Mastering serialVersionUID: Boost Your Java Serialization
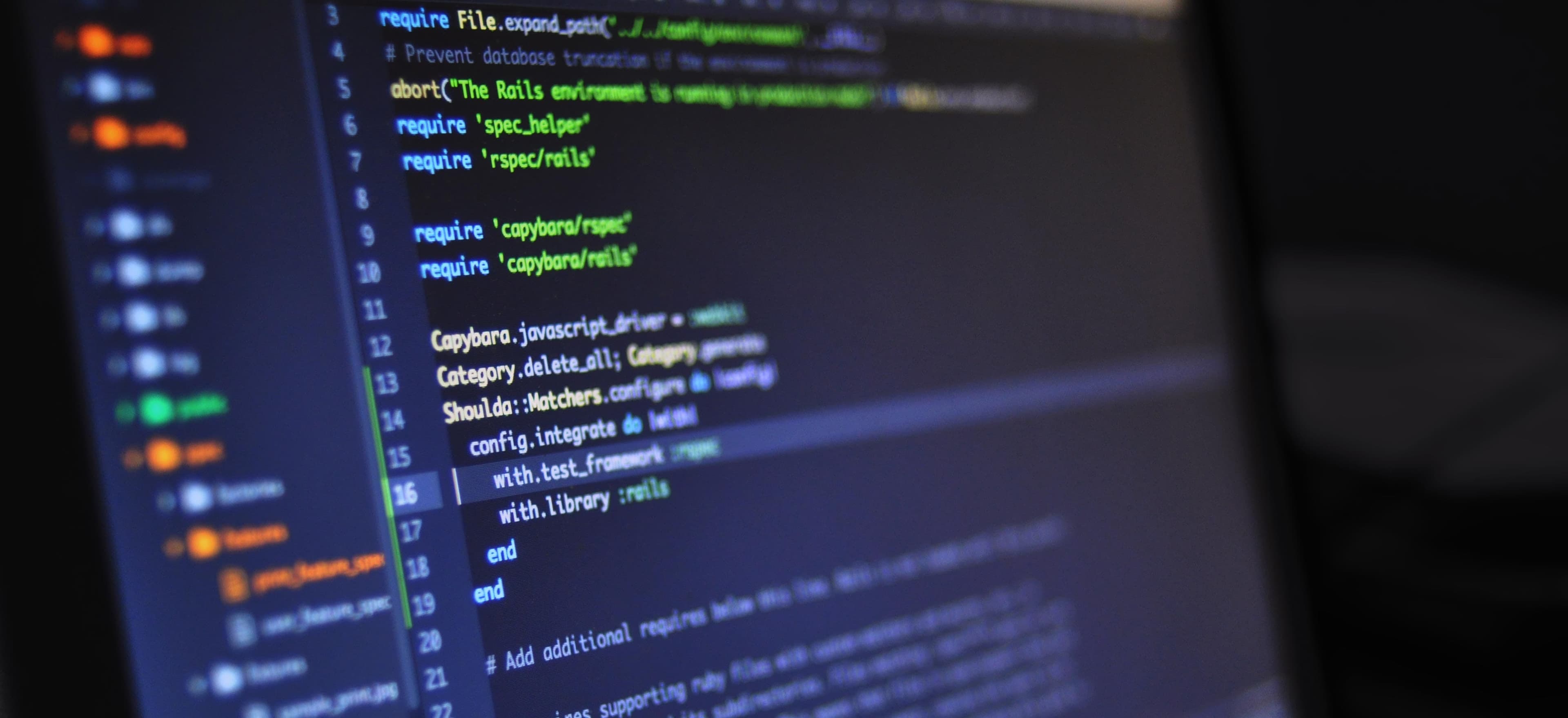
- Published on
Mastering serialVersionUID: Boost Your Java Serialization
Serialization in Java is a powerful mechanism that allows objects to be converted into a stream of bytes for storage or transmission and later restored to their original state. However, it comes with its own challenges, one of them being versioning.
When a serialized object is restored, the JVM checks if the class that was used during serialization is the same as the class during deserialization. If the classes differ, then an InvalidClassException
is thrown.
Here's where the serialVersionUID
comes into play. It's a unique identifier for each version of a Serializable class to ensure that the same class (and not a different version) is used during deserialization. In this blog post, we will delve into the importance of serialVersionUID
and how to effectively manage it.
Understanding serialVersionUID
The serialVersionUID
is a field present in a Serializable class. When an object of a Serializable class is serialized, this unique identifier is also written to the stream. During deserialization, the JVM compares the serialVersionUID
of the serialized object with that of the loaded class. If they do not match, an InvalidClassException
is thrown.
Importance of serialVersionUID
Backward and Forward Compatibility
By explicitly declaring a serialVersionUID
, developers can control the versioning of their classes. This is crucial for backward and forward compatibility of serialized objects. If a change is made to the class that does not affect the compatibility of serialization, the serialVersionUID
can remain the same, ensuring that previously serialized objects can still be deserialized successfully.
Distributed Systems
In distributed systems where different components may be running different versions of the same class, having a consistent serialVersionUID
is essential. It allows for seamless communication and interoperability between different versions of the class.
Managing serialVersionUID
Now, let's look at some best practices for managing the serialVersionUID
in your Serializable classes.
Declaring serialVersionUID
private static final long serialVersionUID = 1L;
When you declare the serialVersionUID
, it's important to understand that it should be declared private
, static
, and final
. It ensures that the serialVersionUID
is associated with the class itself and not with any particular instance. The value can be any long, and it's recommended to use a value of type long
to make it explicit and avoid any accidental integer overflows.
Updating serialVersionUID
When making a non-compatible change to a class, such as adding or removing a field, it's crucial to update the serialVersionUID
to indicate that the new version is incompatible with the old one. Failure to do so would result in an InvalidClassException
during deserialization.
IDE Support
Many modern IDEs provide support for automatically generating and updating the serialVersionUID
for Serializable classes. For example, in IntelliJ IDEA, you can use the "Generate Serial Version UID" quick fix to generate the serialVersionUID
or update it when the class structure changes.
Best Practices
-
Always declare a
serialVersionUID
for Serializable classes, even if the class doesn't currently have any serializable fields. This ensures that theserialVersionUID
is in place if the class structure changes in the future. -
When updating the class in a way that may affect serialization, increment the
serialVersionUID
to indicate the change.
The Last Word
In conclusion, mastering the serialVersionUID
is crucial for effectively managing the versioning of Serializable classes in Java. By understanding its importance and following best practices for declaring and updating it, developers can ensure smooth and seamless serialization and deserialization of objects, even in distributed and evolving systems.
By implementing these best practices and understanding the significance of serialVersionUID
, you can boost your Java serialization and ensure compatibility and consistency across different versions of your Serializable classes.
To dive deeper into Java serialization and versioning, refer to the official Java documentation and explore how different strategies can be used to handle versioning. Happy coding!
Checkout our other articles