Dynamic Template Generation with MVEL: Transforming Static Configurations
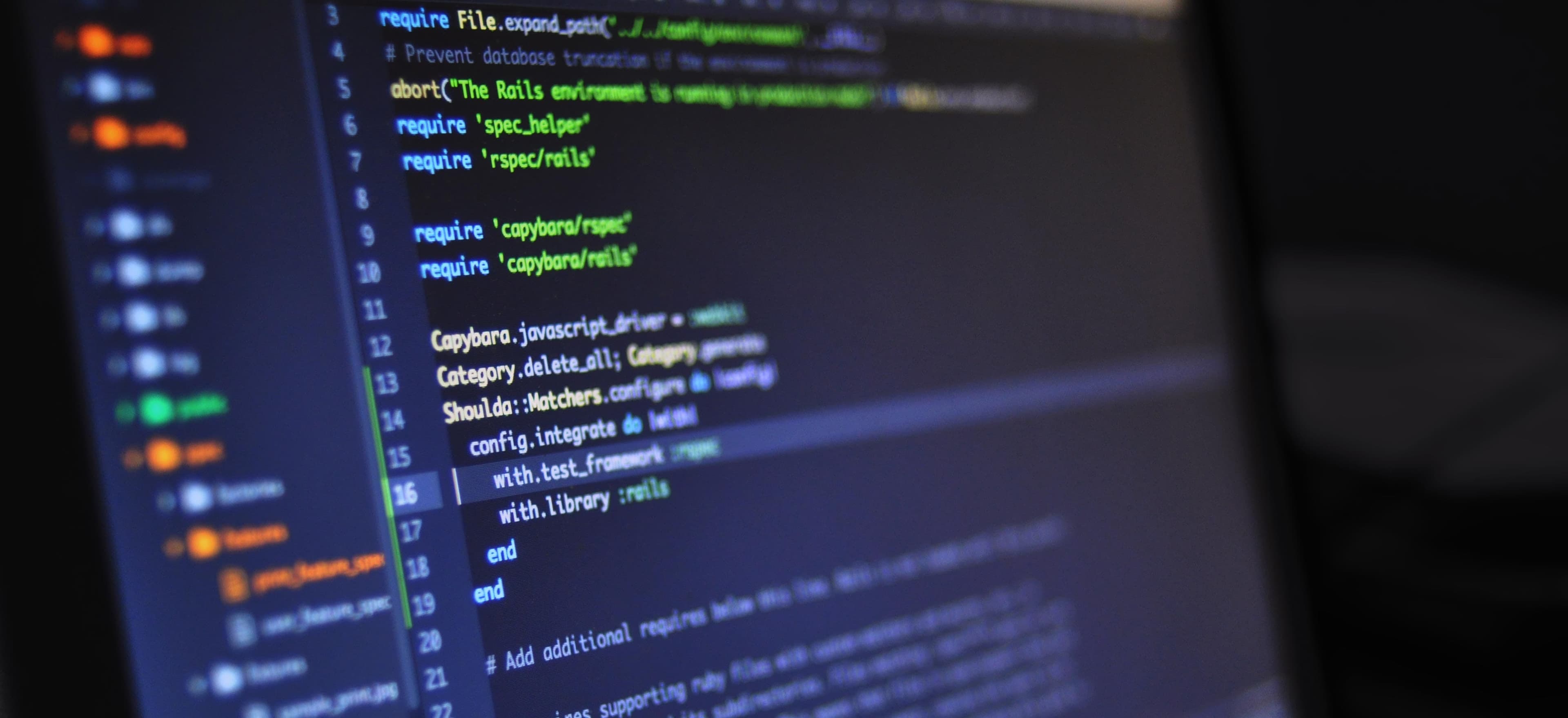
- Published on
Dynamic Template Generation with MVEL: Transforming Static Configurations
In the world of software development, dynamic template generation plays a pivotal role in transforming static configurations into dynamic and flexible solutions. Java developers often face the challenge of efficiently creating, manipulating, and customizing templates to cater to varying requirements. To address this challenge, MVEL (MVFlex Expression Language) emerges as a powerful and versatile tool that enables the generation of dynamic templates in Java applications.
Understanding MVEL
MVEL is a lightweight and powerful expression language for Java-based applications. It provides an intuitive syntax for embedding expressions within templates, making it ideal for dynamic template generation. MVEL is capable of directly evaluating expressions, simplifying complex logic, and seamlessly integrating with Java objects. Its ease of use and flexibility make it suitable for a wide range of use cases, including dynamic document generation, configuration file processing, and business rule evaluation.
