", "keyValue"));
ItemCollection<QueryOutcome> items = index.query(spec);
for (Item item : items) {
System.out.println(item.toJSONPretty());
}
In this example, querying a GSI allows us to perform efficient queries on non-primary key attributes. This flexibility can significantly enhance your application's performance and scalability.
Best Practices for Effective Querying
To conclude, here are some best practices for querying DynamoDB with Java:
- Understand Your Access Patterns: Design your table and indexes based on how your application will access the data.
- Use Batch Operations Wisely: While DynamoDB supports batch operations, they should be used judiciously to avoid throttling.
- Monitor Performance and Costs: Keep an eye on your DynamoDB metrics and costs. Optimizing your queries can lead to significant savings and performance improvements.
Conclusion
Querying DynamoDB with Java is a powerful way to interact with your NoSQL data. By following the guidelines and best practices outlined in this post, you can avoid common pitfalls and build efficient, scalable applications. Remember, the key to success with DynamoDB lies in understanding its unique characteristics and leveraging its capabilities to your advantage.
Resources
- AWS SDK for Java DynamoDB Documentation: AWS SDK for Java
- Amazon DynamoDB Best Practices: Best Practices for Designing and Architecting with DynamoDB
By mastering the art of querying DynamoDB with Java, you unlock the potential to create robust backend systems capable of handling the demands of modern web and mobile applications. Happy coding!
Checkout our other articles