Overcoming EclipseLink JPA-RS Integration Challenges
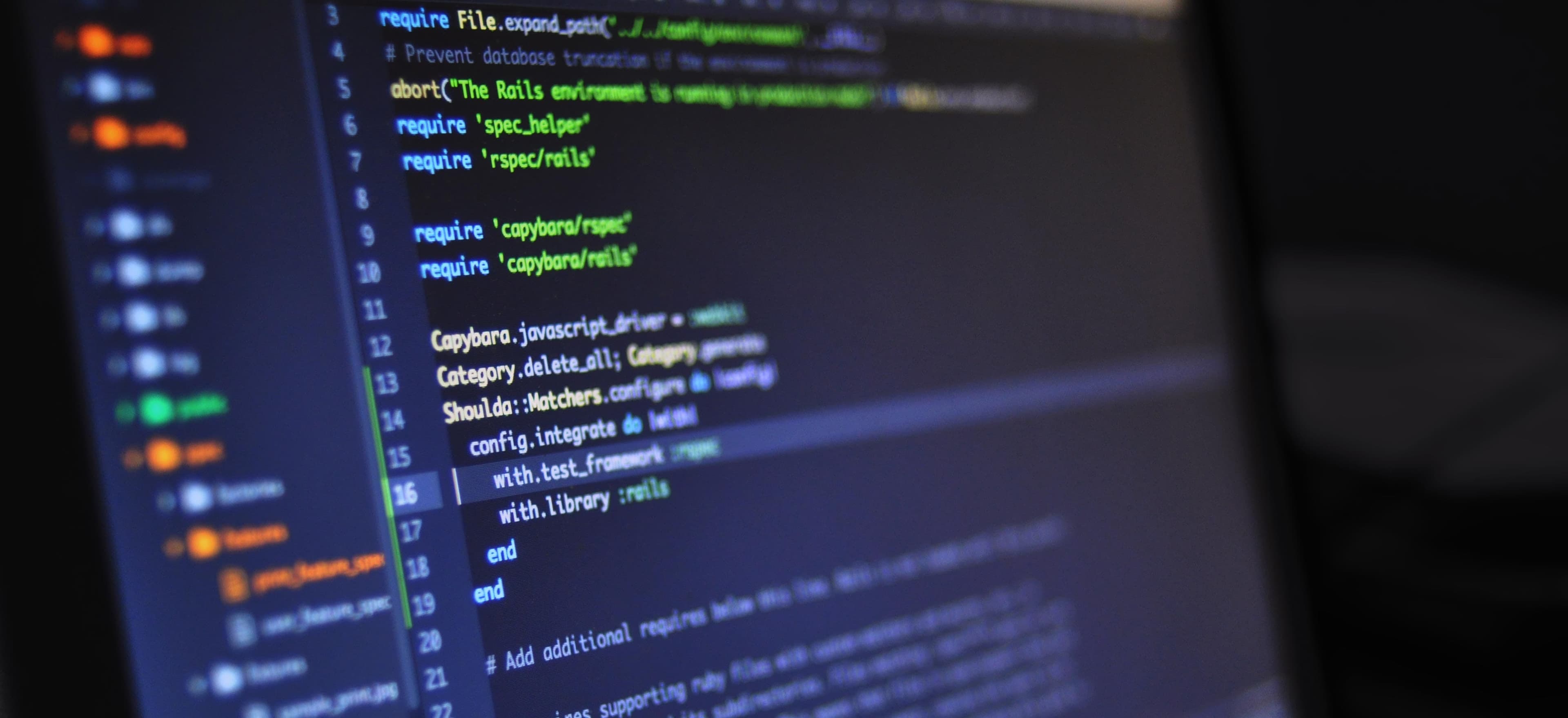
- Published on
Overcoming EclipseLink JPA-RS Integration Challenges
When working with Java applications that utilize JPA (Java Persistence API) for database access and EclipseLink as the JPA provider, you may encounter challenges when integrating JPA-RS for RESTful services. This blog post will discuss common issues faced during this integration and provide solutions to overcome them.
What is JPA-RS?
JPA-RS is a component of EclipseLink that allows for the creation of RESTful services from JPA entities. It enables developers to expose JPA entities as REST resources, providing a straightforward way to interact with the data in a RESTful manner.
Common Integration Challenges
Challenge 1: Configuration
One of the initial challenges developers face is configuring the JPA-RS functionality within the EclipseLink setup. This involves setting up proper dependencies and ensuring that the JPA-RS component is correctly integrated with the existing JPA and EclipseLink setup.
Solution:
To address this challenge, ensure that the necessary dependencies for JPA-RS are included in the project's build path or dependency management tool such as Maven or Gradle. Additionally, configure the persistence.xml
file to enable JPA-RS support by including the following property:
<property name="eclipselink.weaving" value="true"/>
<property name="eclipselink.weaving.rest" value="true"/>
The eclipselink.weaving
property enables bytecode weaving, while eclipselink.weaving.rest
specifically enables weaving for JPA-RS support.
Challenge 2: Entity-to-JSON Conversion
Another common challenge is the conversion of JPA entities to JSON when exposing them as REST resources. This is crucial for ensuring that the data is represented in a format suitable for RESTful interactions.
Solution:
EclipseLink provides the @XmlRootElement
annotation to mark JPA entities for XML/JSON conversion. It works seamlessly with JPA-RS to serialize JPA entities into JSON format. Simply annotate the JPA entity classes with @XmlRootElement
to enable JSON serialization.
Here's an example:
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Book {
// entity attributes and methods
}
Challenge 3: Resource Path Configuration
Defining the resource paths for the exposed JPA entities can be a challenging aspect of integrating JPA-RS. Mapping the entities to specific URI paths requires careful configuration to ensure proper RESTful access.
Solution:
Use the @EntityRest
annotation provided by EclipseLink to specify the base URI path for the JPA entities. By annotating the JPA entity classes with @EntityRest
, you can define the resource path for the corresponding entity.
Here's an example:
import org.eclipse.persistence.oxm.annotations.XmlPath;
@EntityRest(path = "books")
@XmlRootElement
public class Book {
// entity attributes and methods
}
In this example, the @EntityRest
annotation specifies the base URI path for the Book
entity as "books".
Additional Tips for Smooth Integration
Leverage EclipseLink Documentation
EclipseLink offers comprehensive documentation that details the configuration and usage of JPA-RS. Refer to the official documentation for in-depth information on setting up and utilizing JPA-RS within your EclipseLink-based application.
Error Handling and Logging
Implement robust error handling and logging within your JPA-RS RESTful services. Proper exception handling and logging mechanisms can enhance the maintainability and reliability of your RESTful endpoints.
Keep Dependencies Updated
Ensure that you are using the latest compatible versions of EclipseLink and related libraries. Keeping dependencies updated can prevent compatibility issues and leverage the latest features and bug fixes.
Closing the Chapter
Integrating JPA-RS with EclipseLink for RESTful services can be challenging, but with proper configuration and understanding of the key components, these challenges can be overcome. By leveraging the solutions and tips discussed in this post, developers can streamline the integration process and effectively expose JPA entities as REST resources.
In summary, tackling the configuration, entity-to-JSON conversion, and resource path definition challenges, while leveraging additional tips, can lead to a successful integration of JPA-RS within your Java application powered by EclipseLink. For further insights and detailed information, refer to the EclipseLink documentation and explore the rich capabilities of JPA-RS.