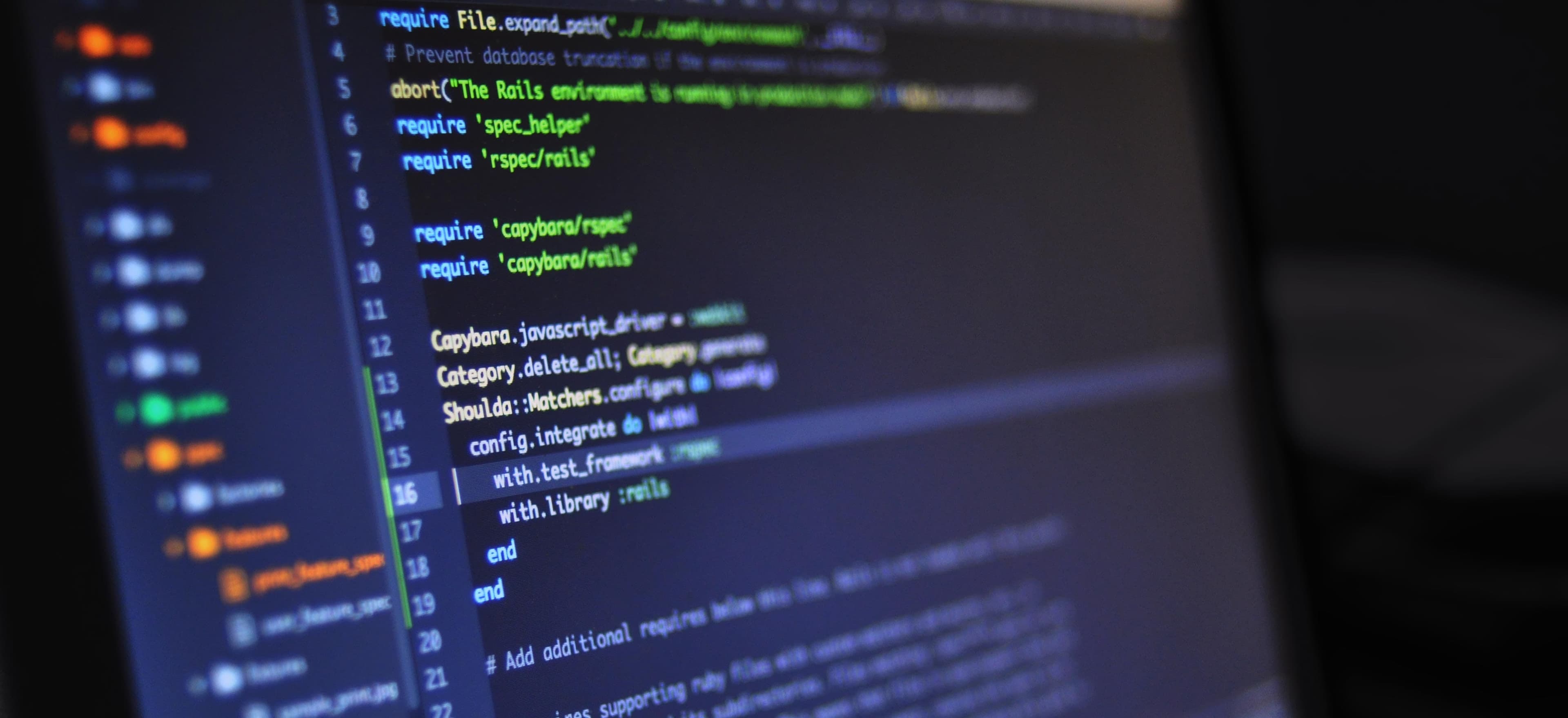
- Published on
Guard Your Secrets: Top Strategy to Protect Sensitive Data
In the world of software development, protecting sensitive data is a critical aspect of building secure applications. With the increasing number of cyber threats, maintaining the confidentiality and integrity of data has become more challenging than ever.
