Mastering CDI: Navigating Transactional Exception Handling
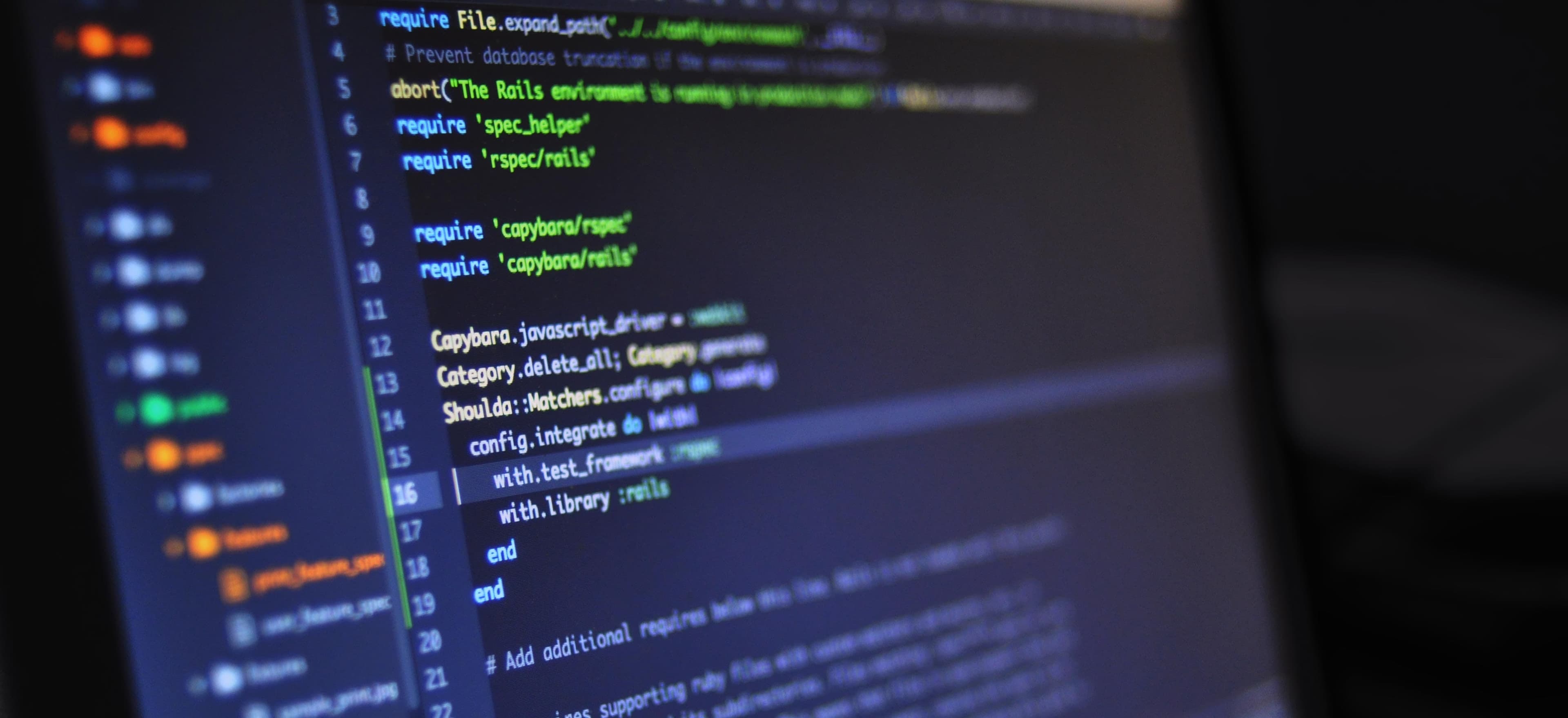
- Published on
Mastering CDI: Navigating Transactional Exception Handling
CDI, or Contexts and Dependency Injection, is a powerful feature of Java EE that allows for the injection of dependencies and management of contextual lifecycle in a Java application. One critical aspect of managing dependencies is handling transactions and exceptions within a CDI environment.
In this blog post, we will delve into the best practices for managing transactions and handling exceptions in CDI, equipping you with the knowledge and tools to navigate these crucial aspects of application development.
Setting the Stage: Understanding CDI Contexts
Before jumping into transactional exception handling, let's quickly refresh our understanding of CDI contexts. CDI manages contextual instances of beans, allowing for the injection of dependencies and the management of their lifecycle.
CDI defines several contexts, including the application context, request context, session context, and conversation context. Each context has a well-defined lifecycle, and CDI ensures that the beans behave accordingly within these contexts.
