Why Overloading Lambdas in Java Can Backfire
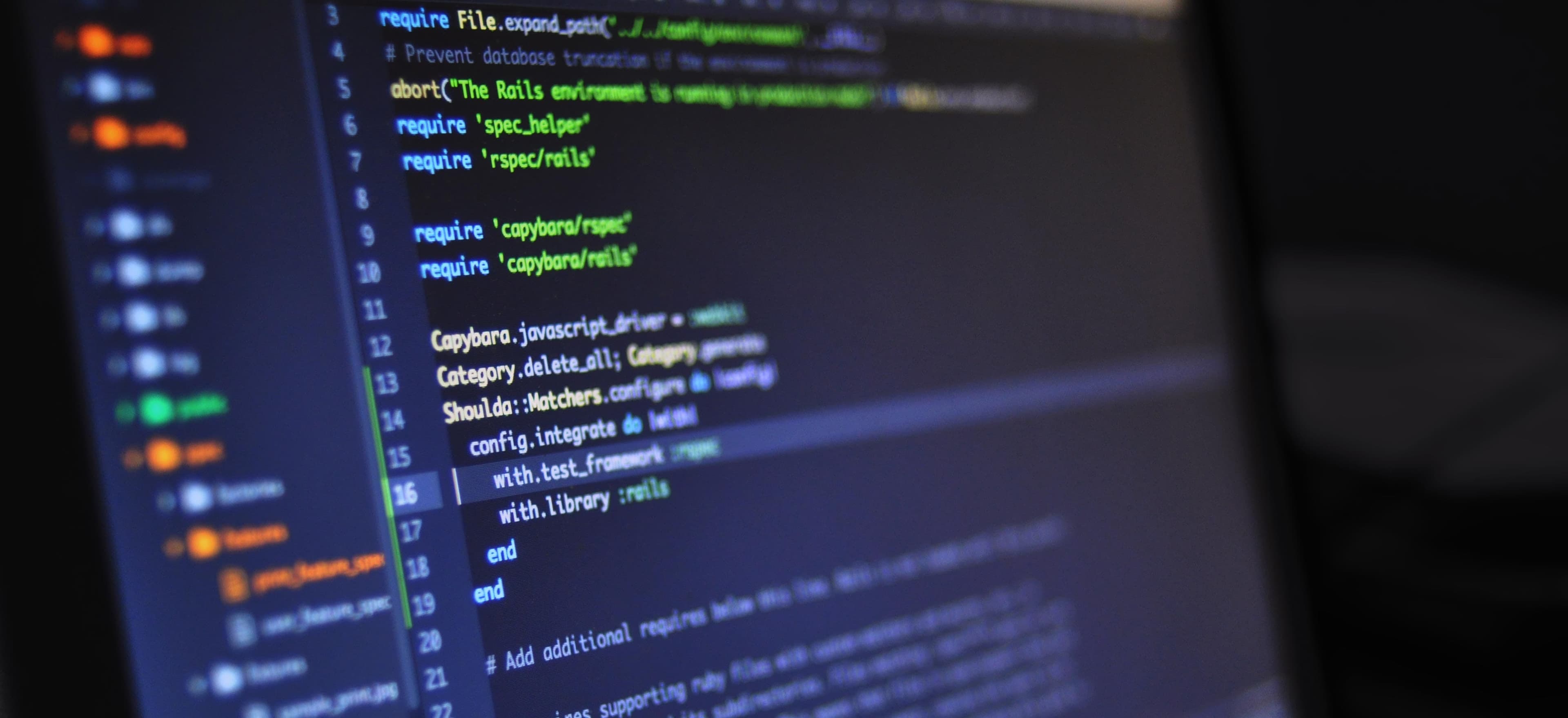
- Published on
Why Overloading Lambdas in Java Can Backfire
Starting Off
Java is a popular programming language used in various domains, including web development, Android apps, and enterprise software. One of the key features introduced in Java 8 was lambda expressions, which revolutionized the way developers write code by providing a more concise and readable syntax for implementing functional interfaces.
