Mastering Spring: Solving FactoryBean Confusion
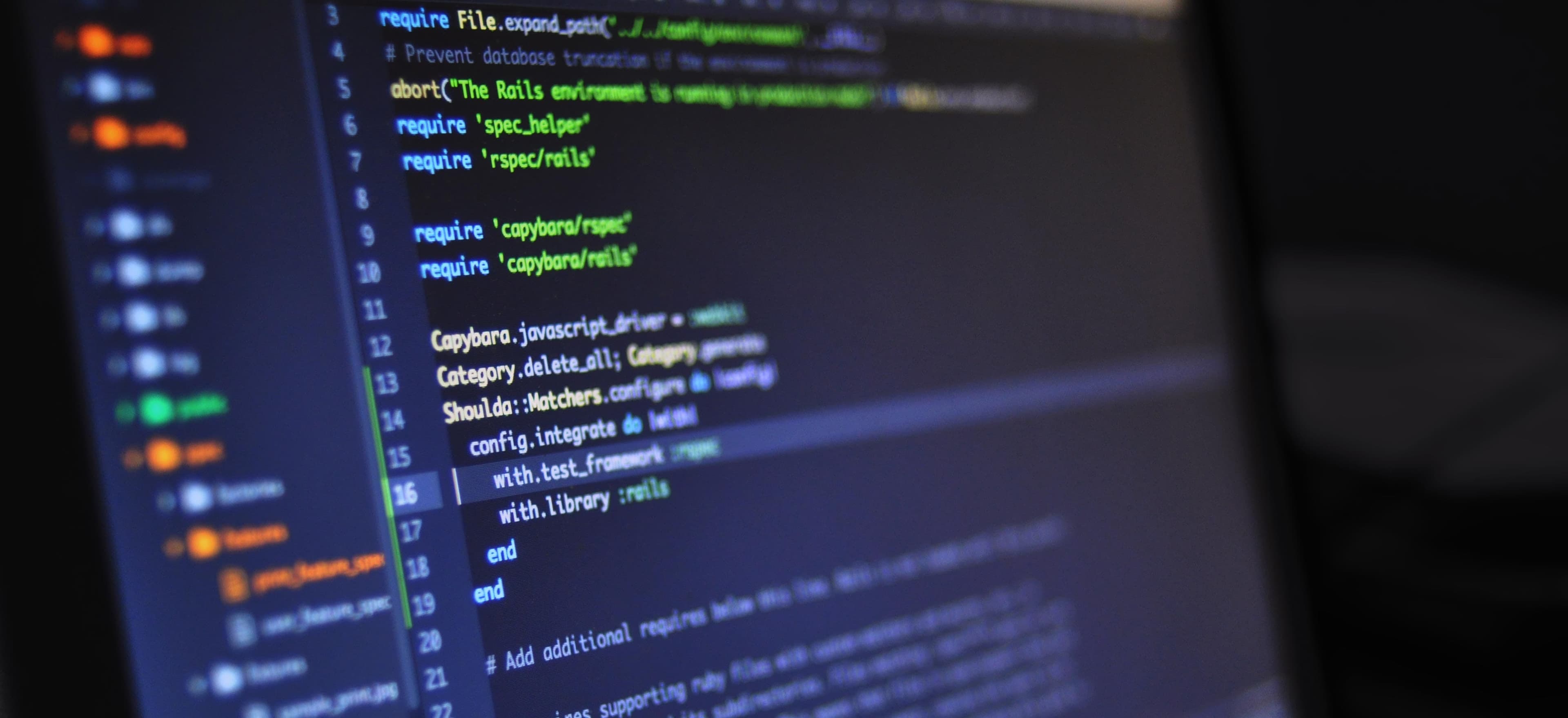
- Published on
Mastering Spring: Solving FactoryBean Confusion
Spring is a powerful and widely-used framework for building Java applications. One of the key features of Spring is its support for Inversion of Control (IoC) and Dependency Injection, which allows for the creation and management of beans in the application context. In this article, we'll delve into the confusion surrounding the usage of FactoryBean in Spring and how to master it effectively.
