Updating Your 2015 Java Code: Avoid Obsolete Trends!
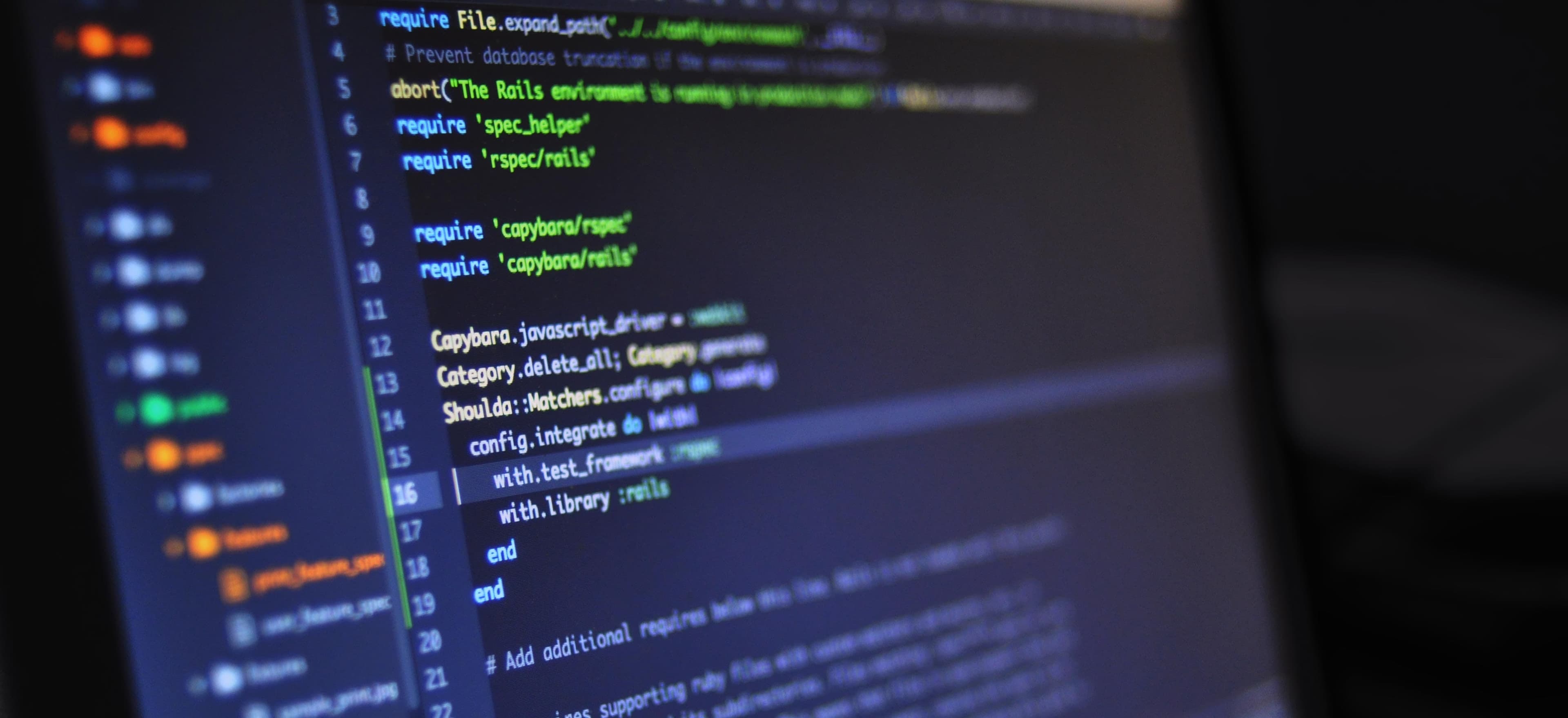
- Published on
Updating Your 2015 Java Code: Avoid Obsolete Trends!
The Java landscape has evolved tremendously over the past few years. With the release of new Java versions, from Java 8 to the cutting-edge Java 17, many practices that were once standard have become obsolete. If you're maintaining Java code that was written around 2015, it's high time to give your codebase a facelift. This guide will walk you through the essential updates, helping you keep your Java applications efficient, secure, and maintainable.
