Maximizing Profit: Solving Data Delays with BigQuery
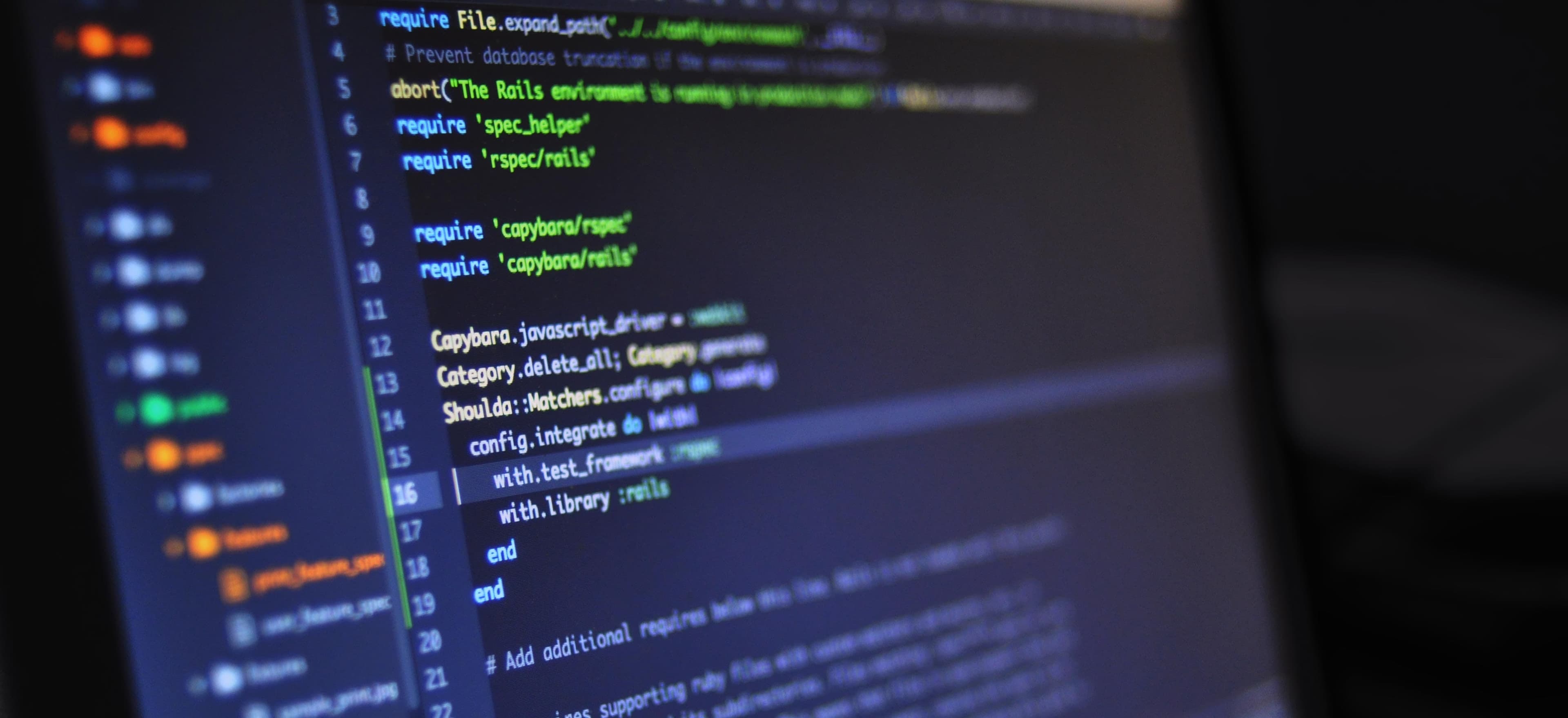
- Published on
Maximizing Profit: Solving Data Delays with BigQuery
In today's fast-paced business world, having access to real-time data is crucial for decision-making and staying ahead of the competition. However, many organizations struggle with data delays, which can impact their ability to make timely and informed decisions. In this blog post, we'll explore how Java developers can leverage Google BigQuery to solve data delays and maximize profit.
Understanding the Problem
Data delays occur when there is a lag between the time data is generated and when it becomes available for analysis and reporting. This delay can be caused by various factors, such as batch processing, outdated data pipelines, or inefficient data storage and retrieval mechanisms. For businesses, data delays can lead to missed opportunities, inaccurate insights, and ultimately, reduced profitability.
Java, being a popular programming language for enterprise applications, plays a critical role in addressing data delays. By utilizing the right tools and technologies, Java developers can build robust solutions to ingest, process, and analyze data in real-time.
Introducing Google BigQuery
Google BigQuery is a fully managed, serverless data warehouse that enables seamless analysis of petabytes of data using ANSI SQL. It offers high performance, scalability, and ease of use, making it an ideal choice for organizations looking to tackle data delays.
