Maximizing JVM Performance Through Effective Garbage Collection
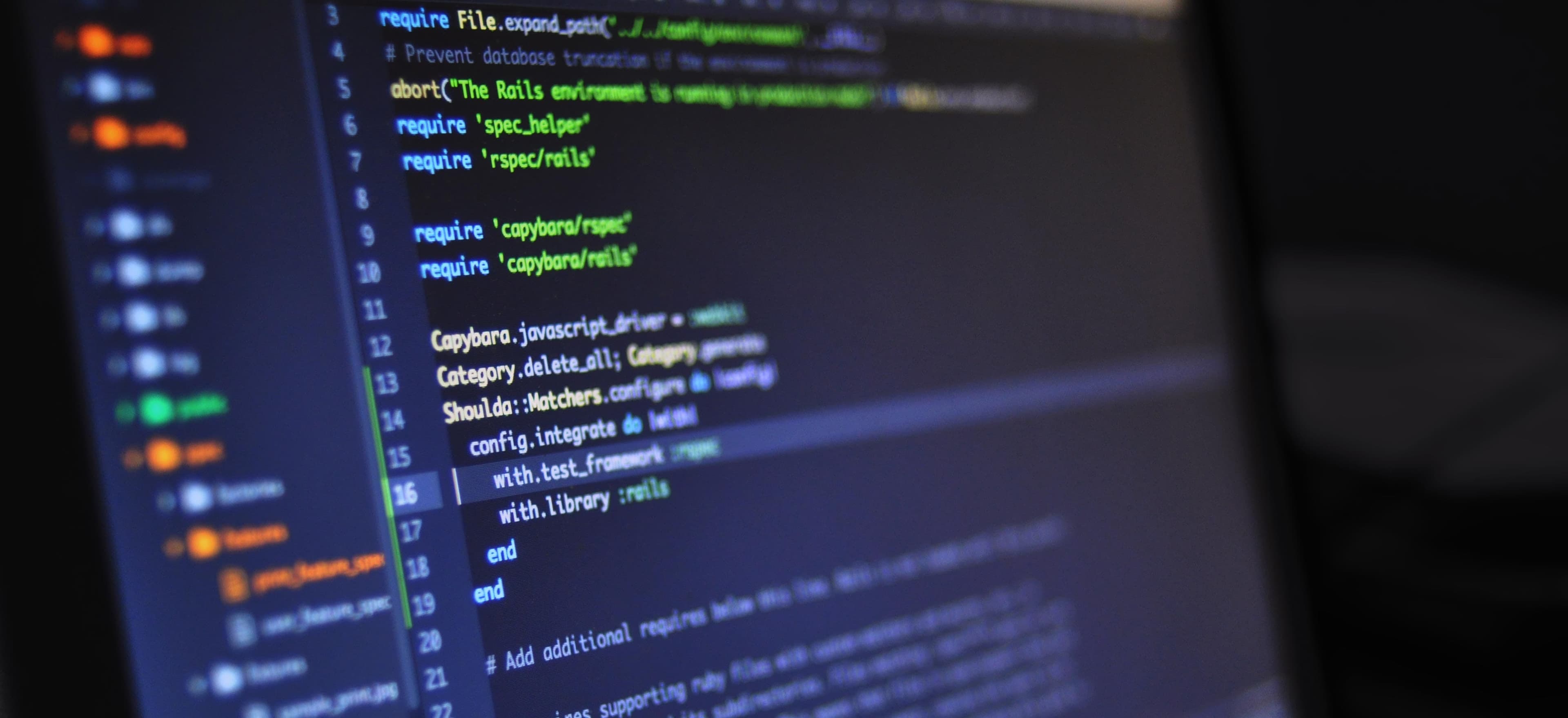
- Published on
Maximizing JVM Performance Through Effective Garbage Collection
When it comes to Java application performance, effective memory management is crucial. The Java Virtual Machine (JVM) manages memory through a process called Garbage Collection (GC). GC is responsible for reclaiming memory occupied by objects that are no longer in use, thus preventing memory leaks and maintaining optimal performance.
In this blog post, we'll delve into the fundamentals of GC, explore different GC algorithms, and provide best practices for tuning GC to maximize JVM performance.
