5 Must-Know Tips Before Choosing Your API Manager
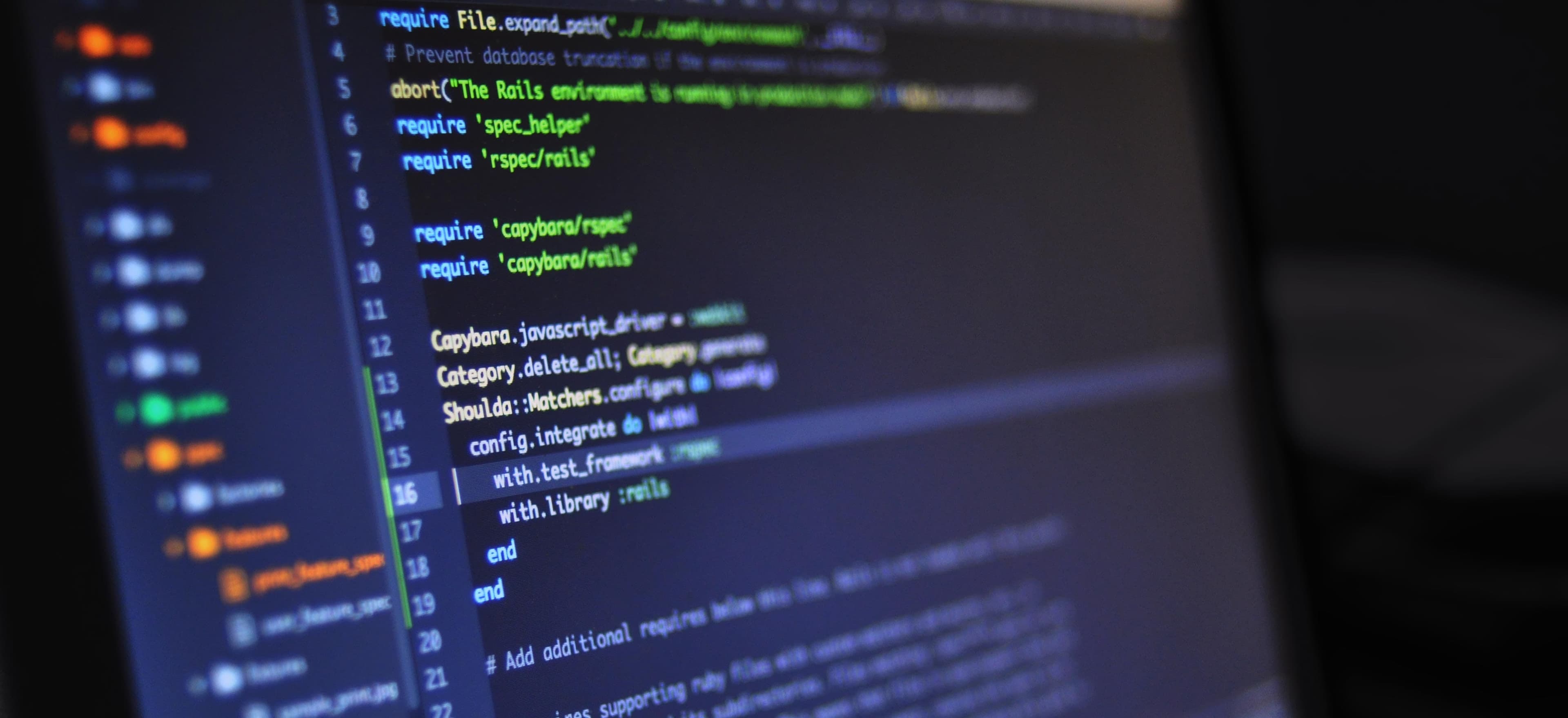
- Published on
5 Must-Know Tips Before Choosing Your API Manager
When it comes to managing APIs, choosing the right API manager is crucial for the success of your project. An API manager helps in maintaining, securing, and distributing APIs to developers and partners. However, with the myriad of options available, it can be overwhelming to select the most suitable API manager for your specific needs. Here are five essential tips to consider before making your decision.
