Fixing Hidden, Not Gone: JavaFX StackPane Dilemma
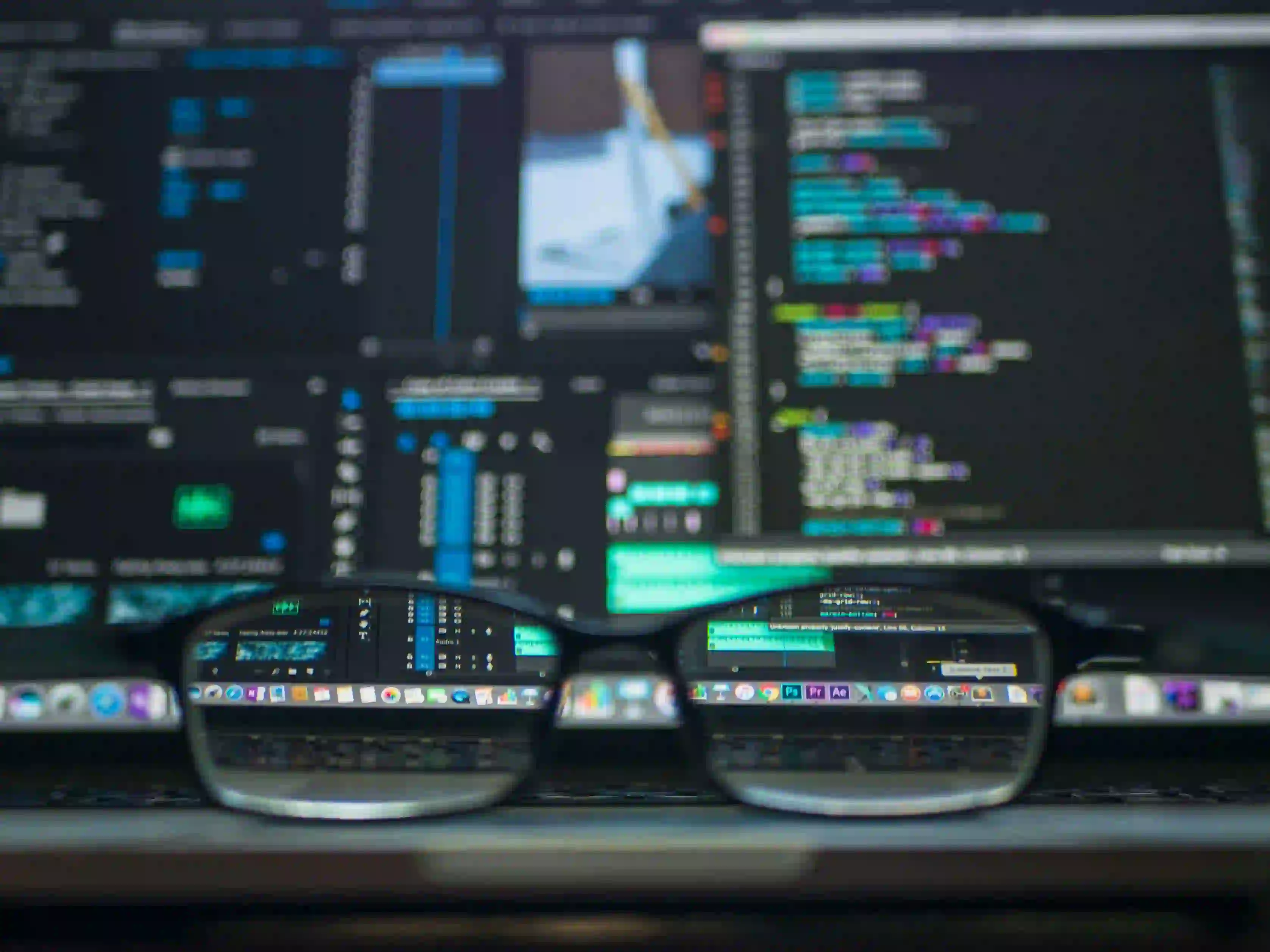
Understanding the JavaFX StackPane Dilemma
In the world of Java GUI programming, JavaFX has emerged as a powerful framework for building rich, interactive applications. When working with complex layouts, developers often find themselves facing the StackPane dilemma - where seemingly disappeared nodes actually remain hidden within the StackPane's layout.
The Problem
Let's imagine a scenario where you have a StackPane with multiple nodes, including a Button and a Label. Upon interacting with the Button, you anticipate the Label to disappear, but it doesn't. You might be baffled by the fact that despite setting the visibility of the Label to false, it retains its position within the StackPane's layout.
The issue arises from the way StackPane manages its children. Because it positions all children at the same location, regardless of their visibility, the hidden nodes still take up space within the layout.
The Solution: Managing Visibility
To address this issue, we need to consider an alternative approach to managing the visibility of nodes within a StackPane. One effective method is to remove the node from the StackPane when it should be hidden and re-add it when it should be visible.
Removing and Adding Nodes
Button toggleButton = new Button("Toggle Label");
Label label = new Label("Hello, World!");
toggleButton.setOnAction(event -> {
if (stackPane.getChildren().contains(label)) {
stackPane.getChildren().remove(label);
} else {
stackPane.getChildren().add(label);
}
});
In the above code, we associate an EventHandler
to the toggleButton
that checks if the stackPane
contains the label
. If it does, it removes the label
from the stackPane
; otherwise, it adds the label
to the stackPane
.
By dynamically removing and adding nodes, we effectively manage their visibility within the StackPane while overcoming the inherent layout constraints.
A Final Look
The JavaFX StackPane dilemma of hidden, not gone, nodes can be effectively resolved by understanding how the StackPane manages its children and employing dynamic removal and addition of nodes to control their visibility.
By implementing these strategies, you can ensure that hidden nodes no longer disrupt the layout of the StackPane, thereby enhancing the user experience of your JavaFX applications.
Remember, understanding the underlying behavior of JavaFX layouts and components is integral to developing robust and intuitive GUI applications.
For more in-depth insights into JavaFX and GUI development, you can refer to the official JavaFX documentation.