Boost Your Metrics with Spring Boot & InfluxDB Integration
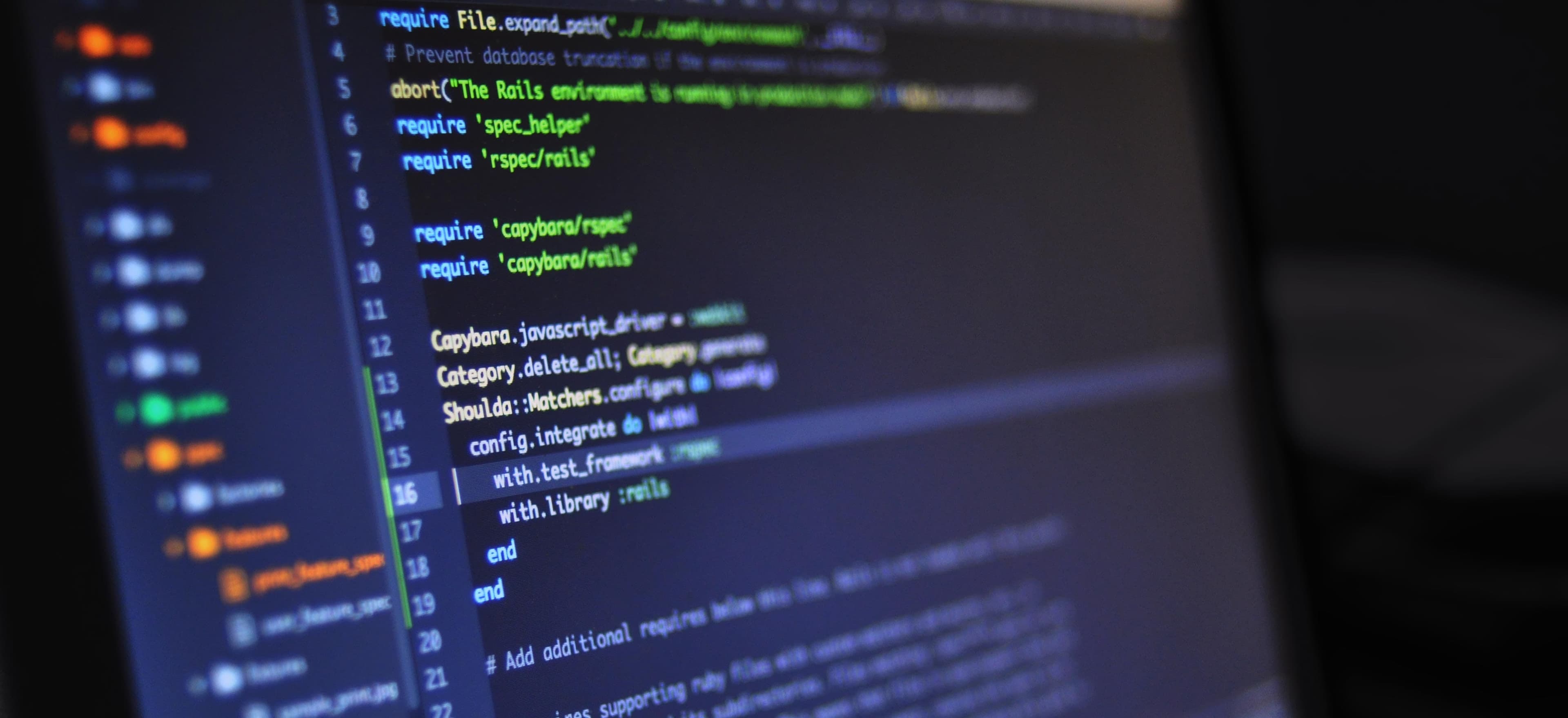
- Published on
Boost Your Metrics with Spring Boot & InfluxDB Integration
Are you managing a Spring Boot application and aiming for better performance metrics? If yes, you've probably considered different storage solutions for your app's data. Today, we're diving deep into the world of metrics storage and retrieval is using InfluxDB, specifically tailored for Spring Boot applications. By integrating InfluxDB, you're not only choosing an optimal storage solution but also setting the stage for insightful data visualization and analysis.
Why InfluxDB with Spring Boot?