1. Security Features Are Non-Negotiable
Security is paramount when it comes to managing APIs. Your API manager should offer robust security features to protect your APIs from potential threats. Look for features such as authentication, authorization, encryption, and threat protection. Additionally, ensure that the API manager is compliant with industry standards such as OAuth and OpenID Connect. By prioritizing security, you can safeguard your APIs and prevent unauthorized access or data breaches.
// Example of security configuration in an API manager using OAuth 2.0
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll()
.and()
.oauth2Login();
}
}
In the above code snippet, the SecurityConfig
class showcases how security is configured using OAuth 2.0 to ensure that API endpoints are accessible only to authenticated users.
2. Scalability Is Key for Future Growth
As your business expands and the usage of your APIs grows, scalability becomes a critical factor. Your API manager should be able to handle increased traffic and growing demands without compromising performance. It should offer scalability features such as load balancing, clustering, and caching to ensure seamless operation during peak loads. Assess the scalability capabilities of the API manager to accommodate the future growth of your API ecosystem.
// Example of implementing caching for improved scalability
@Cacheable("products")
public Product getProductById(String productId) {
// Method logic to retrieve product from database
}
In the above snippet, the @Cacheable
annotation is used to cache the results of the getProductById
method, reducing the need for repeated database calls and improving scalability.
3. Developer-Friendly Tools Promote Adoption
A developer-friendly API manager simplifies the process of API integration and encourages developers to leverage your APIs. Look for features like comprehensive documentation, interactive API consoles, SDKs, and developer portals. These tools empower developers to understand, test, and integrate with your APIs effectively. A user-friendly developer experience can significantly impact the adoption and success of your APIs.
// Example of API documentation using Swagger
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.api.controllers"))
.paths(PathSelectors.any())
.build();
}
}
The aforementioned code demonstrates the use of Swagger to generate API documentation, making it easier for developers to understand and utilize the available endpoints.
4. Analytics and Reporting Provide Valuable Insights
Insightful analytics and reporting capabilities are indispensable for understanding the usage patterns, performance, and impact of your APIs. The API manager should offer comprehensive analytics dashboards, real-time monitoring, and customizable reports. These features enable you to gain actionable insights, identify bottlenecks, track API usage, and make informed decisions to optimize your APIs. Prioritize an API manager that empowers you with meaningful analytics.
// Example of capturing API usage metrics for analytics
@Aspect
@Component
public class ApiUsageAspect {
@AfterReturning(pointcut = "execution(* com.example.api.*Controller.*(..))", returning = "result")
public void captureApiUsage(JoinPoint joinPoint, Object result) {
// Logic to capture and record API usage metrics
}
}
In the code snippet above, an aspect is used to intercept API method executions and capture usage metrics, which can then be utilized for generating valuable analytics.
5. Integration Capabilities Streamline Workflows
Efficient integration capabilities are essential for seamless interoperability with existing systems and tools. Your API manager should support various integration options such as webhooks, messaging queues, and third-party integrations. Furthermore, it should facilitate the integration of API management with other relevant platforms, such as CRM systems or identity providers, to streamline workflows and enhance the overall efficiency of your API ecosystem.
// Example of integrating API manager with a messaging queue
@Component
public class OrderProcessor {
@Autowired
private MessagingQueueService messagingQueueService;
public void processOrder(Order order) {
// Process order logic
messagingQueueService.sendMessage("order-processed", order.getId());
}
}
In the provided code, the OrderProcessor
class demonstrates the integration of the API manager with a messaging queue for asynchronous processing, illustrating how integration capabilities can streamline workflows.
In conclusion, when selecting an API manager, prioritize security, scalability, developer-friendliness, analytics, and integration capabilities to ensure the seamless management and utilization of your APIs. By considering these five essential tips, you can make an informed decision and choose an API manager that aligns with your specific requirements, ultimately contributing to the success of your API initiatives.
Remember, the right API manager sets the foundation for a robust and thriving API ecosystem.
For further insights into API management, you can explore leading API management platforms such as Apigee and MuleSoft Anypoint Platform.
Checkout our other articles