Troubleshooting Microprofile Configurations in Apache TomEE
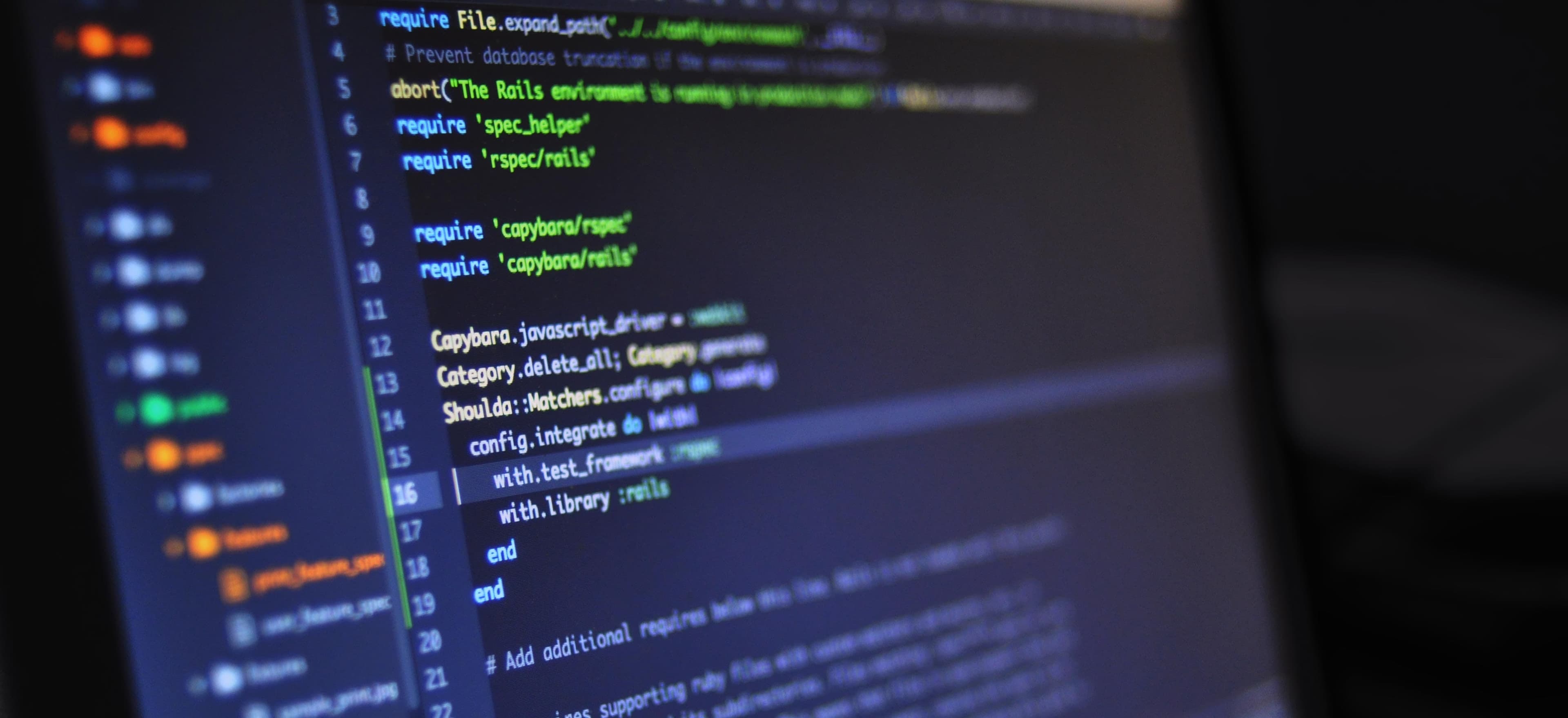
- Published on
Troubleshooting Microprofile Configurations in Apache TomEE
Microprofile has gained popularity as a programming model for building microservices-based applications. Apache TomEE, a lightweight enterprise version of Tomcat, supports Microprofile specifications, making it an attractive choice for developing microservices. However, configuring Microprofile in TomEE can sometimes be challenging, and troubleshooting configuration issues is a crucial aspect of the development process.
In this article, we'll explore some common configuration issues when working with Microprofile in Apache TomEE and how to troubleshoot them effectively.