Understanding Garbage Collection
In Java, memory is allocated to objects on the heap. As the program runs, objects are created and eventually become unreachable, either due to going out of scope or being explicitly set to null. These unreachable objects consume memory and need to be reclaimed to prevent memory exhaustion.
Garbage Collection is the process of identifying and reclaiming memory occupied by unreachable objects. This process involves the following steps:
- Marking: Traversing the object graph from root objects to identify reachable objects.
- Sweeping: Reclaiming memory occupied by unreachable objects.
- Compacting: Rearranging the memory to reduce fragmentation.
Garbage Collection Algorithms
1. Serial Garbage Collector
The Serial Garbage Collector (-XX:+UseSerialGC
) uses a single thread for young generation collection, making it suitable for small applications or environments with limited resources.
2. Parallel Garbage Collector
The Parallel Garbage Collector (-XX:+UseParallelGC
) uses multiple threads for young generation collection, leveraging multiple cores for garbage collection. It is suitable for multi-core machines and can significantly reduce GC pause times.
3. CMS Garbage Collector
The Concurrent Mark-Sweep (CMS) Collector (-XX:+UseConcMarkSweepGC
) reduces pause times by performing most of the GC work concurrently with the application threads. This makes it suitable for large-scale applications where low pause times are crucial.
4. G1 Garbage Collector
The Garbage-First (G1) Collector (-XX:+UseG1GC
) is designed to provide more predictable pause times and to operate efficiently on large heaps. It divides the heap into regions and uses parallelism to achieve both young and old generation collection.
Each GC algorithm has its own advantages and trade-offs. Choosing the right GC algorithm depends on various factors such as application size, available resources, and performance requirements.
Garbage Collection Tuning
Tuning GC involves adjusting parameters to optimize the trade-offs between throughput, latency, and memory footprint. Here are some best practices for GC tuning:
-
Choose the Right GC Algorithm: Understand the characteristics of each GC algorithm and choose the one that best suits your application's requirements.
-
Monitor GC Activity: Use tools like VisualVM, Java Mission Control, or Prometheus with Grafana to monitor GC activity and identify patterns.
-
Adjust Heap Size: Set the initial and maximum heap sizes based on the application's memory requirements to avoid frequent GC cycles.
-
Tune GC Parameters: Adjust parameters such as young generation size, survivor space ratio, and GC pause time goals to achieve optimal performance.
Example: Tuning G1 GC Parameters
-XX:G1HeapRegionSize=4M
-XX:MaxGCPauseMillis=200
-XX:ParallelGCThreads=20
In this example, we set the G1 heap region size to 4MB, configure the maximum GC pause time to 200 milliseconds, and use 20 parallel GC threads for concurrent GC.
-
Avoid Premature Optimization: Measure the performance impact of GC tuning changes before applying them in a production environment to avoid premature optimization.
-
Profile Application Code: Identify memory-intensive or long-lived objects in the application code and optimize their usage to reduce GC pressure.
Closing the Chapter
Effective garbage collection is essential for maximizing JVM performance. Understanding different GC algorithms, tuning GC parameters, and monitoring GC activity are key steps in achieving optimal memory management and overall application performance.
By following best practices for GC tuning and continuously monitoring and optimizing GC activity, Java applications can achieve better responsiveness, lower latency, and improved overall throughput.
Optimizing Garbage Collection is a continuous process, requiring a deep understanding of the application's memory behavior and performance requirements. With the right approach to GC tuning, Java applications can achieve optimal performance and scalability.
Remember, the key to effective GC tuning is to measure, analyze, and iterate based on real performance data, ensuring that the chosen configuration aligns with the application's specific needs.
In conclusion, efficient garbage collection is fundamental in unleashing the full potential of Java applications. By following best practices and understanding the intricacies of GC algorithms, developers can elevate their applications to new levels of performance and efficiency.
For further reading on Java performance optimization and JVM tuning, check out Oracle's Garbage Collection Introduction guide and Understanding Java Garbage Collection by Baeldung.
Remember, the right GC strategy can be a game-changer for Java applications, minimizing downtime, enhancing user experience, and maximizing resource utilization.
Let's continue to unlock the potential of our Java applications through effective garbage collection and performance optimization!