Java 9 Delayed: Navigating the Jigsaw Module Puzzle
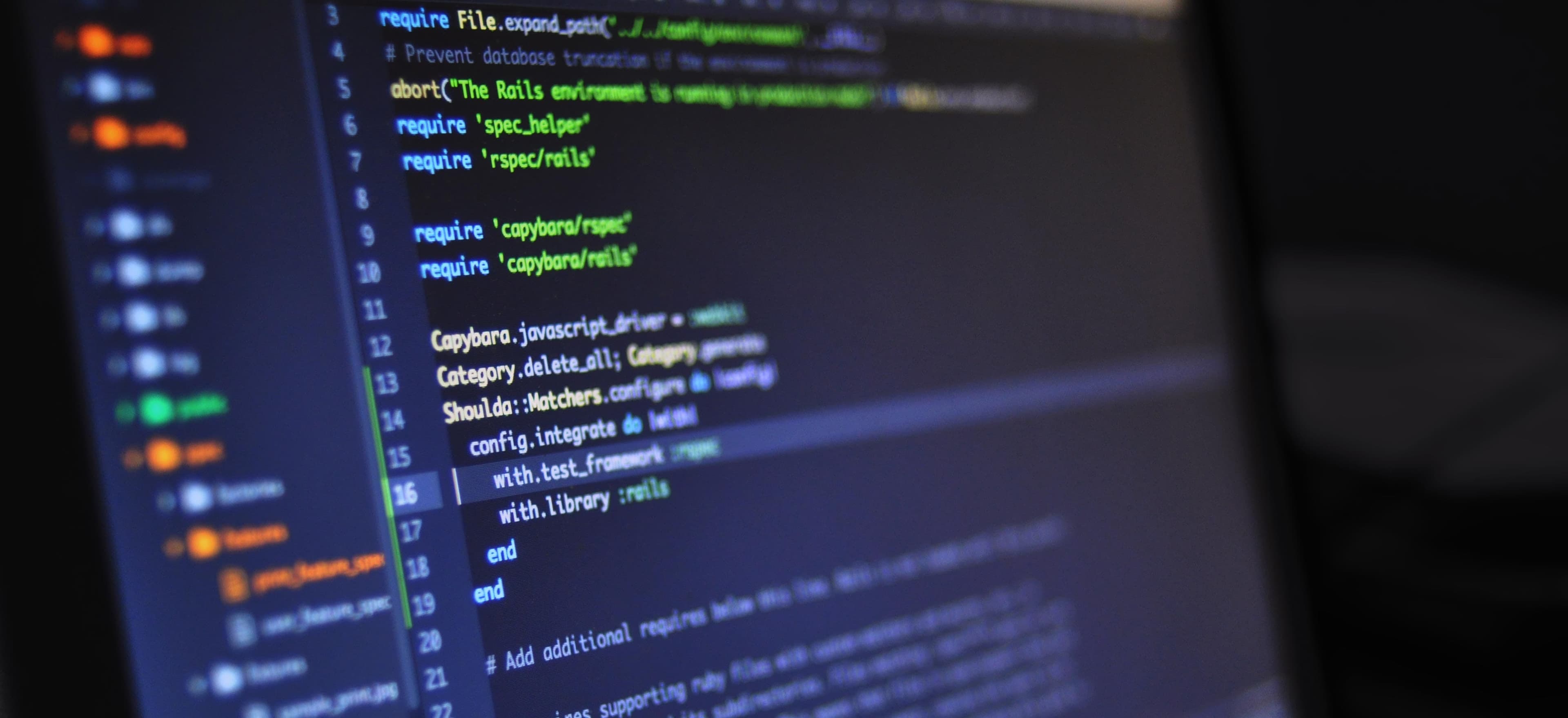
- Published on
Navigating the Jigsaw Module Puzzle in Java 9
Java 9 brought significant changes to the way developers work with the platform, and one of the most prominent additions is the modular system, Project Jigsaw. This system introduces the concept of modules to Java, allowing developers to encapsulate code and specify dependencies. In this article, we will explore the Java 9 module system, its benefits, and how to navigate the Jigsaw module puzzle.
Understanding Java 9 Modules
In Java 9, a module is a collection of code and data that encapsulates a set of related functionalities. This encapsulation provides better maintainability, reusability, and modularity for large-scale applications. To define a module, we use the module-info.java
file, which specifies the module name, dependencies, and exported packages.
module com.example.myapp {
requires java.base;
exports com.example.myapp.util;
}
In this example, the com.example.myapp
module requires the java.base
module and exports the com.example.myapp.util
package for use by other modules.
Benefits of Module System
The module system offers several benefits, including:
- Strong Encapsulation: Modules encapsulate their implementation details, preventing unauthorized access and reducing inter-module dependencies.
- Explicit Dependencies: Modules explicitly declare their dependencies, making the system more maintainable and robust.
- Improved Performance: The module system enables more efficient packaging, deployment, and runtime optimizations.
- Simplified Configuration: The module system simplifies the classpath and reduces classpath-related issues.
Navigating the Module Path
In Java 9, the classpath is augmented with the module path, which is used to locate modules. The module path contains modular JAR files or directories containing modules. To compile and run modular code, we use the --module-path
option to specify the module path.
Compiling Modular Code
To compile the modular code, we use the javac
command with the --module-path
option followed by the path to the module. For example:
javac --module-path mymodules/ -d mods/ src/com.example.myapp/module-info.java src/com.example.myapp/util/Util.java
This command compiles the com.example.myapp
module and places the output in the mods
directory.
Running Modular Applications
When running modular applications, we use the java
command with the --module-path
option and the --module
option to specify the main module. For example:
java --module-path mods/ --module com.example.myapp/com.example.myapp.Main
Here, we run the Main
class from the com.example.myapp
module.
Resolving Module Dependencies
In the module descriptor, the requires
directive specifies the modules required by the current module. During compilation and runtime, the Java platform resolves these dependencies to ensure that the required modules are available.
Automatic Module Names
In scenarios where a library is not a module, Java 9 introduces automatic module names. When a JAR file is placed on the module path, but it does not contain a module descriptor, Java 9 derives a module name from the JAR file's name. This automatic module name allows non-modular JARs to be used in the module system.
Modularizing Existing Libraries
With Java 9's module system, existing libraries or JAR files can be modularized using the jar
tool with the --create
option. Using this approach, developers can transform libraries into modules, enabling them to take advantage of the enhanced modularity features provided by Java 9.
Final Considerations
The module system in Java 9 introduces a new way to organize and structure Java applications, offering numerous benefits for developers and maintainers of Java codebases. By understanding the basics of modules, leveraging the module path, resolving dependencies, and modularizing existing libraries, developers can successfully navigate the Jigsaw module puzzle and harness the power of modularity in Java 9.
For further information, refer to the official Java 9 documentation on Modules.
Stay modular!
Checkout our other articles