Ensuring Data Safety: Mastering Consistency Checks in Neo4j
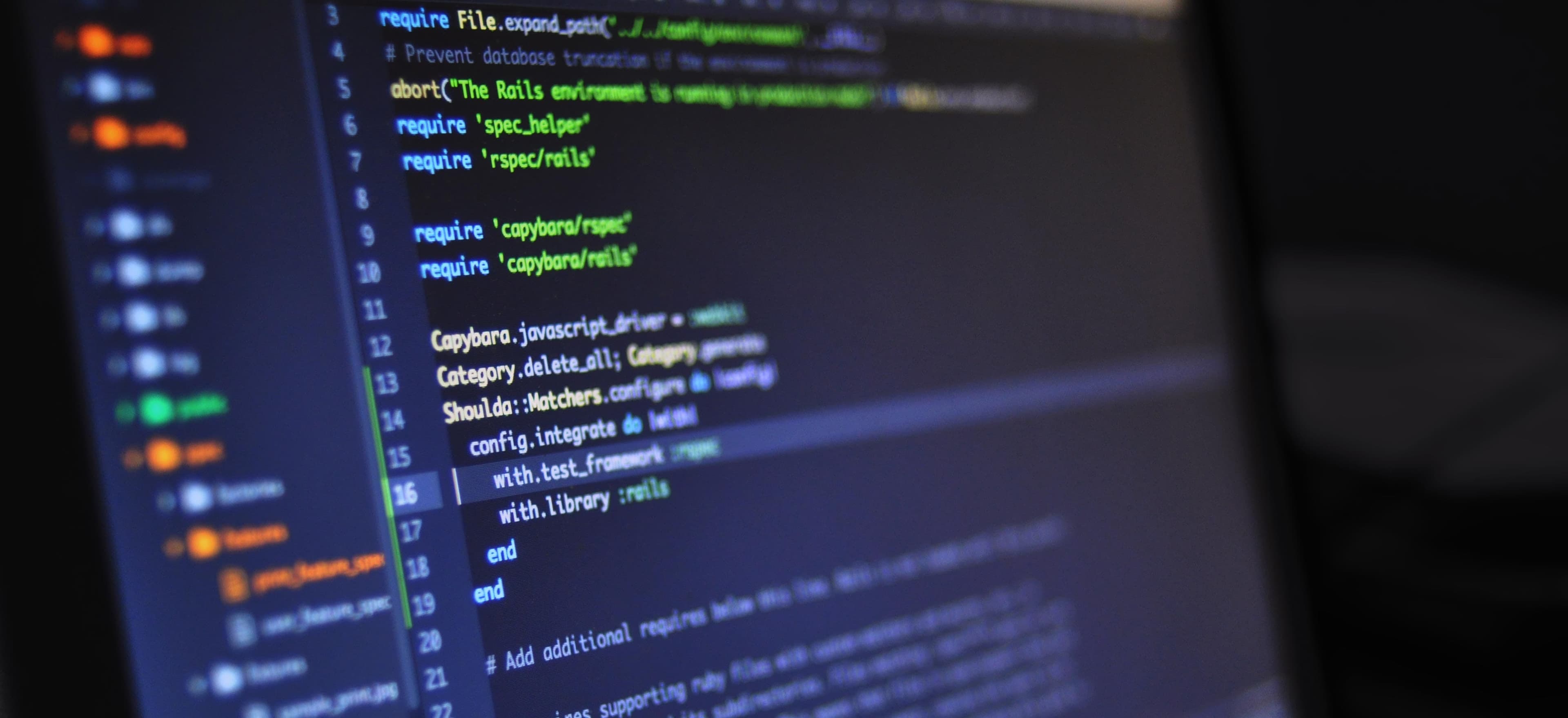
- Published on
Mastering Consistency Checks in Neo4j
In the world of database management, ensuring data consistency is a critical task. Neo4j, a powerful graph database, provides various features and tools to maintain data integrity and consistency. Consistency checks play a vital role in identifying and rectifying data inconsistencies that may arise due to various factors such as hardware faults, software bugs, or human errors.
In this article, we will delve into the significance of consistency checks in Neo4j, explore how they can be performed, and discuss best practices for ensuring data safety.
