Boosting Microservices Speed with SparkJava & GraalVM
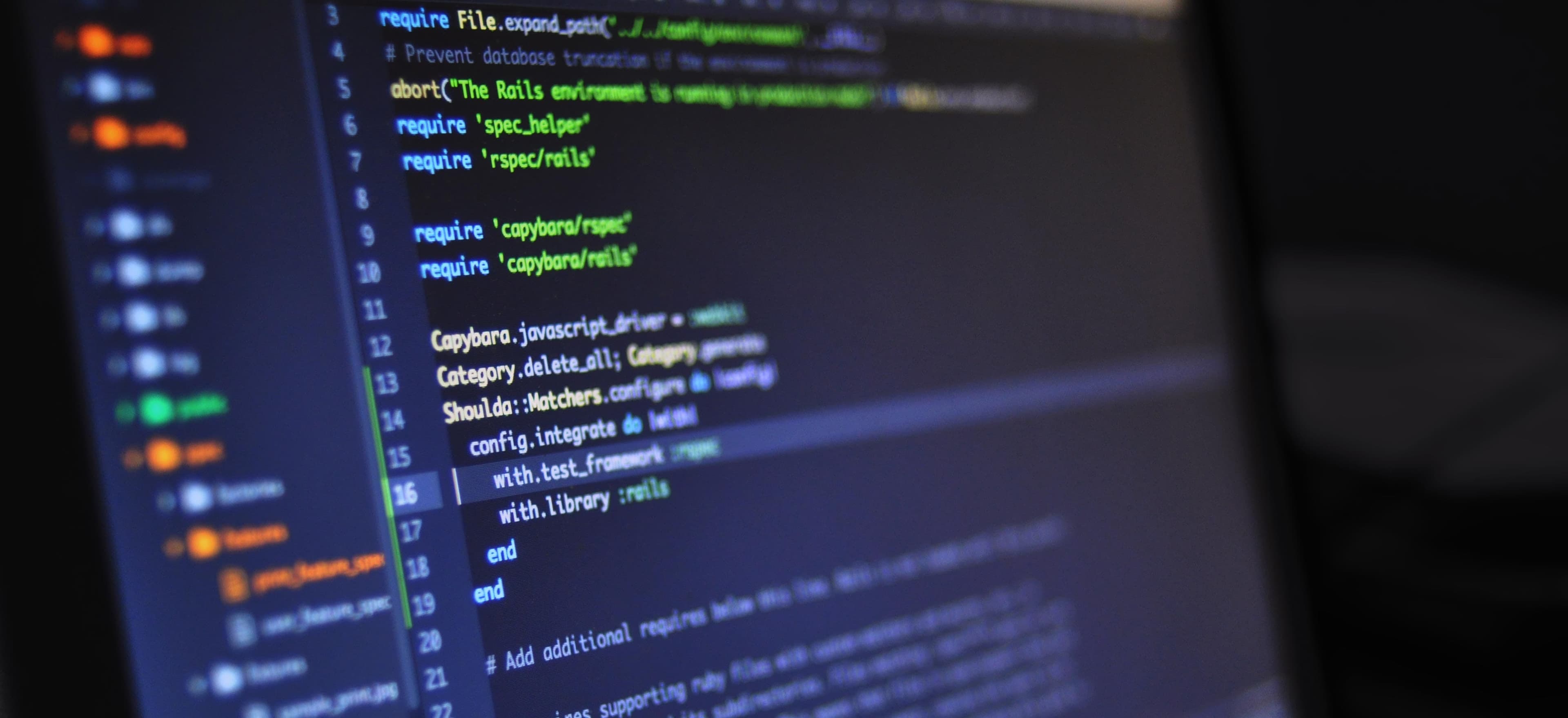
- Published on
Boosting Microservices Speed with SparkJava & GraalVM
In the fast-paced world of microservices, the need for speed and efficiency is paramount. Developers are constantly seeking ways to optimize the performance of their microservices, and one promising approach is leveraging the combination of SparkJava and GraalVM. In this article, we'll delve into how these two technologies can be employed to boost the speed and efficiency of microservices, and explore the benefits they offer.
What is SparkJava?
SparkJava is a lightweight, micro-framework for building web applications in Java. It is known for its simplicity and ease of use, making it an ideal choice for developing microservices. With its minimalistic approach, SparkJava allows developers to quickly create powerful RESTful web services with minimal configuration.
The Power of GraalVM
GraalVM is a universal virtual machine that offers high performance and the ability to run applications written in JavaScript, Python, Ruby, R, and other languages. One of the key features of GraalVM is its native image capability, which allows Java applications to be compiled ahead of time into native executables, resulting in faster startup times and reduced memory overhead.
