Avoiding Common Pitfalls in SWT's asyncExec Usage
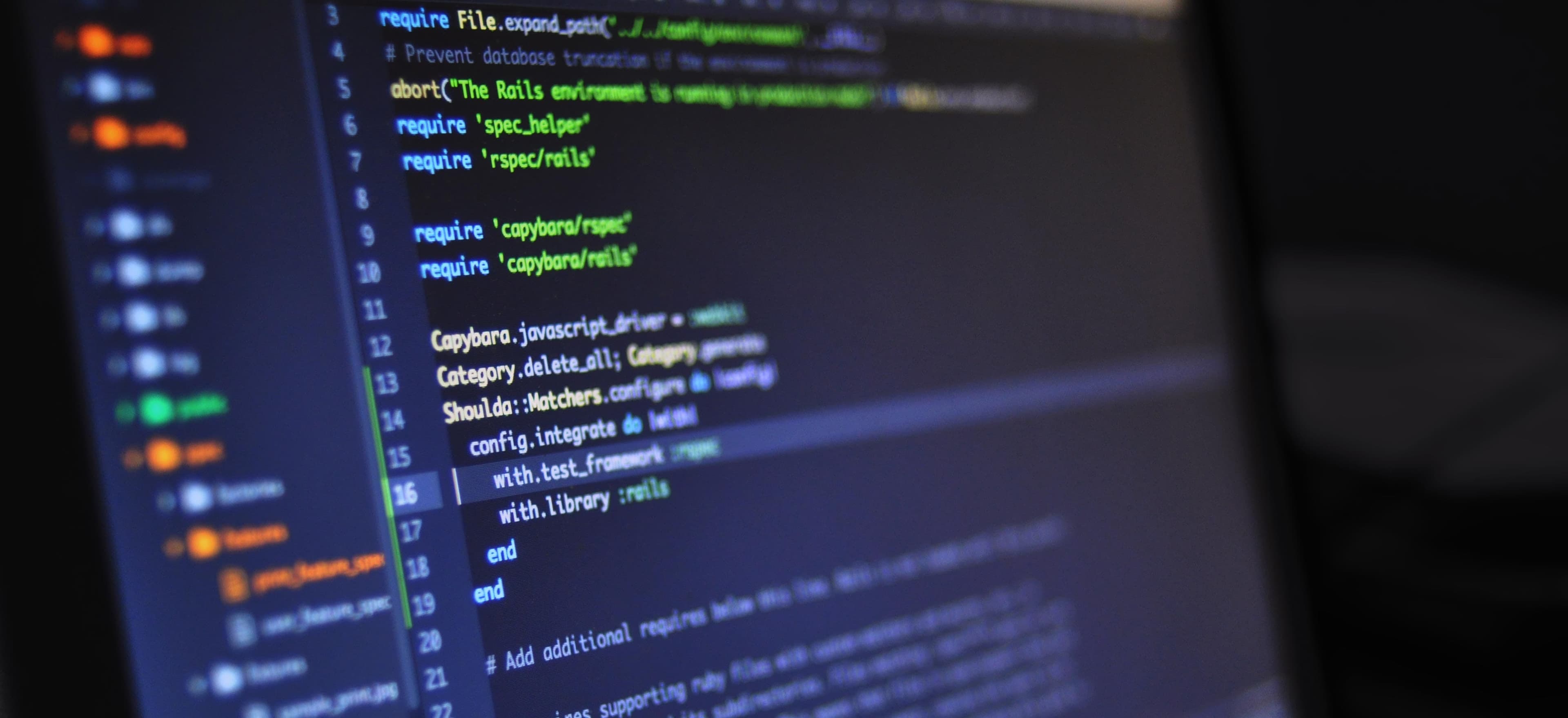
- Published on
Avoiding Common Pitfalls in SWT's asyncExec Usage
When developing applications using the Standard Widget Toolkit (SWT) in Java, it’s crucial to understand and utilize the asyncExec
method correctly. This method allows for safe execution of code in the UI thread, preventing potential race conditions and deadlocks. However, improper usage can lead to bugs that are difficult to debug and fix. In this article, we'll explore common pitfalls in using asyncExec
and how to avoid them.