Why Update Your Java Code?
Updating your Java code from practices common in 2015 to those endorsed in 2023 might seem daunting. However, there are compelling reasons to do so:
- Performance Improvements: Newer Java versions have introduced optimizations that can significantly boost your application's performance.
- Security Enhancements: Outdated code can have vulnerabilities that expose your application to attacks. Keeping your code up-to-date strengthens your defense.
- Cleaner Code: Java's newer features can lead to more readable and maintainable code, making it easier for you and your team to manage.
Key Areas for Code Update
From for
Loops to Streams
Before:
In 2015, iterating over collections with for
loops was common practice:
List<String> filteredList = new ArrayList<>();
for (String s : myList) {
if (s.startsWith("J")) {
filteredList.add(s.toUpperCase());
}
}
After:
Today, the same operation can be more concisely and clearly performed using Streams:
List<String> filteredList = myList.stream()
.filter(s -> s.startsWith("J"))
.map(String::toUpperCase)
.collect(Collectors.toList());
Why This Matters: The Stream API, introduced in Java 8, promotes a more functional programming style. This makes our code more readable and often more efficient, especially when working with large collections or performing complex transformations.
Moving From Null Checks to Optional
Before:
Null checks were a necessary evil to prevent the dreaded NullPointerException
.
if (person != null && person.getAddress() != null) {
String city = person.getAddress().getCity();
// Proceed with city
}
After:
Java 8 introduced Optional
, a container object which may or may not contain a non-null value. This encourages a cleaner, more expressive approach to handling values that may be absent:
Optional.ofNullable(person)
.map(Person::getAddress)
.map(Address::getCity)
.ifPresent(city -> {
// Proceed with city
});
Why This Matters: Optional
helps in explicitly handling cases where a value may be missing. This leads to safer, more readable code that reduces the risk of NullPointerExceptions
.
Updating Date and Time Manipulation
Before:
Earlier code often used Date
and Calendar
classes for date and time which were not thread-safe and lacked flexibility.
Calendar calendar = Calendar.getInstance();
calendar.set(2015, Calendar.JANUARY, 1);
Date janFirst2015 = calendar.getTime();
After:
Java 8 introduced the java.time
package, providing a comprehensive model for date and time manipulation.
LocalDate janFirst2015 = LocalDate.of(2015, Month.JANUARY, 1);
Why This Matters: The java.time
API is immutable and thread-safe, making it a superior choice for date and time operations. It provides a more fluent and intuitive approach to handling time data.
Switching to JUnit 5
Before:
JUnit 4 was the go-to framework for testing Java applications.
import org.junit.Test;
import static org.junit.Assert.*;
public class SampleTest {
@Test
public void testSample() {
assertEquals(1, 1);
}
}
After:
JUnit 5, or Jupiter, is now the preferred testing framework, with more powerful features and more flexible test writing.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class SampleTest {
@Test
void testSample() {
assertEquals(1, 1);
}
}
Why This Matters: JUnit 5 supports Java 8 and above features, offers better extension models, and introduces more expressive asserting methods, making tests easier to write and understand. Upgrade your testing framework to leverage these benefits.
Additional Modernization Tips
- Leverage Var: Java 10 introduced the
var
keyword, enabling you to declare local variables with inferred types, making your code cleaner and reducing boilerplate. - Embrace Records: From Java 14 onwards, records provide a compact syntax for declaring classes that are transparent holders for shallowly immutable data. Use them for data transfer objects (DTOs) to reduce verbosity.
Resources for Further Learning
- For a deeper dive into the Stream API and functional programming in Java, consider exploring Oracle's official documentation.
- To master the new Date and Time API, read through the comprehensive guide available at Baeldung.
Updating your Java code is not merely about keeping up with the latest trends. It's about embracing practices that enhance code quality, readability, and maintainability. By moving away from obsolete 2015 trends and adopting modern Java features, you position your projects to capitalize on the advancements Java has made over the years. Remember, the goal is to write code that not only works well today but is also resilient, secure, and easy to update in the future.
Checkout our other articles