Boost Java Selenium Tests: Modify HTTP Headers Like a Pro!
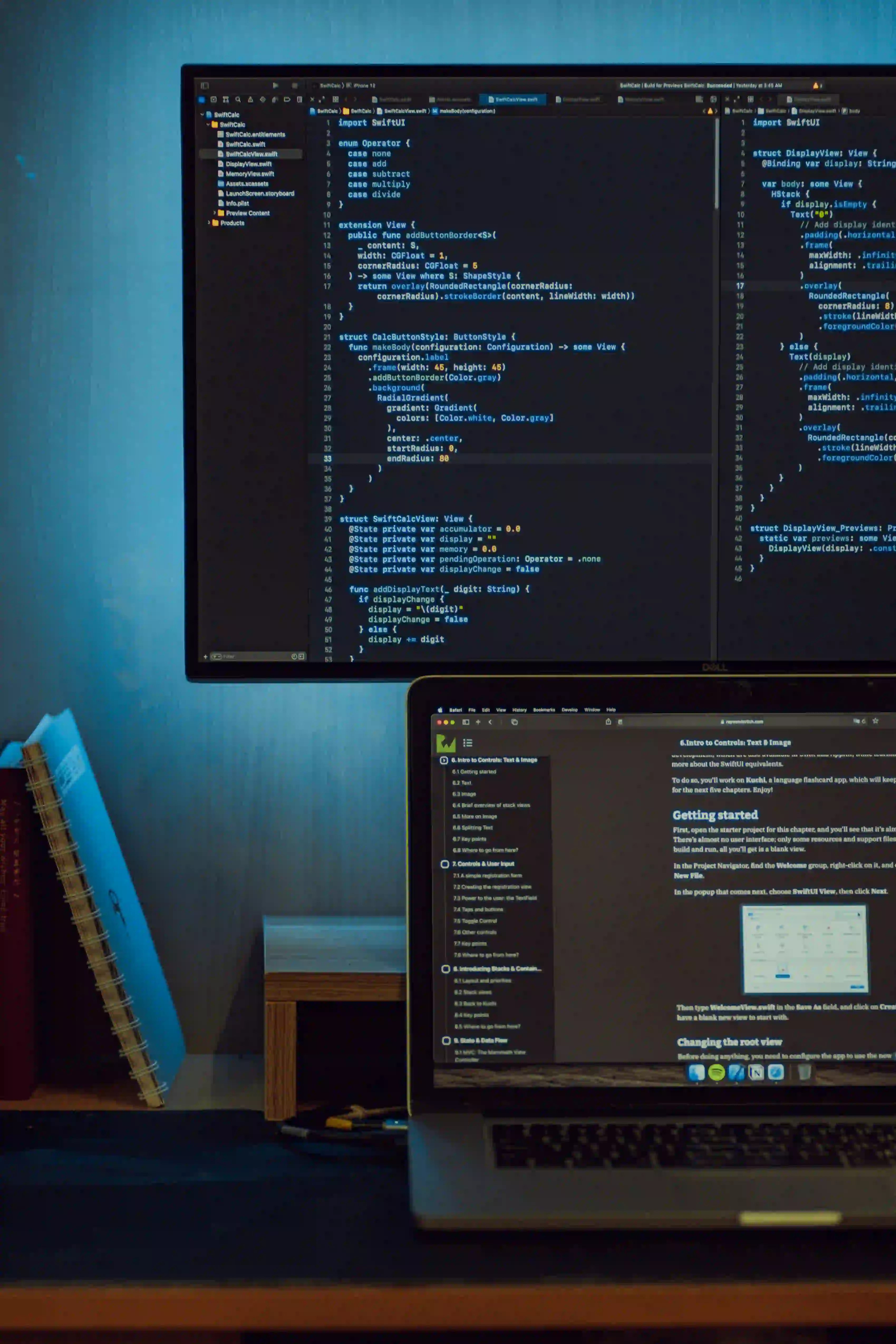
Boost Java Selenium Tests: Modify HTTP Headers Like a Pro!
As a Java developer working with Selenium for automated testing, you understand the importance of interacting with websites in a realistic manner. One way to enhance the realism of your tests is by modifying HTTP headers. In this blog post, we will explore how to achieve this in Java Selenium tests, providing you with the tools and knowledge to take your testing to the next level.
Why Modify HTTP Headers in Selenium Tests?
When a web page is loaded, the browser sends an HTTP request to the server, including headers such as User-Agent, Accept-Language, and more. By modifying these headers, you can simulate different user scenarios, test how your application behaves under varying conditions, and validate its robustness and flexibility.
For example, you might want to test how your web application handles requests from different types of browsers or devices, or how it responds to specific language settings. By modifying HTTP headers, you can mimic these scenarios, ensuring that your application functions correctly for a diverse range of users.