Understanding FactoryBean
In Spring, a FactoryBean is a special type of bean that is responsible for creating other beans within the application context. It provides a way to encapsulate the creation logic of a bean, allowing for more dynamic and flexible bean instantiation. The FactoryBean interface has a method called getObject()
, which returns an instance of the bean it creates.
Let's take a look at an example of a custom FactoryBean implementation:
import org.springframework.beans.factory.FactoryBean;
public class CustomFactoryBean implements FactoryBean<CustomBean> {
@Override
public CustomBean getObject() throws Exception {
// Custom logic to create and return the CustomBean instance
return new CustomBean();
}
@Override
public Class<?> getObjectType() {
return CustomBean.class;
}
}
In this example, CustomFactoryBean
is a custom implementation of FactoryBean
that is responsible for creating instances of CustomBean
. The getObjectType()
method specifies the type of object that will be created by the factory.
Why Use FactoryBean?
You might wonder why we should use FactoryBean when we can simply define beans in the XML configuration or using annotations. The key advantage of using FactoryBean is its ability to apply custom logic during bean creation. This can be useful in scenarios where the instantiation of a bean is complex and requires conditional logic or external configuration.
Usage of FactoryBean
Using a FactoryBean in Spring is straightforward. We define the FactoryBean as a bean in the application context, and when we need an instance of the target bean, we retrieve it from the FactoryBean using the getObject()
method.
Here's how we define a FactoryBean in the Spring configuration:
<bean id="customFactoryBean" class="com.example.CustomFactoryBean"/>
And here's how we retrieve the target bean from the FactoryBean in Java code:
CustomBean customBean = applicationContext.getBean("customFactoryBean", CustomBean.class).getObject();
Common Confusions and How to Solve Them
-
Mismatched Type Error
One common confusion when using FactoryBean is encountering a mismatched type error during bean retrieval. This may occur when trying to retrieve the bean using the wrong type.
To solve this, ensure that when retrieving the bean from the FactoryBean, the correct type is specified to avoid type mismatches.
-
Bean Definition Ambiguity
Another issue that developers face is bean definition ambiguity. This can occur when there are multiple FactoryBeans or bean definitions with similar names or types.
To resolve this, use unique and descriptive bean IDs and names, and organize the bean definitions to minimize ambiguity.
-
Incorrect Configuration
Misconfiguring the FactoryBean in the Spring configuration can lead to runtime errors or unexpected behavior. Double-check the bean definition and configuration to ensure consistency and correctness.
Best Practices for Using FactoryBean
Now that we have demystified the confusion surrounding FactoryBean, let's discuss some best practices for using it effectively in Spring applications:
-
Encapsulate Complex Bean Creation Logic
Use a FactoryBean to encapsulate the complex logic involved in creating a bean, especially when the process is conditional or requires external configuration. This promotes better code organization and maintainability.
-
Consistent Naming and Documentation
Ensure that the FactoryBean, its methods, and the beans it creates are named descriptively. Additionally, provide clear documentation for the FactoryBean's purpose and the process of creating the beans.
-
Testing FactoryBean Implementations
Write unit tests for the FactoryBean implementations to validate the creation logic and ensure that the beans are created as expected. Mockito and JUnit are great tools for writing comprehensive tests.
A Final Look
In this article, we've dived into the world of FactoryBean in Spring and gained a better understanding of its purpose and usage. We've covered the reasons for using FactoryBean, typical confusions that arise, and best practices for leveraging FactoryBean effectively in Spring applications.
By mastering FactoryBean, you can bring greater flexibility and customization to your application's bean creation process, paving the way for more maintainable and scalable code.
So, embrace the power of FactoryBean and level up your Spring skills! Happy coding!
By mastering FactoryBean, you can bring greater flexibility and customization to your application's bean creation process, paving the way for more maintainable and scalable code.
Checkout our other articles