One of the most effective strategies to protect sensitive data in Java applications is to use the Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE) APIs. These APIs provide a framework for encryption, key generation, and key management, allowing developers to implement strong cryptographic algorithms to safeguard sensitive information.
Understanding the Java Cryptography Architecture (JCA)
The Java Cryptography Architecture (JCA) serves as the foundation for the Java platform's security framework. It provides a set of APIs for secure generation of cryptographic keys, encryption and decryption of data, secure random number generation, and digital signatures. The JCA architecture allows developers to integrate cryptographic functionality into their applications in a consistent and secure manner.
Leveraging Java Cryptography Extension (JCE) for Strong Encryption
The Java Cryptography Extension (JCE) is an integral part of the JCA and provides additional cryptographic services, including more robust algorithms for encryption, key generation, and message authentication. By leveraging JCE, developers can implement advanced encryption techniques such as AES (Advanced Encryption Standard) and RSA (Rivest-Shamir-Adleman) to protect sensitive data from unauthorized access.
Example: Encrypting Data using JCE
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.NoSuchAlgorithmException;
public class DataEncryptor {
public byte[] encryptData(String data) throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(256);
SecretKey secretKey = keyGenerator.generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data.getBytes());
}
}
In this example, we use the JCE APIs to generate an AES key, initialize a cipher for encryption, and then encrypt the data using the generated key.
Secure Key Management with Java Keystore
In addition to encryption, secure key management is essential for protecting sensitive data. The Java Keystore is a repository of security certificates, including public keys and private keys. It provides a reliable storage mechanism for cryptographic keys and certificates, allowing developers to securely manage their keys for encryption and authentication purposes.
Example: Storing Keys in Java Keystore
import java.io.FileInputStream;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.cert.CertificateException;
public class KeyStoreManager {
public void storeKey(String alias, SecretKey key, char[] password) throws KeyStoreException, IOException, NoSuchAlgorithmException, CertificateException {
KeyStore keyStore = KeyStore.getInstance("JCEKS");
keyStore.load(null, password);
KeyStore.SecretKeyEntry keyEntry = new KeyStore.SecretKeyEntry(key);
keyStore.setEntry(alias, keyEntry, new KeyStore.PasswordProtection(password));
keyStore.store(new FileOutputStream("keystore.jceks"), password);
}
}
In this example, we use the Java Keystore to store a secret key with a specified alias, protecting it with a password. The keystore is then stored in a secure file, ensuring the confidentiality of the stored key.
Best Practices for Protecting Sensitive Data
While leveraging the JCA, JCE, and Java Keystore provides a robust foundation for protecting sensitive data, it's essential to follow best practices to ensure the overall security of the application.
1. Use Strong Encryption Algorithms
Always use strong encryption algorithms, such as AES or RSA, to encrypt sensitive data. Avoid using weak algorithms that can be easily compromised by attackers.
2. Secure Key Generation
Utilize secure random number generators and strong key generation techniques to create cryptographic keys. Weak keys can undermine the security of the encryption process.
3. Secure Key Storage
Store cryptographic keys and certificates in secure storage mechanisms such as Java Keystore. Avoid hardcoding or embedding keys in the application code.
4. Key Rotation
Regularly rotate cryptographic keys to mitigate the impact of potential key compromises. Implementing key rotation strategies is essential for long-term security.
5. Secure Transmission
Ensure that sensitive data is transmitted over secure channels using protocols like HTTPS to prevent interception and eavesdropping.
My Closing Thoughts on the Matter
Protecting sensitive data in Java applications is paramount in maintaining the trust of users and safeguarding critical information from malicious actors. By leveraging the Java Cryptography Architecture, Java Cryptography Extension, and secure key management practices, developers can build robust and secure applications that prioritize data confidentiality and integrity. Adhering to best practices for encryption, key management, and transmission further strengthens the overall security posture of the application, creating a resilient defense against potential security threats.
Checkout our other articles