From Chat to Code: Overcoming Procrastination in Programming
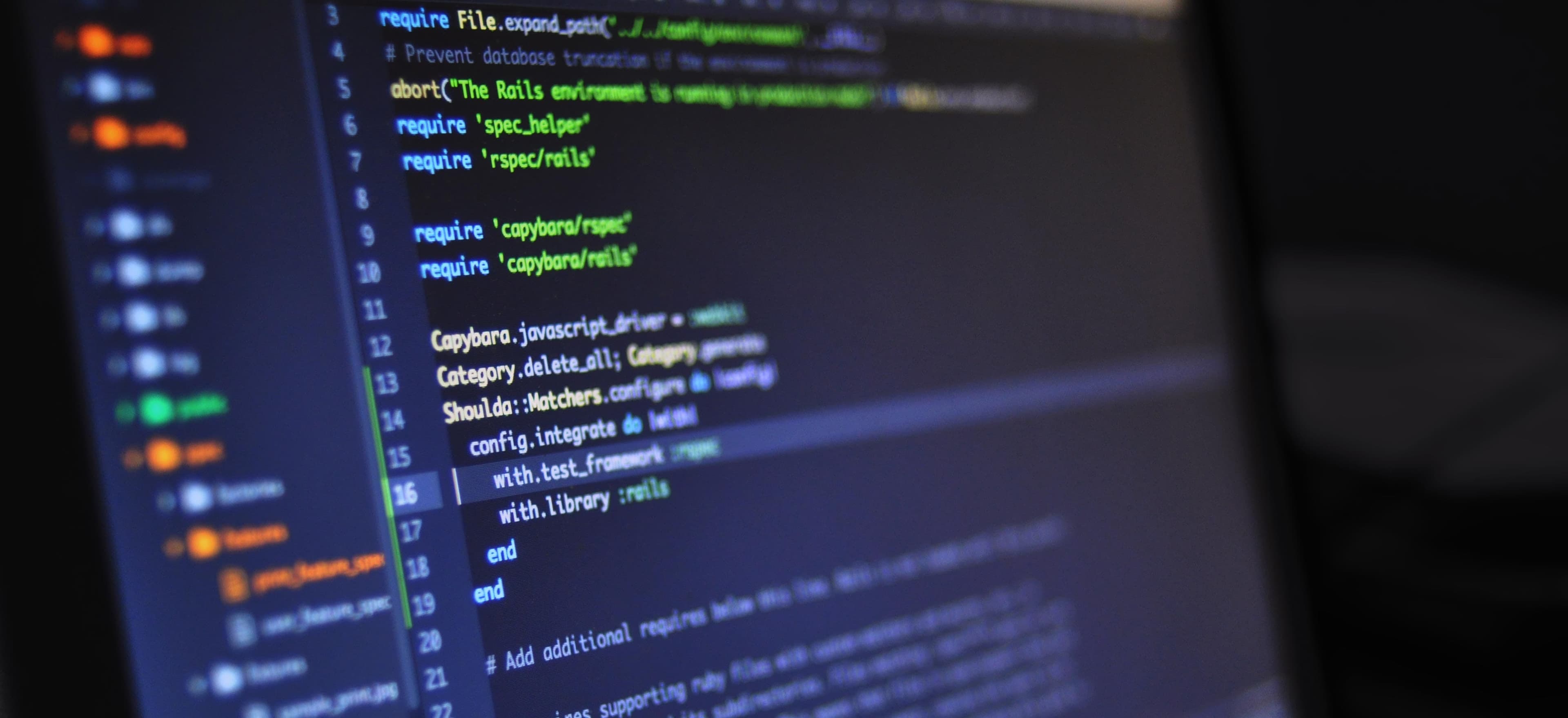
- Published on
Overcoming Procrastination in Programming: From Chat to Code
Procrastination is a common issue that almost all of us face at some point in our lives. When it comes to programming, the temptation to procrastinate can be particularly strong. Whether it's due to the complexity of the task at hand, a lack of motivation, or simply feeling overwhelmed, procrastination can hinder progress and stifle creativity. In this article, we'll explore some practical strategies to overcome procrastination in programming and transform that chat about coding into actual code.
Understanding Procrastination
Before delving into strategies to overcome procrastination in programming, it's essential to understand why we procrastinate in the first place. Procrastination often stems from a combination of factors, including fear of failure, perfectionism, lack of clear goals, and a tendency to prioritize immediate gratification over long-term benefits.
In the context of programming, fear of failure can manifest as a reluctance to tackle complex problems or start working on a challenging project. Additionally, the pursuit of perfect code and the desire to avoid making mistakes can lead to procrastination.
Breaking It Down: The Power of Small Steps
One effective approach to overcoming procrastination in programming is to break down tasks into smaller, more manageable steps. This can help alleviate the feeling of being overwhelmed by a large project or a complex coding problem. By breaking the task into smaller subtasks, you can create a roadmap for your progress and tackle each component one at a time.