Transaction Management with CDI
When dealing with transactional operations within a CDI-managed application, it's essential to understand how CDI integrates with transaction management. CDI seamlessly integrates with Java EE's powerful transaction management capabilities, allowing for declarative transaction demarcation.
Declarative Transaction Demarcation
In CDI, transaction demarcation is achieved through annotations such as @Transactional
to specify the transactional behavior of a method. By annotating a method with @Transactional
, you can indicate that the method should be executed within a transactional context.
Let's consider an example:
import javax.transaction.Transactional;
@ApplicationScoped
public class ProductService {
@Inject
private EntityManager entityManager;
@Transactional
public void updateProduct(Product product) {
entityManager.merge(product);
}
}
In this example, the updateProduct
method is annotated with @Transactional
, indicating that it should be executed within a transactional context. This declarative approach simplifies the management of transactions within CDI-managed beans.
Transaction Propagation
Transaction propagation specifies how transactions are propagated when a method is called. CDI offers support for various transaction propagation types, including REQUIRED
, REQUIRES_NEW
, SUPPORTS
, and NEVER
, allowing you to define the transactional behavior based on the specific requirements of your application.
Exception Handling in CDI
Exception handling is a critical aspect of application development, and CDI provides mechanisms for handling exceptions within its managed beans.
Exception Handling with Interceptors
CDI's interceptor mechanism enables you to implement cross-cutting concerns such as exception handling. By defining an interceptor that captures and handles exceptions, you can centralize the exception handling logic, promoting code reusability and maintainability.
Let's take a look at an example of using CDI interceptors for exception handling:
@Interceptor
public class ExceptionHandlerInterceptor {
@AroundInvoke
public Object handleException(InvocationContext context) throws Exception {
try {
return context.proceed();
} catch (Exception e) {
// Exception handling logic
log.error("An error occurred: " + e.getMessage());
throw e; // Re-throw the exception
}
}
}
In this example, the ExceptionHandlerInterceptor
intercepts method invocations and provides a mechanism for handling exceptions. By centralizing exception handling logic within an interceptor, you can ensure consistent handling across multiple beans.
Conditional Exception Handling
CDI allows for conditional exception handling through the use of qualifiers. Qualifiers enable you to selectively apply interception based on specific conditions, allowing for fine-grained control over exception handling.
Here's an example of using qualifiers for conditional exception handling:
@Qualifier
@Target({ElementType.TYPE, ElementType.METHOD, ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
public @interface LoggedException {
}
@Interceptor
@LoggedException
public class LoggedExceptionInterceptor {
@AroundInvoke
public Object handleLoggedException(InvocationContext context) throws Exception {
try {
return context.proceed();
} catch (Exception e) {
log.error("Logged exception: " + e.getMessage());
throw e;
}
}
}
In this example, the LoggedException
qualifier is used to selectively apply the LoggedExceptionInterceptor
for handling exceptions. By leveraging qualifiers, you can target specific methods or beans for customized exception handling behavior.
Putting It All Together: Practical Considerations
When working with CDI, there are several practical considerations to keep in mind for effective transaction management and exception handling.
Avoiding Transactional Annotations on Private Methods
It's important to note that placing the @Transactional
annotation on private methods within a CDI-managed bean may not yield the expected transactional behavior. CDI operates based on proxies, and private methods are not accessible through proxies, leading to the transactional annotation being bypassed.
Using Bean Validation for Robust Error Handling
Utilize Java Bean Validation (JSR 380) to enforce robust error handling within your CDI-managed beans. Bean validation annotations such as @NotNull
, @Size
, and @Valid
can be used to ensure that the input parameters and data satisfy the required constraints, contributing to more resilient exception handling.
Leveraging DeltaSpike Exception Control for Advanced Exception Handling
For advanced exception handling requirements, consider leveraging Apache DeltaSpike with its Exception Control mechanism. DeltaSpike provides a powerful set of tools for handling exceptions, including the ability to define exception handlers and manage exception control flow with ease.
The Last Word
In this blog post, we explored the intricacies of transaction management and exception handling within a CDI environment. By leveraging declarative transaction demarcation, interceptors, and qualifiers, you can effectively manage transactions and handle exceptions in a clear and concise manner.
Mastering CDI's transactional exception handling capabilities empowers you to build robust and resilient applications, where the management of transactions and handling of exceptions are seamlessly integrated into the application's architecture.
As you continue to refine your skills in Java EE application development, harnessing the power of CDI for transactional exception handling will undoubtedly contribute to the robustness and reliability of your applications.
Remember, embracing best practices for transaction management and exception handling in CDI sets the stage for the development of high-quality, enterprise-grade Java applications.
So, go forth with confidence, armed with the knowledge to navigate the complexities of transactional exception handling with CDI, and elevate your Java EE development expertise to new heights.