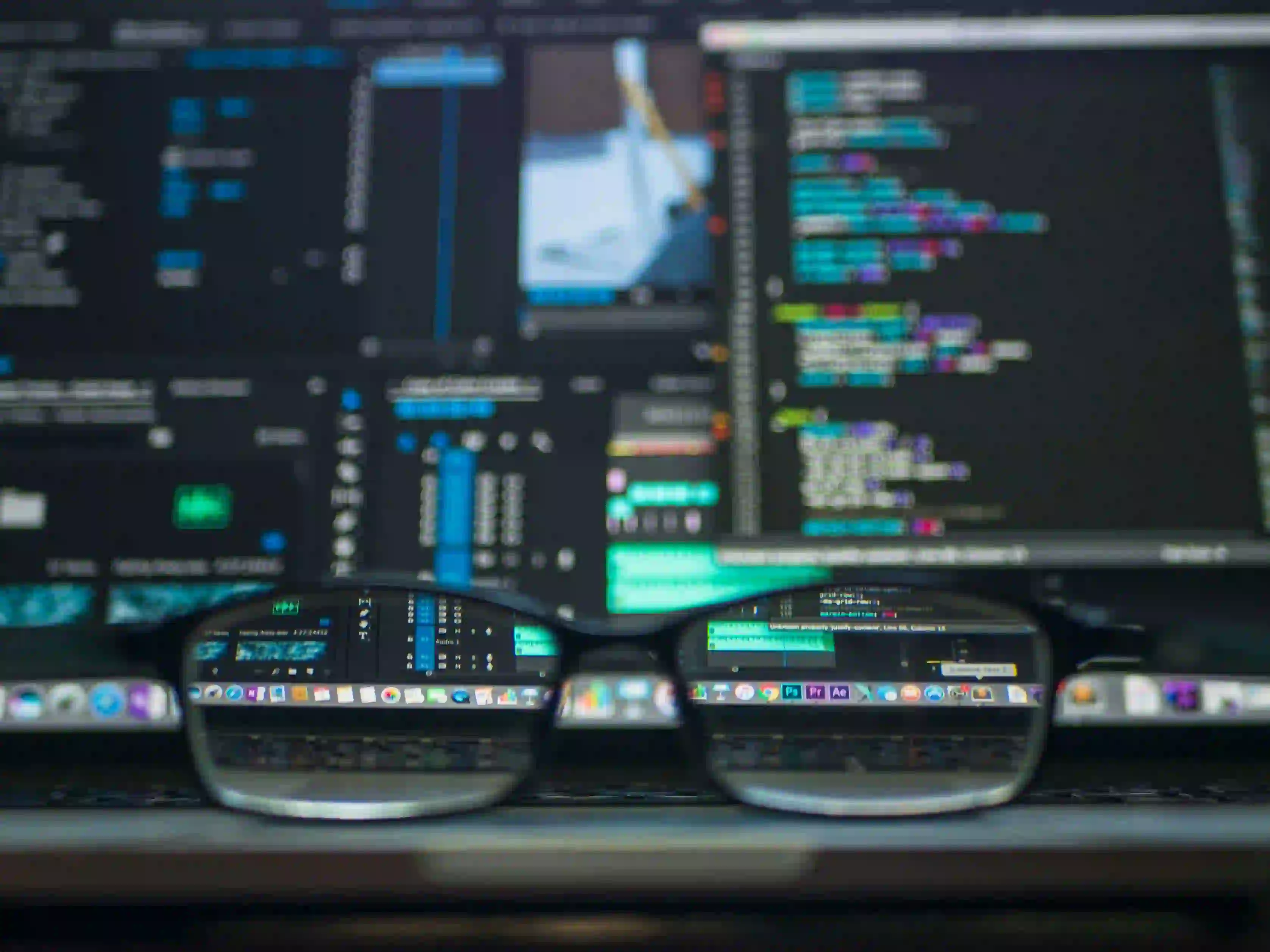
Choosing Java Build Tools: Ant, Maven, or Gradle?
When it comes to building and managing Java projects, the choice of build tool is crucial. The right build tool can streamline the development process, enhance collaboration, and ensure the efficiency and stability of the project. In the Java ecosystem, three prominent build tools stand out: Apache Ant, Apache Maven, and Gradle. Each of these tools offers unique features and benefits. In this blog post, we will delve into the details of each build tool, compare their strengths and weaknesses, and discuss the factors to consider when choosing the most suitable build tool for your Java project.
Apache Ant: The OG Build Tool
Apache Ant, the original Java build tool, has been a stalwart in the Java development community for many years. It is known for its flexibility and simplicity. Ant uses XML-based configuration files, allowing developers to define build tasks and dependencies in a structured manner.