1. Checking Microprofile Feature Availability
Before troubleshooting any configuration issues, it's important to ensure that the Microprofile feature is available in the TomEE server. Microprofile is supported in TomEE by adding the appropriate feature using the tomee.xml
configuration file.
<tomee>
<tomee-xml>
<tomee>
<Service>
// Add Microprofile feature here
</Service>
</tomee>
</tomee-xml>
</tomee>
Ensure that the tomee.xml
file contains the necessary configuration to enable Microprofile features. If it's not present, add the Microprofile feature to the configuration and restart the server to apply the changes.
2. Resolving Classpath Issues
When working with Microprofile in Apache TomEE, classpath issues can often arise, leading to configuration problems. It's essential to verify that the required Microprofile libraries are included in the application's classpath.
Ensure the microprofile-config-api-2.x.jar
(replace 2.x
with the appropriate version) is included in the application's WEB-INF/lib
directory. Additionally, verify that the required dependencies are correctly specified in the application's pom.xml
if using Maven or build.gradle
if using Gradle.
<dependency>
<groupId>org.eclipse.microprofile.config</groupId>
<artifactId>microprofile-config-api</artifactId>
<version>2.x</version>
</dependency>
This ensures that the necessary Microprofile libraries are available to the application at runtime, resolving classpath issues that could affect configuration.
3. Debugging Configuration Properties
Debugging configuration properties is an integral part of troubleshooting Microprofile configuration issues. Use logging frameworks such as SLF4J or Jakarta Commons Logging to output the configuration properties at runtime.
import org.eclipse.microprofile.config.inject.ConfigProperty;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyConfigurableClass {
private static final Logger logger = LoggerFactory.getLogger(MyConfigurableClass.class);
@Inject
@ConfigProperty(name = "my.config.property")
private String myConfigProperty;
public void someMethod() {
logger.info("Value of my.config.property: " + myConfigProperty);
}
}
By logging the configuration properties, you can verify if the properties are being loaded correctly and if their values are as expected. This form of debugging provides valuable insights into the configuration behavior at runtime.
4. Validating Configuration Sources
Microprofile configuration properties can be sourced from various providers such as environment variables, system properties, and configuration files. It's essential to validate that the configuration sources are correctly configured and accessible to the application.
Check the order of configuration sources to ensure that the application is reading properties from the intended provider. Additionally, verify the precedence of configuration sources to determine which values take precedence in case of conflicts.
Understanding the precedence and order of configuration sources helps in diagnosing issues related to incorrect property values being picked up by the application.
5. Handling Configuration Location
Microprofile allows configuring the location of configuration files using the MP_CONFIG_DIR
and MP_CONFIG_FILE
environment variables. If the application is not picking up the expected configuration files, ensure that these environment variables are correctly set.
export MP_CONFIG_DIR=/path/to/config/directory
export MP_CONFIG_FILE=my-config.properties
Set the MP_CONFIG_DIR
to the directory containing the configuration files, and specify the MP_CONFIG_FILE
to point to the desired configuration file. This ensures that the application picks up the configurations from the specified location.
6. Using Health Checks
Microprofile Health Checks provide a way to verify the status of a microservice and its dependencies. Integrate health checks in the application to monitor the status of the configuration sources and properties.
@Health
public class ConfigurationHealthCheck implements HealthCheck {
@Override
public HealthCheckResponse call() {
// Check the status of configuration sources and properties
// Return a HealthCheckResponse based on the status
}
}
By implementing custom health checks, you can assess the health of the configuration sources and properties, identifying any potential issues that may affect the application's behavior.
Bringing It All Together
Troubleshooting Microprofile configurations in Apache TomEE requires a systematic approach to identify and resolve issues effectively. By ensuring the availability of Microprofile features, addressing classpath issues, debugging configuration properties, validating configuration sources, handling configuration location, and using health checks, developers can effectively troubleshoot and resolve configuration problems.
Remember to consult the Microprofile documentation and the Apache TomEE documentation for detailed information on configuration and troubleshooting.
By following these best practices and leveraging the troubleshooting techniques discussed in this article, developers can streamline the process of identifying and resolving Microprofile configuration issues in Apache TomEE.
Checkout our other articles