Solving Maven & Tomcat Deployment Woes for Spring-Angular Apps
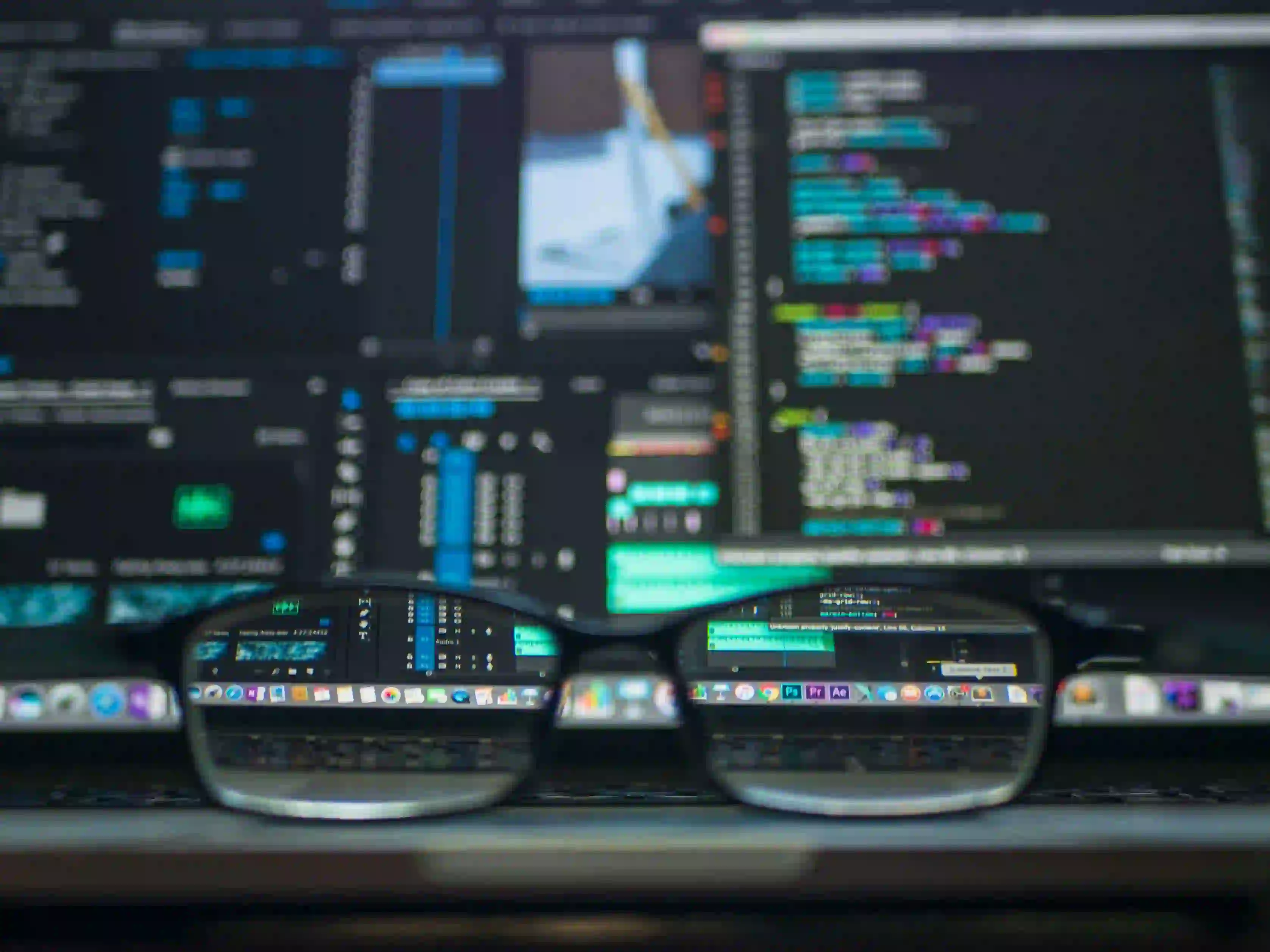
Solving Maven & Tomcat Deployment Woes for Spring-Angular Apps
Let us Begin
Deploying Spring-Angular applications using Maven and Tomcat can be both an exciting and daunting task for developers. While Maven simplifies the build process and dependency management, Tomcat serves as a capable container for running Java web applications. As for Spring and Angular, their combination offers a robust platform for creating modern, full-stack applications.
What is Maven?
Maven is a powerful build automation tool that manages project dependencies and facilitates the build process. It uses a Project Object Model (POM) file to define project structure, dependencies, and build configurations.
What is Tomcat?
Apache Tomcat, often referred to as Tomcat, is an open-source implementation of the Java Servlet, JavaServer Pages, Java Expression Language, and Java WebSocket technologies. It serves as a web server and Java servlet container, providing a platform for deploying Java-based web applications.
Why Spring and Angular?
Spring is a popular Java framework known for its dependency injection, inversion of control, and powerful support for enterprise-level features. Angular, on the other hand, is a widely used front-end framework for building dynamic web applications. The combination of Spring and Angular allows for seamless integration between the backend and frontend, enabling the creation of sophisticated, responsive web applications.
The Challenge of Deployment
The deployment of Spring-Angular applications using Maven and Tomcat presents several challenges, including issues with Maven builds, errors during Tomcat deployment, and potential integration problems that may arise between Spring and Angular.
Developers often encounter compatibility problems between different versions of the libraries, incorrect configurations in the deployment descriptors, and runtime issues related to cross-origin resource sharing (CORS). These issues can lead to frustration and inefficiencies during the deployment process, affecting the overall development lifecycle.