Why Consistency Checks Matter
Data consistency ensures that the data within a database remains accurate and reliable. In a graph database like Neo4j, where relationships between data are of paramount importance, maintaining consistency is crucial. Inaccurate data can lead to incorrect analysis, flawed decision making, and ultimately, loss of trust in the application.
By performing consistency checks, you can identify and rectify inconsistencies such as missing nodes, incorrect relationships, or orphaned nodes. This proactive approach helps in maintaining data accuracy and improves the overall reliability of the database.
Performing Consistency Checks in Neo4j
Neo4j provides several methods for performing consistency checks. Let's explore some of the key approaches:
1. Cypher Queries for Consistency Checks
Cypher, Neo4j's query language, empowers users to perform various consistency checks. For instance, you can run queries to find nodes with missing properties, relationships with incorrect data, or nodes with unexpected relationships.
// Find nodes with missing properties
MATCH (n)
WHERE NOT exists(n.property)
RETURN n
// Find relationships with incorrect data
MATCH ()-[r]->()
WHERE r.invalid = true
DELETE r
// Find nodes with unexpected relationships
MATCH (n)
WHERE size((n)--()) > 10
RETURN n
In the above example, we're using Cypher to identify nodes with missing properties, relationships with incorrect data, and nodes with an unusually high number of relationships.
2. Constraint Validation
Neo4j allows you to define constraints on the data model, ensuring that the data remains consistent. By creating unique constraints and node property existence constraints, you can prevent data inconsistencies at the time of data insertion or modification.
// Creating a unique constraint
CREATE CONSTRAINT ON (n:NodeLabel) ASSERT n.property IS UNIQUE
// Creating a node property existence constraint
CREATE CONSTRAINT ON (n:NodeLabel) REQUIRE n.property
By defining constraints, Neo4j ensures that the data adheres to the specified rules, thus maintaining consistency.
3. Using APOC Library
The APOC (Awesome Procedures on Cypher) library provides a wide array of procedures and functions to perform advanced database operations, including consistency checks.
// Using APOC to find orphaned nodes
CALL apoc.periodic.iterate(
'MATCH (n)
WHERE NOT (n)--()
RETURN n',
'DETACH DELETE n',
{batchSize:10000,parallel:false}
)
In the above example, we're utilizing APOC's apoc.periodic.iterate
to find and delete orphaned nodes, thus improving data consistency.
Best Practices for Data Consistency
While performing consistency checks is crucial, adopting best practices can further enhance data safety in Neo4j:
Regular Scheduled Checks
Schedule consistency checks at regular intervals to proactively identify and rectify data inconsistencies. Automated scripts or tools can be employed to streamline this process.
Utilize Indexes
Create indexes on properties that are frequently used for querying. Indexes improve query performance and ensure that the data can be accessed efficiently, reducing the likelihood of inconsistencies.
Monitor Performance Impact
Consistency checks, especially complex ones, can impact database performance. Monitor the performance impact of these checks and optimize them as required.
Use Transactions
When performing data modifications, encapsulate the operations within transactions. Transactions ensure that a group of operations either succeed as a whole or fail together, preventing partial updates and maintaining consistency.
Backup Data Regularly
In the event of data inconsistencies or failures, having regular backups ensures that you can restore the database to a consistent state.
Closing Remarks
Maintaining data consistency is pivotal in ensuring the reliability and accuracy of a Neo4j graph database. Consistency checks, when performed diligently, can help in identifying and rectifying data inconsistencies, thus upholding data integrity.
By utilizing Cypher queries, constraints, APOC library, and adhering to best practices, you can master consistency checks in Neo4j, paving the way for a robust and dependable database environment.
In conclusion, mastering consistency checks in Neo4j is an integral part of database management, contributing to data safety and trustworthiness. By integrating these practices into your database management strategies, you can fortify the foundations of your Neo4j database, securing the integrity and reliability of your data.
Remember, the journey to mastering consistency checks is an ongoing endeavor, and staying abreast of the latest advancements in Neo4j and data management practices will further augment your capabilities in ensuring data safety and reliability.
So, embrace the power of Neo4j, harness the capabilities of consistency checks, and embark on a journey towards a robust, consistent, and dependable graph database environment.