As Java developers embraced the power of lambdas, they also encountered challenges related to method overloading with lambda expressions. In this article, we explore why overloading lambdas in Java can backfire and provide best practices to avoid potential issues.
What is Lambda Overloading?
Lambda overloading refers to the practice of overloading methods with parameters that accept different functional interfaces. For example, consider a class with overloaded methods where each method accepts a different functional interface:
public class LambdaOverloadingExample {
public void process(Consumer<String> consumer) {
// Process using Consumer
}
public void process(Function<String, String> function) {
// Process using Function
}
}
When a lambda expression is passed as an argument to the process
method, the compiler may face ambiguity in determining which overloaded method to call, leading to the resolution problem.
LambdaOverloadingExample example = new LambdaOverloadingExample();
example.process(s -> s.toLowerCase()); // Error: Ambiguous method call
In this example, the compiler struggles to resolve the call because the lambda expression s -> s.toLowerCase()
can be compatible with both the Consumer
and the Function
interfaces.
Why Overloading Lambdas Can Backfire
Several issues can arise from overloading lambdas in Java, impacting both code performance and maintainability.
Performance Implications
When overloading methods with different functional interfaces, the method resolution process becomes more complex, potentially affecting the performance of the application. This complexity arises from the need for the compiler to determine the most specific method that can be invoked based on the lambda expression's type.
Maintainability and Readability Concerns
Overloading methods with lambda parameters can make the code harder to understand and maintain. It introduces ambiguity, making it challenging for developers to discern the intended function call when reading the code. This can lead to confusion and errors during code maintenance and future enhancements.
Real-world Scenarios
In real-world scenarios, overloading lambdas can pose challenges in complex systems or APIs where multiple methods accept lambda expressions. As codebases grow, the overuse of method overloading with lambdas can become a maintenance nightmare, hampering the understanding and modification of the code.
Best Practices to Avoid Issues
To avoid the issues associated with lambda overloading in Java, it is advisable to follow certain best practices that promote code clarity and maintainability.
Use Distinct Method Names
Instead of overloading methods with different functional interfaces, consider using distinct method names that clearly indicate the intended functionality. This practice makes the code more explicit and easier to comprehend.
Example:
public class LambdaMethodNamesExample {
public void processWithConsumer(Consumer<String> consumer) {
// Process using Consumer
}
public void processWithFunction(Function<String, String> function) {
// Process using Function
}
}
By using distinct method names, developers can bypass the ambiguity associated with overloading and provide clear indications of the functions performed by each method.
Leverage Custom Functional Interfaces
Create custom functional interfaces with clearly typed methods tailored to specific use cases. By doing so, the potential for ambiguity in method resolution is mitigated, and the code becomes more descriptive and maintainable.
Example:
@FunctionalInterface
interface TextProcessor {
String process(String input);
}
public class CustomFunctionalInterfaceExample {
public void processText(TextProcessor textProcessor) {
// Process using the custom functional interface
}
}
Custom functional interfaces provide explicit contracts for their usage, making the code more transparent and easier to work with, especially when dealing with lambda expressions.
The Bottom Line
The use of lambdas in Java has undoubtedly improved the readability and expressive power of the language. However, overloading methods for lambda expressions can lead to confusion, performance issues, and decreased maintainability. By adhering to best practices such as using distinct method names and custom functional interfaces, developers can avoid the potential pitfalls of lambda overloading and ensure clear, maintainable code.
It's essential for Java developers to continuously explore best practices and understand the nuances of language features to deliver high-quality, maintainable code in their projects.
Further Reading
- The Java™ Tutorials - Lambda Expressions
- Java™ Platform, Standard Edition API Specification - Functional Interfaces
(Optional) Comments Section
Have you encountered challenges with overloading lambdas in Java? Share your experiences and any tips or tricks you've found useful to handle lambda overloading effectively.
SEO Keywords
Java programming, Lambda expressions in Java, Method overloading, Functional programming in Java, Best practices in Java coding