Combining SparkJava with GraalVM can result in significant performance enhancements, making it an attractive choice for developing high-speed microservices.
Faster Startup Times with GraalVM's Native Image
GraalVM's native image capability plays a crucial role in enhancing the speed of microservices developed using SparkJava. By compiling the Java application into a native executable, GraalVM significantly reduces the startup time of the microservice. This is particularly beneficial in scenarios where microservices need to scale rapidly in response to fluctuating demands. The reduced startup time ensures that new instances of microservices can be brought online swiftly, contributing to overall system responsiveness.
Optimizing Microservice Performance with JIT Compilation
In addition to faster startup times, GraalVM's just-in-time (JIT) compilation capabilities contribute to optimizing the performance of microservices developed with SparkJava. The ability to dynamically adapt and optimize the running application code can lead to improved execution speed and reduced latency. This is particularly valuable in scenarios where microservices are expected to handle a high volume of concurrent requests, as it enables them to deliver speedy responses while efficiently utilizing system resources.
Leveraging Native Image to Reduce Memory Footprint
Another significant benefit of using GraalVM's native image capability with SparkJava is the reduction in memory footprint. The compilation of Java applications into native executables results in smaller memory overhead compared to traditional Java applications, which can contribute to more efficient resource utilization in microservices environments. The conservation of memory resources is particularly advantageous in cloud-based microservices architectures, where optimizing resource consumption is crucial for cost efficiency and scalability.
Embracing Polyglot Microservices with GraalVM's Language Support
In addition to its performance benefits, GraalVM enables developers to embrace polyglot microservices architectures by supporting multiple programming languages. This flexibility allows developers to leverage the strengths of different languages for specific microservices tasks, such as using JavaScript for front-end interactions or Python for data processing. By integrating GraalVM's support for multiple languages with SparkJava, developers can build polyglot microservices that are tailored to specific requirements, without sacrificing performance or speed.
Getting Started: Creating a Microservice with SparkJava and GraalVM
Now that we understand the benefits of combining SparkJava and GraalVM for microservice development, let's walk through the process of creating a simple microservice using these technologies.
Setting Up the Development Environment
Before we begin, ensure that you have the following prerequisites installed:
- Java Development Kit (JDK) 11 or later
- Apache Maven
Creating a SparkJava Project
To create a new SparkJava project, you can use Maven to generate a basic project structure:
mvn archetype:generate -DarchetypeGroupId=com.sparkjava -DarchetypeArtifactId=spark-java-archetype -DarchetypeVersion=1.0 -DgroupId=com.example -DartifactId=my-microservice -Dversion=1.0-SNAPSHOT
This command generates a new Maven project with the necessary dependencies for building a SparkJava-based microservice.
Adding Dependencies
Open the generated pom.xml
file and add the GraalVM native image plugin to the build configuration:
<build>
<plugins>
<plugin>
<groupId>org.graalvm.nativeimage</groupId>
<artifactId>native-image-maven-plugin</artifactId>
<version>21.2.0</version>
<executions>
<execution>
<goals>
<goal>native-image</goal>
</goals>
<configuration>
<!-- Configure native image options here -->
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
In addition to the native image plugin, include the SparkJava dependencies in the pom.xml
file:
<dependency>
<groupId>com.sparkjava</groupId>
<artifactId>spark-core</artifactId>
<version>2.9.3</version>
</dependency>
Developing a Simple Microservice
Create a new Java class to define the microservice endpoints using SparkJava:
import static spark.Spark.*;
public class Main {
public static void main(String[] args) {
port(8080);
get("/hello", (req, res) -> "Hello, Microservices!");
}
}
In this example, we define a simple /hello
endpoint that returns the text "Hello, Microservices!".
Building and Compiling the Native Image
To compile the microservice into a native image using GraalVM, execute the following Maven command:
mvn package -Pnative-image
This command triggers the native image compilation process, generating an executable that can be run directly without requiring a Java runtime environment.
The Bottom Line
In the realm of microservices development, speed and efficiency are essential components of success. By harnessing the power of SparkJava and GraalVM, developers can achieve significant performance improvements, faster startup times, reduced memory overhead, and the flexibility to build polyglot microservices. Armed with this potent combination, microservices can thrive in demanding, high-throughput environments, delivering seamless and responsive experiences to users.
As you embark on your journey to maximize the speed and efficiency of your microservices, consider adopting SparkJava and GraalVM to elevate the performance of your applications and pave the way for a resilient and high-performing microservices architecture.
Checkout our other articles