Getting Started with Modifying HTTP Headers in Java Selenium
To begin modifying HTTP headers in your Java Selenium tests, you'll need to add a dependency for a tool that allows you to intercept and modify network traffic. One popular tool for this is the BrowserMob Proxy. Let's go through the steps to set up BrowserMob Proxy in your project.
Step 1: Add BrowserMob Proxy Dependency
Add the following dependency to your Maven project's pom.xml
file:
<dependency>
<groupId>net.lightbody.bmp</groupId>
<artifactId>browsermob-core</artifactId>
<version>${browsermob-proxy-version}</version>
</dependency>
Replace ${browsermob-proxy-version}
with the latest version of BrowserMob Proxy.
Step 2: Create a BrowserMob Proxy Server
Now, let's create a method to start the BrowserMob Proxy server and modify the HTTP headers. Here's an example of setting up BrowserMob Proxy in your Java Selenium test:
import net.lightbody.bmp.BrowserMobProxy;
import net.lightbody.bmp.BrowserMobProxyServer;
import net.lightbody.bmp.proxy.CaptureType;
public class HeaderModifier {
public static void modifyHttpHeaders() {
// Start the BrowserMob Proxy server
BrowserMobProxy proxy = new BrowserMobProxyServer();
proxy.start(0);
// Enable capturing of request headers
proxy.enableHarCaptureTypes(CaptureType.REQUEST_HEADERS);
// Perform your Selenium actions with the modified headers
// This is where you would initialize your WebDriver and navigate to a web page
}
}
In this code, we initialize the BrowserMob Proxy server, enable capturing of request headers, and perform Selenium actions with the modified headers. We'll now explore how to modify the HTTP headers within the Selenium test.
Step 3: Modify HTTP Headers
After setting up the BrowserMob Proxy server, you can modify the HTTP headers as needed. Here's how you can modify the User-Agent header:
import net.lightbody.bmp.client.ClientUtil;
import org.openqa.selenium.Proxy;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class HeaderModifier {
public static void modifyHttpHeaders() {
// Start the BrowserMob Proxy server
BrowserMobProxy proxy = new BrowserMobProxyServer();
proxy.start(0);
// Enable capturing of request headers
proxy.enableHarCaptureTypes(CaptureType.REQUEST_HEADERS);
// Modify the User-Agent header
String userAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36";
proxy.addRequestFilter((request, contents, messageInfo) -> {
request.headers().add("User-Agent", userAgent);
return null;
});
// Perform your Selenium actions with the modified headers
}
}
In this snippet, we modify the User-Agent header by setting a custom user agent string. This can be useful for testing how your web application responds to different browser types and versions.
Applying Modified HTTP Headers in Your Tests
Now that you have set up BrowserMob Proxy and learned how to modify HTTP headers, you can integrate this approach into your Selenium tests. Let's demonstrate how to apply the modified headers to a Selenium WebDriver instance.
public class ModifiedHeaderSeleniumTest {
public static void main(String[] args) {
// Modify HTTP headers
HeaderModifier.modifyHttpHeaders();
// Set up Chrome options with proxy
Proxy seleniumProxy = ClientUtil.createSeleniumProxy(proxy);
ChromeOptions options = new ChromeOptions();
options.setCapability(CapabilityType.PROXY, seleniumProxy);
// Initialize Selenium WebDriver with modified headers
WebDriver driver = new ChromeDriver(options);
driver.get("https://www.example.com");
// Rest of your Selenium test code
}
}
In this example, we modify the HTTP headers using BrowserMob Proxy and integrate them into a Chrome WebDriver instance. This allows you to test your web application with the modified headers, enabling comprehensive testing of various scenarios.
Key Takeaways
By modifying HTTP headers in your Java Selenium tests, you can create more realistic and comprehensive test scenarios, leading to improved test coverage and robustness. With the BrowserMob Proxy tool and the techniques described in this post, you have the capability to take your Selenium testing to a professional level, ensuring that your web application functions seamlessly for a diverse user base.
We've covered the basics of modifying HTTP headers in Java Selenium tests, from setting up BrowserMob Proxy to integrating modified headers into your test scenarios. Incorporating this approach into your testing practices will elevate the quality and effectiveness of your Selenium tests, paving the way for more reliable and resilient web applications.
Start leveraging the power of modified HTTP headers in your Java Selenium tests today and elevate your testing game like a pro!
Additional Resources
Remember, the key to successful testing is in the details, and modifying HTTP headers in your Java Selenium tests equips you with the precision and control needed to achieve comprehensive test coverage. Embrace this advanced technique and empower your testing endeavors with unparalleled realism and accuracy.