Example of an Ant Build File:
<project name="MyProject" default="build" basedir=".">
<property name="src.dir" value="src"/>
<property name="build.dir" value="build"/>
<target name="init">
<mkdir dir="${build.dir}"/>
</target>
<target name="compile" depends="init">
<javac srcdir="${src.dir}" destdir="${build.dir}"/>
</target>
<target name="build" depends="compile">
<jar destfile="MyProject.jar" basedir="${build.dir}"/>
</target>
</project>
In this example, the Ant build file defines tasks for initializing the build directory, compiling source code, and creating a JAR file.
Advantages of Apache Ant:
- Flexibility: Ant allows fine-grained control over the build process, making it suitable for complex build requirements.
- Simplicity: The XML-based configuration is easy to read and understand, making it accessible to developers.
Disadvantages of Apache Ant:
- Boilerplate Code: Writing and maintaining XML build files can lead to verbosity and repetition.
- Limited Dependency Management: Ant lacks built-in dependency management capabilities, relying on manual inclusion of external libraries.
Apache Maven: Convention over Configuration
Apache Maven, introduced as an evolution from Ant, brought convention over configuration to the build process. Maven relies on predefined project structures and lifecycle phases, reducing the need for manual configuration.
Example of a Maven Project Structure:
MyProject/
āāā src/
ā āāā main/
ā āāā java/
ā āāā resources/
āāā pom.xml
Example of a Maven POM File:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>MyProject</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
In this example, the Maven POM file defines project metadata and dependencies.
Advantages of Apache Maven:
- Convention over Configuration: By adhering to conventions, Maven reduces the need for extensive build configuration.
- Dependency Management: Maven centralizes dependency management, simplifying the inclusion of external libraries.
Disadvantages of Apache Maven:
- Rigid Structure: Maven's reliance on convention may not be suitable for all project structures and requirements.
- Plugins Overhead: Customizing the build process often requires the use of Maven plugins, leading to additional complexity.
Gradle: The New Kid on the Block
Gradle, a relatively newer entrant in the Java build tool landscape, aims to combine the best features of Ant and Maven while addressing their limitations. Gradle uses a Groovy-based DSL, allowing developers to express build configurations and tasks using a concise and expressive syntax.
Example of a Gradle Build Script:
plugins {
id 'java'
}
repositories {
jcenter()
}
dependencies {
implementation 'junit:junit:4.12'
}
In this example, the Gradle build script defines project plugins, repositories, and dependencies.
Advantages of Gradle:
- Concise DSL: The use of a Groovy-based DSL makes Gradle build scripts more expressive and succinct.
- Flexibility: Gradle offers the flexibility to define custom build logic, making it suitable for diverse project requirements.
Disadvantages of Gradle:
- Learning Curve: Transitioning from Ant or Maven to Gradle may require time to familiarize with the Groovy DSL and its ecosystem.
- Plugin Ecosystem: While Gradle offers a rich plugin ecosystem, managing plugin versions and compatibility can be challenging.
Choosing the Right Build Tool for Your Java Project
When it comes to selecting a build tool for your Java project, there are several factors to consider:
-
Project Complexity: For simple and straightforward projects, Apache Ant's flexibility may be unnecessary, making Apache Maven's convention over configuration a suitable choice. However, for complex or specialized projects, Gradle's flexibility can be invaluable.
-
Team Familiarity: Consider the familiarity and expertise of your development team. If they have extensive experience with a particular build tool, it may influence your decision.
-
Ecosystem Integration: Evaluate how well the build tool integrates with other development tools, such as IDEs, CI/CD pipelines, and artifact repositories.
-
Community and Support: Assess the community activity, documentation, and support resources available for each build tool.
-
Future Scalability: Anticipate the future scalability and extensibility of your project. Choose a build tool that can accommodate the evolving needs of your project.
In conclusion, the choice between Apache Ant, Apache Maven, and Gradle depends on the specific requirements and dynamics of your Java project. While Apache Ant brings flexibility, Apache Maven offers convention, and Gradle provides a balanced blend of both. It is essential to evaluate your project's characteristics and your team's preferences to make an informed decision.
In the ever-evolving landscape of Java development, the right build tool can streamline the development process, foster collaboration, and contribute to the overall success of your project.
Remember, the choice of build tool is not set in stone, and projects may evolve to leverage different build tools at different stages of their lifecycle. Stay open to re-evaluating your build tool to ensure it aligns with your project's evolving needs.
Let's stay connected! For more insights on Java development and best practices, you can check out Oracle's official Java documentation. If you're interested in exploring the latest trends and community discussions, you may find valuable resources on Java subreddit.