Avoid These Classic Software Development Blunders!
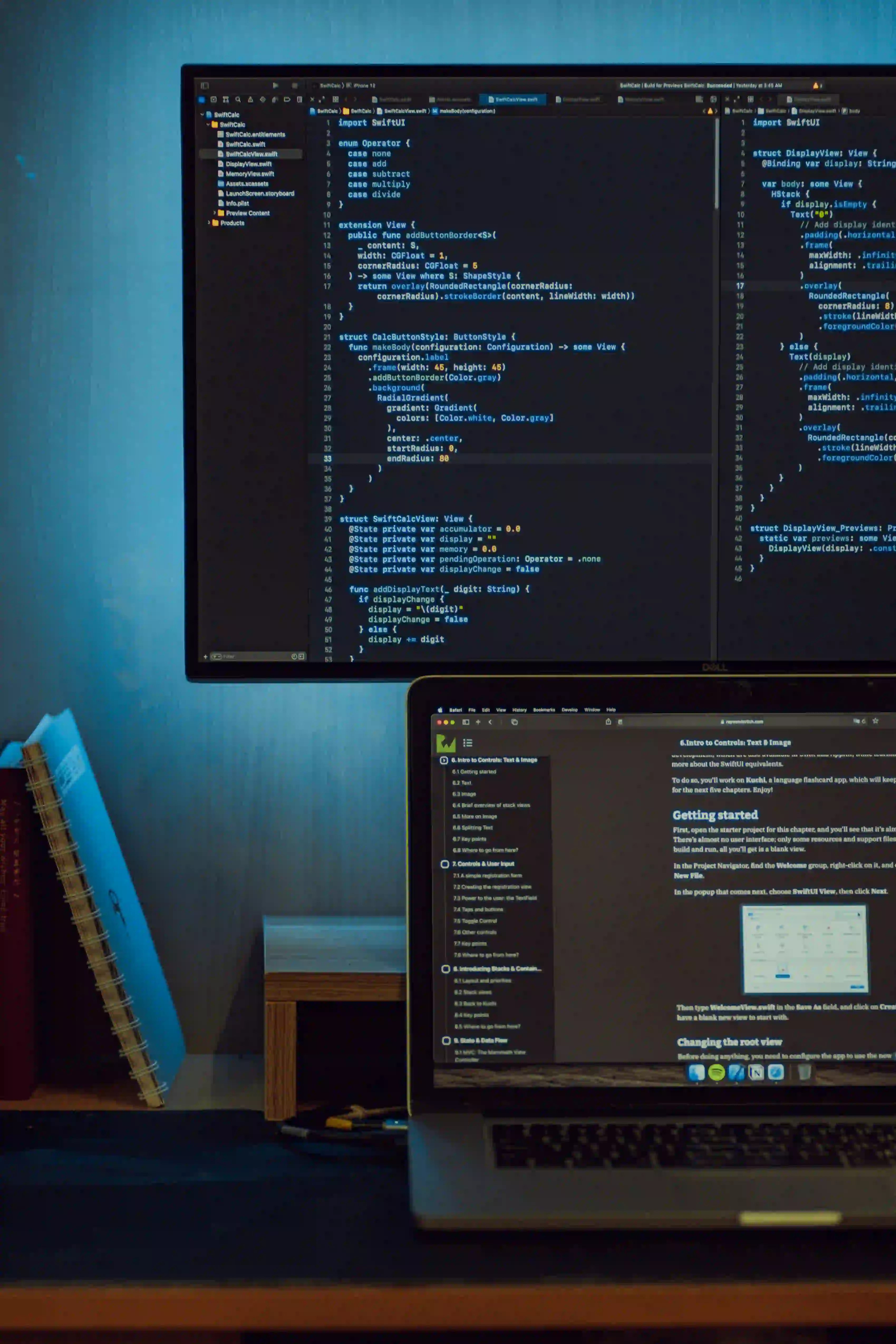
The Costly Pitfalls of Classic Java Development Blunders
In the fast-paced world of software development, steering clear of common mistakes is paramount. As a Java developer, understanding and sidestepping classic blunders can save time, resources, and headaches down the road. Let's delve into some of these pitfalls and explore why they should be avoided at all costs.
