Master JBoss Forge & NetBeans: A Quick Start Guide
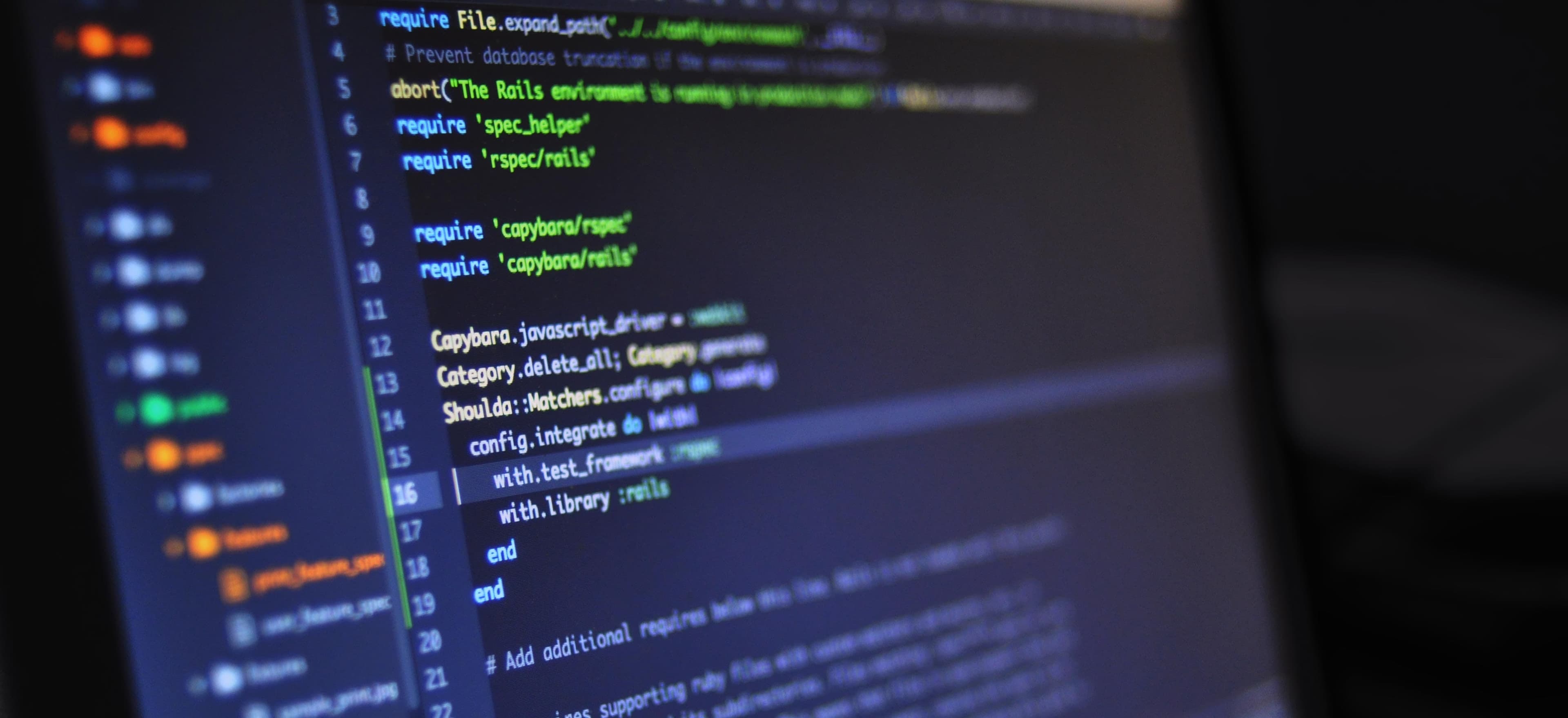
- Published on
Master JBoss Forge & NetBeans: A Quick Start Guide
Are you looking to streamline your Java development process and increase productivity? Look no further than JBoss Forge and NetBeans. In this guide, I will walk you through the basics of JBoss Forge, a powerful code generation tool, and how you can integrate it seamlessly with NetBeans, one of the most popular Java IDEs. Let's dive in and learn how to master these tools to supercharge your Java development workflow.