Let's consider an example of breaking down a larger coding task into smaller steps. Suppose you're tasked with building a web application with multiple features. Instead of viewing the entire project as a daunting task, you can break it down into stages such as setting up the project structure, designing the user interface, implementing backend functionality, and integrating external APIs. Each of these stages can be further broken down into specific tasks, such as creating individual components, handling user input, or testing API endpoints.
// Example of breaking down a web application project into smaller steps
public class WebApplication {
public void setupProjectStructure() {
// Code for setting up project structure
}
public void designUserInterface() {
// Code for designing the user interface
}
public void implementBackendFunctionality() {
// Code for implementing backend functionality
}
public void integrateExternalAPIs() {
// Code for integrating external APIs
}
}
By breaking down the tasks and implementing them one by one, you can make steady progress while mitigating the overwhelming feeling that often leads to procrastination.
Setting Clear Goals and Deadlines
Without clear goals and deadlines, it's easy to succumb to procrastination. In the world of programming, setting specific, achievable goals and deadlines can provide a sense of structure and urgency, motivating you to start working on your code.
Consider establishing daily or weekly goals for your programming tasks. These goals could include completing a specific function, refactoring a module, or resolving a particular bug. Setting deadlines, whether self-imposed or project-based, adds a layer of accountability and helps enforce a sense of urgency.
// Setting a deadline for completing a specific task
public class TaskDeadline {
public void completeTaskByDeadline(Date deadline) {
// Code to track task completion by the given deadline
}
}
Additionally, leveraging project management tools such as Jira, Trello, or Asana can assist in setting and tracking goals and deadlines, allowing for a more organized approach to programming tasks.
Embracing Imperfection: The Iterative Approach
Perfectionism often fuels procrastination in programming. The pursuit of flawless, impeccable code can lead to paralysis, as the fear of making mistakes or writing suboptimal code grips the programmer. Embracing an iterative approach to programming can help overcome this barrier.
Instead of striving for perfect code from the outset, adopt an iterative mindset. Start by writing code that accomplishes the immediate task at hand, even if it's not the most elegant or optimized solution. Through iteration and incremental improvements, you can refine and enhance the code over time.
// Embracing an iterative approach to programming
public class IterativeProgramming {
public void iterativeRefinement() {
// Code that accomplishes the immediate task
// Iterative improvements and refinements over time
}
}
The iterative approach allows you to make progress without being bound by the pressure of perfection. As you receive feedback and gain a deeper understanding of the problem domain, you can iteratively enhance your code, leading to more robust and efficient solutions.
Leveraging the Pomodoro Technique
The Pomodoro Technique is a time management method that can be particularly beneficial for combating procrastination. The technique involves breaking work into intervals, typically 25 minutes long, separated by short breaks. This structured approach can help maintain focus and productivity while mitigating the urge to procrastinate.
When applying the Pomodoro Technique to programming, allocate a Pomodoro session to a specific programming task, such as debugging a section of code, implementing a new feature, or writing unit tests. Setting a timer and committing to focused work during each interval can promote a sense of accomplishment and momentum, reducing the likelihood of procrastination.
// Applying the Pomodoro Technique to programming
public class PomodoroProgramming {
public void applyPomodoroForTask(int taskDurationInMinutes) {
// Code to track and manage work intervals using the Pomodoro Technique
// Focus on the programming task during the allocated time interval
}
}
By incorporating the Pomodoro Technique into your programming routine, you can carve out dedicated, uninterrupted periods for coding, thereby minimizing distractions and boosting productivity.
Seeking Support and Collaboration
Programming can often feel solitary, which can contribute to feelings of isolation and, consequently, procrastination. Seeking support from peers, mentors, or online communities can offer valuable perspectives, guidance, and accountability, helping you stay motivated and committed to your programming endeavors.
Engaging in pair programming or code reviews with colleagues can provide opportunities for collaborative problem-solving and knowledge sharing. Additionally, participating in programming forums, attending meetups, or joining online communities such as Stack Overflow or GitHub can facilitate interactions with fellow developers, providing a sense of community and fostering a supportive environment.
// Leveraging collaboration for programming support
public class CollaborationProgramming {
public void pairProgramming() {
// Collaborative coding session with a colleague
}
public void codeReview() {
// Participating in code reviews for mutual feedback and improvement
}
}
Collaboration not only enriches your programming skills but also combats the sense of isolation that often accompanies procrastination, as you benefit from shared experiences and diverse insights.
Closing the Chapter
Overcoming procrastination in programming requires a combination of proactive strategies and mindset shifts. By breaking down tasks, setting clear goals and deadlines, embracing imperfection, leveraging time management techniques, and seeking support and collaboration, you can effectively navigate the challenges of procrastination and transform your coding aspirations into tangible code.
Remember, the journey from procrastination to productivity is a continuous learning process, and each small step taken toward overcoming procrastination brings you closer to unleashing your full programming potential. So, put the procrastination aside, and let's turn that chat about coding into meaningful code!
Now, it's time to transition from chat to code!