Prerequisites
Before diving into the deployment process, ensure that the following prerequisites are met:
- Java Development Kit (JDK) 8 or higher installed
- Apache Maven 3.3.9 or later installed
- Apache Tomcat 8.5.x or later installed
- Basic knowledge of Spring and Angular setup
Now that we've outlined the challenges and prerequisites, let's delve into the deployment process and explore how to overcome these obstacles effectively.
Step 1: Setting Up Your Maven Project for Spring and Angular
To start, let's configure a Maven project that integrates both Spring and Angular. We'll define the project structure and required dependencies in the pom.xml file to seamlessly manage the backend and frontend components.
Directory Structure
In a Maven project, the directory structure should reflect the separation between the Spring backend and Angular frontend. Below is an example structure:
project-root
ā
āāā backend
ā āāā src
ā ā āāā main
ā ā ā āāā java
ā ā ā āāā resources
ā ā āāā test
āāā frontend
āāā src
ā āāā app
ā āāā assets
āāā angular.json
pom.xml Configurations
The pom.xml file is the heart of the Maven build process. Let's look at a simplified version with key dependencies and plugins:
<project>
<!-- Other configurations -->
<dependencies>
<!-- Dependencies for Spring backend -->
</dependencies>
<build>
<plugins>
<!-- Maven plugins for building and packaging -->
</plugins>
</build>
</project>
Commentary
In the pom.xml file, we define dependencies for the Spring backend, such as Spring Boot, Hibernate, and other necessary libraries. Additionally, we configure Maven plugins to handle the build, test, and packaging processes. These configurations lay the foundation for a well-structured Maven project that integrates Spring and Angular.
Step 2: Integrating Angular Build with Maven
Integrating the Angular build process into Maven ensures a seamless end-to-end deployment workflow. The frontend-maven-plugin simplifies this integration by automating the build process for the Angular application within the Maven lifecycle.
Using frontend-maven-plugin
The frontend-maven-plugin allows for the execution of Node.js and npm commands during the Maven build. It automates the installation of frontend dependencies and the execution of build scripts, such as ng build
.
<build>
<plugins>
<plugin>
<groupId>com.github.eirslett</groupId>
<artifactId>frontend-maven-plugin</artifactId>
<version>1.13.1</version>
<configuration>
<workingDirectory>frontend</workingDirectory>
</configuration>
<executions>
<execution>
<id>install node and npm</id>
<goals>
<goal>install-node-and-npm</goal>
</goals>
</execution>
<execution>
<id>npm install</id>
<goals>
<goal>npm</goal>
<configuration>
<arguments>install</arguments>
</configuration>
</goals>
</execution>
<execution>
<id>npm build</id>
<goals>
<goal>npm</goal>
<configuration>
<arguments>run build --prod</arguments>
</configuration>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Commentary
The <configuration>
tag specifies the working directory for the frontend-maven-plugin. The <executions>
section defines multiple goals for installing Node.js, running npm install
, and executing the production build using ng build --prod
. By incorporating these configurations into the pom.xml, we streamline the frontend build process as part of the overall Maven build.
Step 3: Configuring Tomcat for Deployment
With the Maven setup in place, it's time to focus on configuring Tomcat for deploying the Spring-Angular application. Proper configurations regarding server settings, context paths, and deployment strategies are essential for a successful deployment.
Setting up Tomcat server.xml
In the server.xml file of Tomcat, specific configurations can optimize the server for running the Spring-Angular application. Fine-tuning attributes related to connectors, thread pools, and other settings can enhance performance and scalability.
Configuring Context Paths
Correctly defining context paths in both the Tomcat server and the application is crucial for routing requests effectively. It ensures that the application is accessible via the desired URL and maintains a clear separation between different deployed applications on the same server.
Deploying WAR files vs Exploded Directories
While deploying a WAR file (Web Application Archive) offers portability and encapsulation, using exploded directories provides flexibility during development and debugging. Understanding the pros and cons of each approach helps in making an informed deployment choice.
Step 4: Troubleshooting Common Deployment Issues
During the deployment process, several common issues might arise, such as CORS conflicts, Maven build errors, and Tomcat deployment failures. Let's address each of these and provide actionable solutions.
Dealing with CORS Issues
Cross-Origin Resource Sharing (CORS) issues can hinder communication between the Spring backend and Angular frontend, leading to blocked requests. One way to mitigate this is by configuring CORS handling in the Spring application, allowing for secure cross-origin requests.
Resolving Maven Build Errors
Maven build errors can stem from incorrect configurations, missing dependencies, or compatibility issues. Thoroughly analyzing build logs and validating project configurations can aid in identifying and resolving these errors.
Fixing Tomcat Deployment Errors
Tomcat deployment errors may arise due to misconfigured context paths, conflicting dependencies, or server configuration issues. Regularly monitoring Tomcat logs and debugging deployment-related errors can help in diagnosing and addressing deployment failures.
Wrapping Up
Deploying Spring-Angular applications using Maven and Tomcat encompasses a myriad of configurations and integrations across the backend, frontend, and server environment. By embracing best practices and addressing common deployment challenges, developers can streamline the deployment process and ensure a robust, stable environment for their applications.
Additional Resources
For further reading and exploration, refer to the following resources:
- Official Maven Documentation
- Tomcat's Official Documentation
- Spring Framework Documentation
- Angular Official Guide
In summary, mastering the deployment of Spring-Angular applications with Maven and Tomcat not only enhances development efficiency but also lays a strong foundation for delivering high-quality, modern web applications.
In conclusion, the deployment of Spring-Angular applications using Maven and Tomcat can present significant challenges, but with the right tools and configurations, these challenges can be overcome. By following the steps outlined in this article and understanding the common pitfalls, developers can ensure smooth and efficient deployment of their applications.