Overcoming Integration Hurdles for Seamless App Synergy
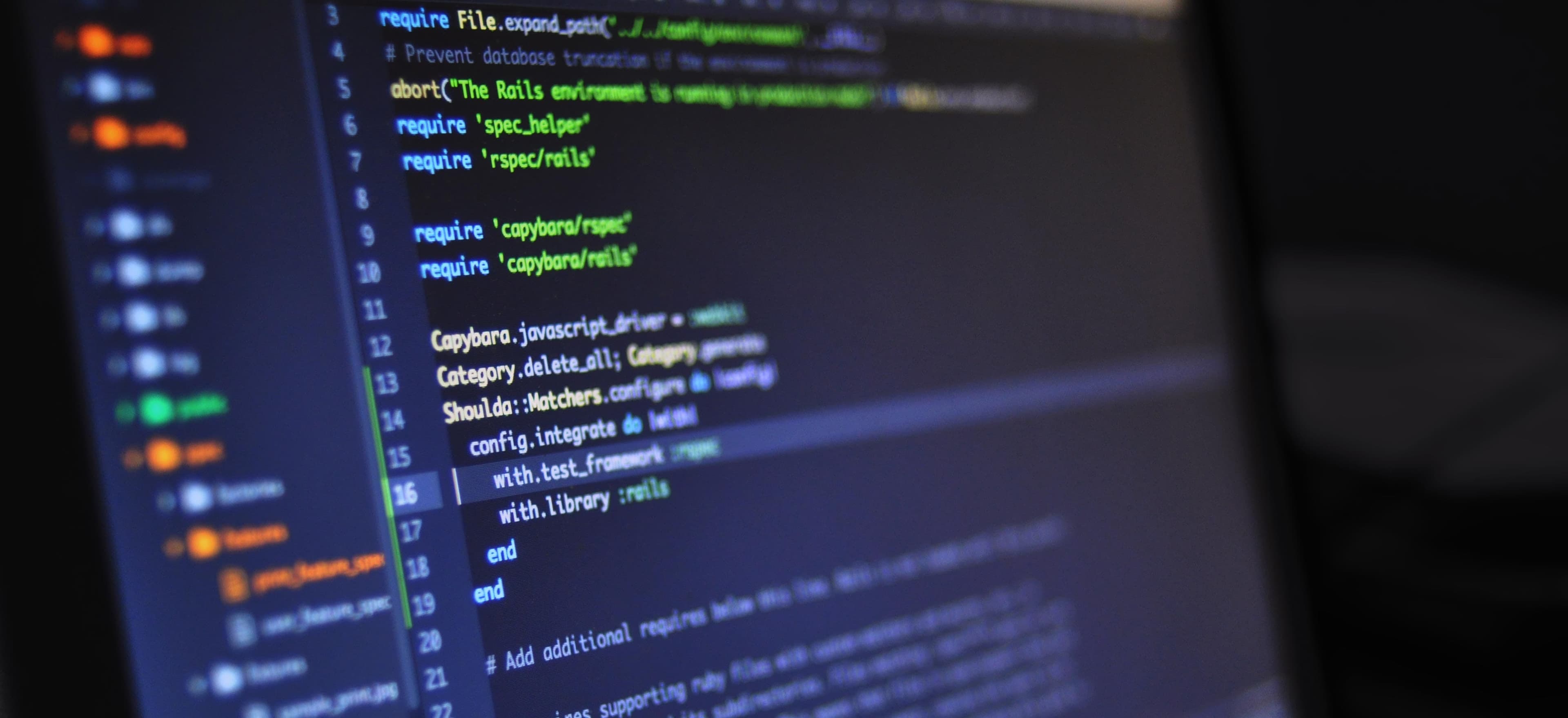
- Published on
Overcoming Integration Hurdles for Seamless App Synergy
In today's fast-paced technological landscape, the ability of software applications to seamlessly integrate with one another is essential for businesses striving for operational efficiency and enhanced user experiences. Java, with its robust and versatile nature, plays a pivotal role in this integration process. In this article, we will delve into the challenges faced during app integration and how Java, with its vast array of tools and libraries, can be leveraged to overcome these hurdles, ensuring a seamless synergy between different applications.
The Challenges of App Integration
App integration involves connecting different software applications and systems to function harmoniously, enabling data and processes to flow between them. However, various challenges can impede this harmonious integration:
-
Diverse Technologies: Applications may be built using different programming languages, frameworks, or data storage systems, making it complex to establish seamless communication.
-
Data Synchronization: Ensuring that data is synchronized and up-to-date across integrated applications is a critical challenge, particularly when dealing with large volumes of real-time data.
-
Security Concerns: Integrating applications often raises security issues, as it involves the sharing of sensitive data and the potential exposure of vulnerabilities.
-
Scalability and Performance: As the integrated system grows, maintaining optimal performance and scalability becomes increasingly challenging.
Leveraging Java for Seamless Integration
Java, with its strong emphasis on portability, robustness, and extensive ecosystem, provides a solid foundation for addressing the challenges of app integration.