Understanding asyncExec
SWT is designed to be single-threaded for UI updates. As a result, any UI-related code must be executed in the UI thread to prevent concurrency issues. This is where asyncExec
comes in. It allows code to be executed asynchronously in the UI thread, ensuring that UI updates are performed safely.
The basic syntax of the asyncExec
method is as follows:
display.asyncExec(new Runnable() {
public void run() {
// UI-related code goes here
}
});
The display
object represents the UI thread's display, and the Runnable
defines the code to be executed asynchronously.
Pitfalls to Avoid
1. Accessing Non-final Variables
One common mistake when using asyncExec
is accessing non-final variables from the enclosing scope within the Runnable
. This can lead to unexpected behavior due to the nature of anonymous inner classes in Java.
Incorrect Usage:
String message = "Hello";
display.asyncExec(new Runnable() {
public void run() {
System.out.println(message); // Accessing non-final variable
}
});
In the above example, accessing the non-final variable message
within the Runnable
can result in unpredictable behavior.
Correct Usage:
final String message = "Hello"; // Declare the variable as final
display.asyncExec(new Runnable() {
public void run() {
System.out.println(message); // Safe access to final variable
}
});
By declaring the variable as final
, it becomes immutable within the Runnable
, ensuring safe access.
2. Accessing UI Elements Directly from asyncExec
Another common mistake is attempting to access UI elements directly from the asyncExec
block. Since UI updates should only be performed within the UI thread, directly accessing UI elements from other threads can lead to potential race conditions and UI inconsistencies.
Incorrect Usage:
Button button = new Button(shell, SWT.PUSH);
display.asyncExec(new Runnable() {
public void run() {
button.setText("Clicked"); // Direct access to UI element
}
});
In the above example, directly accessing the UI element button
from asyncExec
can lead to UI-related issues.
Correct Usage:
display.asyncExec(new Runnable() {
public void run() {
Button button = new Button(shell, SWT.PUSH); // Create UI element within asyncExec
button.setText("Clicked"); // Safe access within UI thread
}
});
It's essential to create and manipulate UI elements within the asyncExec
block to ensure that all UI updates are performed in the UI thread.
3. Handling Disposed UI Elements
When working with asynchronous UI updates, it's important to handle disposed UI elements properly. Disposed UI elements no longer exist in the UI thread and attempting to access them can lead to SWTException
s and potential application crashes.
Incorrect Usage:
display.asyncExec(new Runnable() {
public void run() {
if (!button.isDisposed()) {
button.setText("Updated"); // Accessing disposed UI element
}
}
});
In the above example, checking whether the UI element button
is disposed within asyncExec
doesn't provide complete safety when accessing the element.
Correct Usage:
display.asyncExec(new Runnable() {
public void run() {
if (button != null && !button.isDisposed()) {
button.setText("Updated"); // Safe access with null check and disposal check
}
}
});
By performing a null check and verifying the element's disposal status, you can safely access UI elements within asyncExec
.
Best Practices
To avoid the pitfalls mentioned above and ensure safe usage of asyncExec
, consider the following best practices:
-
Encapsulate UI Updates: Encapsulate UI updates within dedicated methods to be executed using
asyncExec
, promoting reusability and maintainability. -
Use Data Transfer Objects (DTOs): When passing data to the UI thread using
asyncExec
, consider using immutable DTOs to prevent concurrent modification issues. -
Avoid Lengthy Tasks: Ideally,
asyncExec
should handle quick UI updates. For lengthy tasks, consider usingsyncExec
or background threads to avoid UI unresponsiveness. -
Unit Testing: Unit test code blocks within
asyncExec
to ensure they behave as expected in the UI thread. -
Code Reviews: Conduct code reviews to identify potential pitfalls in
asyncExec
usage and share best practices among team members.
To Wrap Things Up
Correct usage of asyncExec
is crucial for developing robust and responsive SWT-based applications. By understanding common pitfalls and adhering to best practices, developers can ensure the safe and effective execution of UI-related code in the UI thread. Remember to encapsulate UI updates, handle disposed UI elements, and avoid accessing non-final variables within asyncExec
to maintain a stable and predictable UI behavior.
Refer to the SWT Documentation for further insights into SWT development, as well as sample applications and code snippets. By following these guidelines, you can harness the full potential of asyncExec
while sidestepping common pitfalls, ensuring a seamless and engaging user experience in your SWT Java applications.
Checkout our other articles