Incorporating MVEL
To incorporate MVEL into a Java project, add the MVEL dependency to the project’s build configuration file, such as Maven's pom.xml:
<dependency>
<groupId>org.mvel</groupId>
<artifactId>mvel2</artifactId>
<version>2.4.11.Final</version>
</dependency>
Once the dependency is added, MVEL is ready to be utilized for dynamic template generation within the application.
Dynamic Template Generation
Dynamic template generation with MVEL involves creating template files with embedded expressions that can be evaluated and resolved at runtime. Templates can be designed using a simple syntax that includes placeholders for dynamic values. Let’s explore an example of a dynamic template using MVEL:
Consider a template file named invoice_template.mvel
with the following content:
Invoice Number: ${invoice.number}
Date: ${invoice.date}
Total Amount: ${invoice.totalAmount}
In this template, the expressions wrapped in ${}
denote placeholders that will be dynamically replaced with actual values at runtime.
Dynamic Template Evaluation
To evaluate the dynamic template and populate it with actual data, MVEL provides a straightforward mechanism. By leveraging MVEL’s TemplateRuntime
class, the template file can be loaded, and the placeholders can be resolved using a context object that contains the actual dynamic values. Below is a code snippet demonstrating dynamic template evaluation with MVEL:
import org.mvel2.templates.TemplateRuntime;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.Map;
public class InvoiceGenerator {
public static void main(String[] args) throws IOException {
String templateContent = new String(Files.readAllBytes(Paths.get("invoice_template.mvel")));
Map<String, Object> context = new HashMap<>();
context.put("invoice", new Invoice("INV-001", "2023-10-15", 250.00));
String populatedTemplate = (String) TemplateRuntime.eval(templateContent, context);
System.out.println(populatedTemplate);
}
}
class Invoice {
private String number;
private String date;
private double totalAmount;
public Invoice(String number, String date, double totalAmount) {
this.number = number;
this.date = date;
this.totalAmount = totalAmount;
}
// Getters and setters
}
In this example, the InvoiceGenerator
class loads the invoice_template.mvel
file, creates a context map with dynamic data, and utilizes MVEL’s TemplateRuntime.eval
to populate the template and obtain the final output.
Advantages of Dynamic Template Generation with MVEL
Dynamic template generation with MVEL offers several advantages:
- Flexibility: MVEL allows for the creation of highly dynamic and adaptable templates, catering to diverse use cases without the need for extensive code changes.
- Separation of Concerns: Templates separate the presentation layer from the data, promoting a clean and organized code structure.
- Expression Evaluation: MVEL’s support for complex expression evaluation facilitates the incorporation of business logic directly within the templates.
- Ease of Maintenance: With MVEL, templates can be easily maintained, modified, and extended, enhancing the overall maintainability of the application.
In Conclusion, Here is What Matters
Incorporating MVEL for dynamic template generation empowers Java developers to efficiently transform static configurations into dynamic, adaptable, and expressive templates. The seamless integration of MVEL within Java applications, combined with its intuitive syntax and powerful capabilities, makes it a valuable asset for addressing the complexities of template-based dynamic content generation.
By employing MVEL, developers can unleash the potential of dynamic templates, streamline the generation of complex documents, and enhance the overall flexibility of their applications, contributing to a more robust and agile software development process.
Utilizing MVEL for dynamic template generation offers Java developers a powerful and versatile solution to transform static configurations into dynamic and flexible templates. Leveraging MVEL enables developers to create, manipulate, and customize templates efficiently, catering to diverse requirements and use cases. This detailed guide explores the integration of MVEL, dynamic template creation, evaluation, and the advantages of employing MVEL for dynamic template generation.
Incorporating MVEL within a Java project is a straightforward process, requiring the addition of the MVEL dependency to the project’s build configuration. Once integrated, MVEL facilitates dynamic template generation by allowing developers to embed expressions within templates and resolve placeholders at runtime.
MVEL’s seamless integration within Java applications, coupled with its intuitive syntax and powerful capabilities, positions it as a valuable tool for addressing the complexities of dynamic template generation. Through the utilization of MVEL, developers can streamline the generation of complex documents, enhance application flexibility, and promote a more robust software development process.