First things first, let's discuss why integrating InfluxDB into your Spring Boot application is a stellar idea. InfluxDB is a time series database specifically designed to handle high write and query loads, which is perfect for storing and analyzing time-stamped data. Given that Spring Boot is a popular framework for building microservices and web applications, combining it with InfluxDB presents a powerful duo for managing real-time analytics, monitoring, and event logging.
The Integration Magic
The magic behind integrating Spring Boot with InfluxDB lies in the simplicity and efficiency it brings to your project. InfluxDB provides a Spring Data InfluxDB starter that simplifies the process, making the integration smoother and hassle-free.
Setting the Scene
Before we dive into the implementation, make sure you have the following prerequisites in your environment:
- JDK 11 or newer
- Maven or Gradle
- Spring Boot 2.3.0.RELEASE or newer
- An InfluxDB instance (You can use a local instance, or set up a free account on InfluxDB Cloud at InfluxDB Cloud)
Step-by-Step Integration
Step 1: Add Spring Data InfluxDB Dependency
First, add the Spring Data InfluxDB starter to your project's pom.xml
file if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-influxdb</artifactId>
<version>YOUR_SPRING_BOOT_VERSION</version>
</dependency>
Or to your build.gradle
file if you are using Gradle:
implementation 'org.springframework.boot:spring-boot-starter-data-influxdb:YOUR_SPRING_BOOT_VERSION'
Replace YOUR_SPRING_BOOT_VERSION
with the version of Spring Boot you are using.
Step 2: Configuration
Next, you'll need to configure the connection to your InfluxDB instance. This is done by adding properties to your application.properties
or application.yml
file. Here's an example for application.properties
:
spring.influx.url=http://localhost:8086
spring.influx.database=mydatabase
spring.influx.user=myuser
spring.influx.password=mypassword
spring.influx.retention-policy=autogen
Make sure to replace the values with those corresponding to your InfluxDB setup.
Step 3: Writing and Reading Data
With InfluxDB integrated and configured in your Spring Boot app, it's time to start writing and reading data. Let's look at a simple example of how to do this.
Writing Data
You can define a Spring service that uses InfluxDB's InfluxDBTemplate
to write data points to your database:
@Service
public class MetricService {
@Autowired
private InfluxDBTemplate<Point> influxDBTemplate;
public void writeData() {
Point point = Point.measurement("memory")
.time(System.currentTimeMillis(), TimeUnit.MILLISECONDS)
.addField("used", 1024)
.build();
influxDBTemplate.write(point);
}
}
In this snippet, we're creating a data point for a measurement called 'memory', with a single field 'used' representing used memory. This is a simplistic example; your real-world usage might involve more complex data structures.
Reading Data
Reading data from InfluxDB can be achieved using the InfluxDBTemplate
as well. Here's a simple select query:
public List<Point> readData() {
String query = "SELECT * FROM memory";
QueryResult queryResult = influxDBTemplate.query(new Query(query, database));
// Process queryResult to extract data points
}
In this example, after executing the query, you would need to process queryResult
to extract the data points and transform them into a more usable format, typically involving some mapping to domain-specific classes or structures.
Further Work and Optimization
After getting the basics right, consider looking into more advanced features and optimizations like:
- Batching Writes - InfluxDB supports batched writes for improved performance. Check out their documentation on batch processing for more details.
- Custom Retention Policies - Managing data lifecycle in InfluxDB by defining custom retention policies suited to the nature of your data.
- High-Availability Setup - If your application demands high availability, explore setting up InfluxDB in a clustered environment.
Bringing It All Together
Integrating Spring Boot with InfluxDB opens a gateway to efficiently handling time-series data, which is invaluable for metrics, monitoring, and analytics in modern applications. This integration not only leverages the strengths of both Spring Boot's and InfluxDB's ecosystems but also empowers developers to build robust, scalable applications with a solid data storage and analytics backbone.
For a deeper dive into InfluxDB's features and more complex scenarios, visit the official InfluxDB documentation.
Remember, the core of integrating any technology is understanding not just the 'how' but the 'why' - and with Spring Boot and InfluxDB, the 'why' is clear: performance, scalability, and insightful data analysis.
Good luck on your journey to creating more performant and insightful Spring Boot applications with InfluxDB!
Checkout our other articles