Master Your Code: A Beginner's Guide to Git
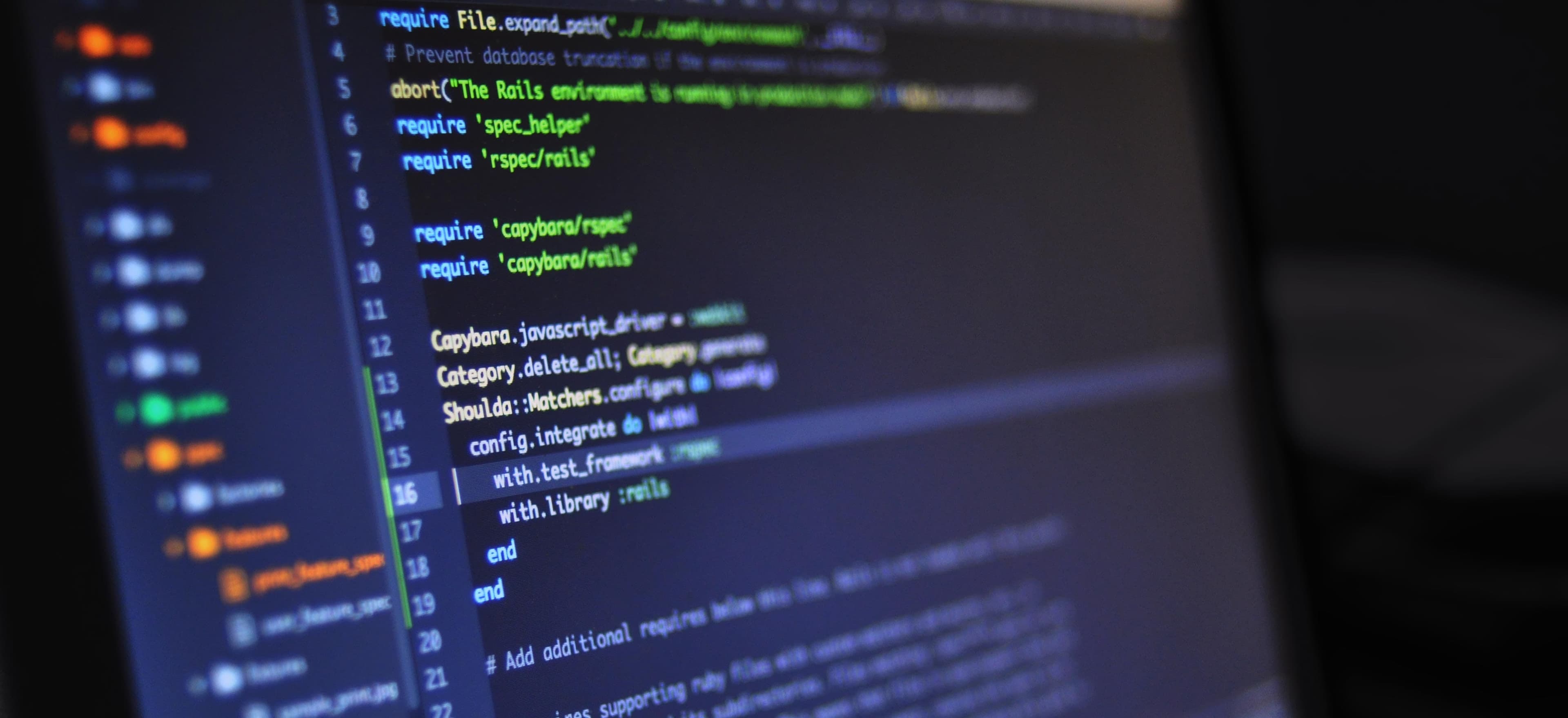
- Published on
Master Your Code: A Beginner's Guide to Git
If you’re a beginner developer, you’re probably eager to learn about version control systems that can help you manage your code effectively. And when it comes to version control, Git is the go-to choice for many developers. In this guide, we’ll walk you through the essential concepts of Git and how to use it efficiently in your Java projects.
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It was created by Linus Torvalds in 2005 for development of the Linux kernel, with the primary goal of supporting non-linear development.
Why Use Git?
- Collaboration: Git allows multiple developers to work on a project simultaneously without interfering with each other's work.
- Tracking Changes: Git makes it easy to track changes in your code, making it simpler to pinpoint when errors were introduced.
- Undo Mistakes: You can easily rollback to a previous version if something goes wrong.
- Branching and Merging: Git’s branching and merging capabilities allow for parallel development and experimental features development.
Installing Git
To get started with Git, you first need to install it. You can download Git from the official Git website, and install it by following the installation instructions for your specific operating system.
Git Basics
Initializing a Git Repository
To start using Git in your Java project, navigate to your project directory in the terminal and run the following command:
git init
This command initializes a new Git repository for your project. It will create a hidden directory named .git
where Git keeps all of its necessary files and directories.
Adding Files to the Staging Area
Before committing your changes, you need to add the files to the staging area. This can be done using the following command:
git add <file_name>
After adding the files, you can check the status of the staged files using:
git status
Committing Changes
Once your files are staged, you can commit them using the following command:
git commit -m "Your commit message here"
This creates a new commit containing the changes you’ve made to your code.
Viewing Commit History
You can view the commit history using:
git log
This shows the commit history, including the commit message, author, date, and a unique hash for each commit.
Branching and Merging
Creating a New Branch
To create a new branch, use the following command:
git branch <branch_name>
This creates a new branch but doesn't switch to it. To switch to the new branch, use:
git checkout <branch_name>
Merging Branches
After making changes in a separate branch, you might want to merge those changes back into the main branch. To do this, switch to the main branch using:
git checkout main
Then, run the merge command:
git merge <branch_name>
Working with Remotes
Cloning a Repository
To clone an existing remote repository, use:
git clone <remote_repository_url>
This creates a local copy of the remote repository in your current directory.
Pushing Changes
To push your committed changes to the remote repository, use:
git push origin <branch_name>
This command sends your commits to the remote repository.
Pulling Changes
If there are new changes in the remote repository, you can pull them to your local repository using:
git pull origin <branch_name>
Ignoring Files
Sometimes, there are files or directories in your project that you don't want Git to track. You can ignore them by creating a .gitignore
file in the root of your project and adding the file/directory names to be ignored.
Best Practices
Write Descriptive Commit Messages
When making a commit, it's important to write clear and descriptive commit messages that explain the changes you’ve made. This makes it easier for you and other developers to understand the purpose of each commit.
Keep Commits Small and Focused
Instead of making a large commit with multiple changes, it’s better to keep your commits small and focused on a single task. This makes it easier to track changes and understand their impact.
Use Branches for Feature Development
When working on new features or fixing bugs, it’s best to create a new branch for each task. This keeps the main branch clean and allows for better collaboration.
Regularly Pull and Push Changes
Frequent pulling and pushing of changes to and from the remote repository ensures that your local repository is up to date and that your changes are backed up on the remote server.
Review Diffs Before Committing
Before making a commit, review the changes you’ve made using the git diff
command to ensure that you’re committing the intended changes.
A Final Look
Git is a powerful tool that can greatly improve your workflow as a Java developer. By understanding the basics of Git and adhering to best practices, you can ensure smoother collaboration with other developers and better manage the version control of your Java projects. Start by practicing the fundamental commands and gradually explore advanced features to become a proficient Git user.
Checkout our other articles