One of the key advantages of BigQuery is its ability to handle real-time data streams, allowing businesses to gain insights and take action as soon as data is generated. This is particularly valuable in scenarios such as e-commerce, IoT, and financial services, where timely decisions directly impact the bottom line.
Solving Data Delays with Java and BigQuery
Step 1: Data Ingestion
The first step in addressing data delays is to ensure that data is ingested into BigQuery in a timely manner. Java developers can achieve this by leveraging Google Cloud Client Libraries for Java, which provide a straightforward way to interact with BigQuery's REST API.
import com.google.cloud.bigquery.BigQuery;
import com.google.cloud.bigquery.BigQueryOptions;
import com.google.cloud.bigquery.DatasetId;
import com.google.cloud.bigquery.TableId;
import com.google.cloud.bigquery.Schema;
import com.google.cloud.bigquery.StandardTableDefinition;
import com.google.cloud.bigquery.TableInfo;
import com.google.cloud.bigquery.Job;
import com.google.cloud.bigquery.JobInfo;
import com.google.cloud.bigquery.JobId;
import com.google.cloud.bigquery.QueryJobConfiguration;
BigQuery bigquery = BigQueryOptions.getDefaultInstance().getService();
String query = "SELECT * FROM your_realtime_data_source WHERE timestamp > TIMESTAMP_SUB(CURRENT_TIMESTAMP(), INTERVAL 1 MINUTE)";
QueryJobConfiguration queryConfig = QueryJobConfiguration
.newBuilder(query)
.setUseLegacySql(false)
.build();
JobId jobId = JobId.of(UUID.randomUUID().toString());
Job queryJob = bigquery.create(JobInfo.newBuilder(queryConfig).setJobId(jobId).build());
queryJob = queryJob.waitFor();
if (queryJob == null) {
throw new RuntimeException("Job no longer exists");
} else if (queryJob.getStatus().getError() != null) {
throw new RuntimeException(queryJob.getStatus().getError().toString());
}
// Data ingestion successful
In the code snippet above, we are using the BigQuery Java client library to run a SQL query against a real-time data source and ingest the results into BigQuery. By utilizing the Timestamp
filter, we ensure that only recent data is ingested, thus enabling real-time analytics.
Step 2: Real-time Analysis
Once data is ingested into BigQuery, Java developers can perform real-time analysis using BigQuery's powerful SQL capabilities. This allows for interactive exploration of data, ad-hoc querying, and generating insights on the fly.
String realTimeAnalysisQuery = "SELECT COUNT(*) as total_records FROM your_realtime_data_table";
QueryJobConfiguration realTimeAnalysisConfig = QueryJobConfiguration
.newBuilder(realTimeAnalysisQuery)
.setUseLegacySql(false)
.build();
Job realTimeAnalysisJob = bigquery.create(JobInfo.of(realTimeAnalysisConfig));
realTimeAnalysisJob = realTimeAnalysisJob.waitFor();
if (realTimeAnalysisJob == null) {
throw new RuntimeException("Job no longer exists");
} else if (realTimeAnalysisJob.getStatus().getError() != null) {
throw new RuntimeException(realTimeAnalysisJob.getStatus().getError().toString());
}
// Process real-time analysis results
In the code snippet above, we are executing a real-time analysis query to calculate the total number of records in the ingested data. This demonstrates how Java developers can harness the power of BigQuery to gain immediate insights into real-time data, enabling quick decision-making.
Step 3: Automated Reporting and Visualization
To further maximize profit, organizations can automate reporting and visualization of real-time insights using Java-based web applications or dashboards. By integrating with tools like Google Data Studio or custom-built visualization components, businesses can ensure that relevant stakeholders have immediate access to critical information.
// Java web application or dashboard integration with BigQuery for real-time reporting and visualization
// Example: Utilizing Java web frameworks like Spring or Play to fetch and display real-time insights from BigQuery
By integrating Java web applications with BigQuery, businesses can create custom real-time dashboards and reports to monitor key performance indicators, identify trends, and make informed decisions on the go.
Closing the Chapter
In conclusion, Java developers can play a vital role in solving data delays and maximizing profit by leveraging Google BigQuery for real-time data analysis. By seamlessly ingesting, analyzing, and visualizing data, businesses can gain a competitive edge and capitalize on opportunities as they arise.
With the power of Java and BigQuery, organizations can transition from being reactive to proactive, making data-driven decisions in real time and ultimately driving profitability.
To learn more about Java development and data analysis with BigQuery, check out the official documentation and tutorials provided by Google Cloud Platform.
Start maximizing profit today with Java and BigQuery!
Checkout our other articles