Java's Versatile Integration Tools and Libraries
Java offers a plethora of integration tools and libraries that facilitate seamless communication between applications. Some notable tools include:
-
Java Database Connectivity (JDBC): JDBC enables Java applications to interact with various databases, providing a standardized method for managing relational databases.
-
Java Message Service (JMS): JMS is a messaging standard that allows Java applications to asynchronously send messages and receive messages.
-
Spring Integration: Part of the larger Spring ecosystem, Spring Integration provides a framework for building messaging and event-driven architecture within Java applications.
-
Apache Camel: Apache Camel is an open-source integration framework that provides a rule-based routing and mediation engine to facilitate the integration of disparate systems.
Sample Code - Leveraging Java Message Service (JMS)
import javax.jms.*;
import javax.naming.InitialContext;
public class JMSExample {
public static void main(String[] args) throws Exception {
InitialContext context = new InitialContext();
ConnectionFactory connectionFactory = (ConnectionFactory) context.lookup("jms/ConnectionFactory");
Destination destination = (Destination) context.lookup("jms/queue/myQueue");
try (Connection connection = connectionFactory.createConnection()) {
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
MessageProducer producer = session.createProducer(destination);
TextMessage message = session.createTextMessage("Hello, this is a JMS example!");
producer.send(message);
}
}
}
In this sample code, we demonstrate the use of JMS within a Java application to send a message to a JMS queue. JMS plays a pivotal role in enabling asynchronous communication between distributed applications, providing a robust foundation for app integration.
Embracing Microservices Architecture with Java
Microservices, a popular architectural style for building applications as a collection of loosely coupled services, further emphasize the need for seamless integration. Java, with frameworks like Spring Boot and MicroProfile, provides a robust ecosystem for developing and integrating microservices.
In a sample microservices integration scenario, a Java-based service developed with Spring Boot can communicate with other microservices via RESTful APIs, leveraging JSON or XML for data interchange.
Addressing Data Synchronization Challenges with Java
Data synchronization is a critical aspect of app integration, especially when dealing with real-time data across disparate systems. Java offers robust solutions for addressing data synchronization challenges, such as:
-
Change Data Capture (CDC): Tools like Debezium, which integrates with databases like MySQL and MongoDB, capture and propagate data changes in real-time, enabling seamless synchronization between diverse data sources.
-
Apache Kafka: Kafka, a distributed streaming platform, is widely used for building real-time data pipelines and streaming applications, facilitating the seamless flow of data across integrated systems.
Java, with its strong support for both Debezium and Kafka through dedicated client libraries, emerges as a potent tool for addressing data synchronization challenges in app integration scenarios.
Ensuring Security in Java-Based App Integration
Security is paramount in app integration, particularly when sensitive data is exchanged between applications. Java, known for its robust security features, provides various mechanisms to ensure secure app integration:
-
Java Authentication and Authorization Service (JAAS): JAAS provides a framework for user authentication and authorization, enabling secure access control for integrated applications.
-
SSL/TLS Support: Java's robust support for SSL/TLS ensures secure communication between integrated applications over the network, mitigating potential security vulnerabilities.
-
Security Libraries: Java offers a wide array of security libraries, such as Apache Shiro and Spring Security, which can be seamlessly integrated into Java-based applications to enforce authentication, authorization, and data encryption.
By integrating these security mechanisms, Java empowers developers to build a secure integration layer that safeguards sensitive data during app integration processes.
Scaling and Performance Considerations with Java
As integrated systems expand, maintaining optimal performance and scalability becomes crucial. Java, with its focus on performance optimization and scalability, provides various tools and techniques to address these considerations:
-
Java Virtual Machine (JVM) Tuning: JVM offers a range of tuning options, such as garbage collection settings and heap memory management, to optimize performance and ensure efficient resource utilization.
-
Caching Strategies: Java-based caching solutions like Ehcache and Hazelcast provide in-memory data storage and retrieval, enhancing performance by reducing the need to frequently access external data sources during integration.
-
Asynchronous Processing: Leveraging Java's support for asynchronous processing, developers can design integration solutions that efficiently handle concurrent operations, thereby improving overall system scalability.
By implementing these strategies, Java-based integrated systems can maintain optimal performance and scale seamlessly as the demands on the system grow.
The Last Word
In a digital landscape where the integration of diverse applications is pivotal for modern businesses, Java emerges as a powerful ally in overcoming the challenges of seamless app synergy. With its rich ecosystem of integration tools, robust support for microservices, data synchronization capabilities, security mechanisms, and performance optimization techniques, Java empowers developers to build integrated systems that operate harmoniously, delivering enhanced value to businesses and end users alike. Embracing Java for app integration not only addresses the complexities of integration but also paves the way for a more agile, efficient, and interconnected digital ecosystem.
By understanding the challenges of app integration and harnessing the strengths of Java, businesses can propel themselves towards a future where their diverse applications work together seamlessly, driving innovation, productivity, and competitive advantage.
Checkout our other articles