Neglecting Exception Handling
One of the classic blunders in Java development is neglecting proper exception handling. Failing to handle exceptions can lead to unexpected program termination and potential data loss. It's crucial to anticipate potential errors and handle them gracefully through try-catch blocks.
try {
// Risky code that might throw an exception
} catch (SomeException e) {
// Handle the exception or log it
}
Why: Exception handling ensures that your application can gracefully recover from errors, enhancing its reliability and robustness.
Ignoring Performance Optimization
Overlooking performance optimization is another stumbling block. Java developers often focus solely on functionality without considering the efficiency of their code. Utilizing tools like profilers and writing efficient algorithms can significantly enhance the performance of Java applications.
Why: Optimized code leads to faster execution, lower resource consumption, and an overall smoother user experience.
Forgoing Unit Testing
Neglecting unit testing can have dire consequences. Writing code without accompanying unit tests can result in undetected bugs and make it challenging to refactor or modify existing code. Embracing test-driven development and writing comprehensive unit tests is crucial for building reliable and maintainable Java applications.
@Test
public void testSomeFunctionality() {
// Test the functionality and assert expected behavior
}
Why: Unit tests serve as a safety net, providing confidence in the code's correctness and facilitating seamless code changes and enhancements.
Neglecting Code Documentation
Failing to document code comprehensively is a detriment to both the current and future developers working on the codebase. Inadequate comments and documentation make it arduous to understand the logic, purpose, and usage of various components within the application.
Why: Clear and concise documentation enhances code maintainability, aids collaboration, and reduces the learning curve for new developers joining the project.
Overlooking Security Vulnerabilities
Disregarding security best practices is a critical blunder in Java development. Failing to sanitize inputs, using outdated libraries, or neglecting proper access control can expose the application to security vulnerabilities. Embracing secure coding practices and staying updated on potential threats is paramount.
Why: Prioritizing security safeguards the application against potential exploits, data breaches, and regulatory non-compliance.
Relying Excessively on Static Methods and Variables
Excessive reliance on static methods and variables can hinder the testability and maintainability of code. While static elements have their use cases, overuse can lead to tightly coupled and hard-to-test code. Embracing object-oriented principles and dependency injection can alleviate these issues.
Why: Proper usage of object-oriented principles leads to code that is easier to test, maintain, and extend, promoting a more robust and adaptable codebase.
Disregarding Code Reviews and Collaboration
Failing to engage in regular code reviews and disregarding collaboration with team members can impede the overall quality of the codebase. Code reviews foster knowledge sharing, catch potential issues early, and enhance the overall quality of the code through constructive feedback.
Why: Code reviews and collaboration cultivate a culture of shared ownership, continuous improvement, and adherence to coding standards within the development team.
Ignoring Memory Leaks and Garbage Collection
Neglecting to address memory leaks and disregarding the behavior of the garbage collector can result in performance degradation and application instability over time. Profiling the application for memory leaks and understanding the nuances of garbage collection is crucial for maintaining the application's health.
Why: Addressing memory leaks and understanding garbage collection behavior promotes optimal memory utilization and overall application stability.
Lessons Learned
In the realm of Java development, steering clear of classic blunders is integral to building robust, efficient, and maintainable applications. By prioritizing exception handling, performance optimization, unit testing, code documentation, security, object-oriented principles, collaboration, and memory management, Java developers can elevate the quality and reliability of their codebases. Awareness of these blunders and a proactive approach to mitigating them are hallmarks of seasoned Java developers.
Embracing best practices and continually refining development skills is essential for navigating the complex terrain of Java software development. By evading these classic blunders and integrating best practices, Java developers can carve a path towards success in their development endeavors.
Remember, vigilance against classic blunders sets the stage for crafting exceptional Java applications. Happy coding!
Disclaimer: This article is intended for educational purposes and does not guarantee the prevention of all potential software development blunders.