What is JBoss Forge?
JBoss Forge is a powerful and extensible code generation tool for Java. It allows developers to create, modify, and scaffold Java applications with ease. By using Forge, developers can focus on writing business logic instead of boilerplate code. It provides a set of commands that can be executed from the command line or within an IDE to automate repetitive tasks, such as generating entities, REST endpoints, and more.
Setting Up JBoss Forge with NetBeans
To get started with JBoss Forge and NetBeans, you first need to install JBoss Forge and the NetBeans Forge plugin. Once you have both tools installed, you can seamlessly integrate JBoss Forge into your NetBeans workflow.
Step 1: Install JBoss Forge
You can download JBoss Forge from the official website or use a build tool like Maven to include it as a dependency in your project. Once you have JBoss Forge installed, you can start using its powerful code generation capabilities.
Step 2: Install NetBeans Forge Plugin
NetBeans Forge Plugin allows you to use JBoss Forge within the NetBeans IDE. You can install the Forge plugin directly from the NetBeans Plugin Manager. Once installed, you can access JBoss Forge commands directly from within NetBeans.
Using JBoss Forge with NetBeans
Now that you have JBoss Forge and NetBeans set up, let's explore some practical examples of how you can use JBoss Forge to streamline your Java development process within the NetBeans IDE.
Generating Entities
One of the most common tasks in Java development is creating entity classes for your application. With JBoss Forge, you can generate these entities quickly and easily. Let's take a look at an example of how to generate a simple User
entity class using JBoss Forge within NetBeans.
package com.example.entity;
public class User {
private Long id;
private String username;
private String email;
// Getters and setters
}
Now, let's use JBoss Forge to generate this entity class within NetBeans. Simply execute the following command in the JBoss Forge console:
entity --named User --named id --type Long --named username --type String --named email --type String
This command will generate a User
entity class with the specified fields in your NetBeans project. By using JBoss Forge, you can save time and avoid writing boilerplate code for entity classes.
Generating REST Endpoints
Another common task in modern Java development is creating REST endpoints for your application. JBoss Forge makes this task a breeze. Let's see how you can generate a REST endpoint for the User
entity we created earlier using JBoss Forge within NetBeans.
package com.example.rest;
@Path("/users")
public class UserResource {
@Inject
private UserService userService;
@GET
@Path("/{id}")
public Response getUser(@PathParam("id") Long id) {
// Retrieve user from the database
}
@POST
public Response createUser(User user) {
// Create a new user
}
// Other endpoints
}
To generate this REST endpoint using JBoss Forge, execute the following command in the JBoss Forge console:
rest setup --activator User
This will generate the necessary REST endpoint code for the User
entity in your NetBeans project. With JBoss Forge, you can consistently and efficiently generate REST endpoints without the need to write repetitive code.
Lessons Learned
In this guide, we've explored the basics of JBoss Forge, a powerful code generation tool for Java, and how you can integrate it seamlessly with NetBeans to boost your productivity as a Java developer. By leveraging JBoss Forge's capabilities within NetBeans, you can save time, reduce boilerplate code, and focus on writing business logic. If you're looking to streamline your Java development workflow, mastering JBoss Forge and NetBeans is a valuable skill to have.
With the right tools and knowledge, you can take your Java development to the next level. Start incorporating JBoss Forge and NetBeans into your workflow today and experience the efficiency and productivity gains they offer.
Now that you have a solid understanding of JBoss Forge and NetBeans integration, it's time to put that knowledge into practice and explore the advanced features of these tools. Keep coding, keep learning, and keep exploring new ways to enhance your Java development experience!
Checkout our other articles