Mastering Spring: Solving JSON IdentityInfo Hurdles
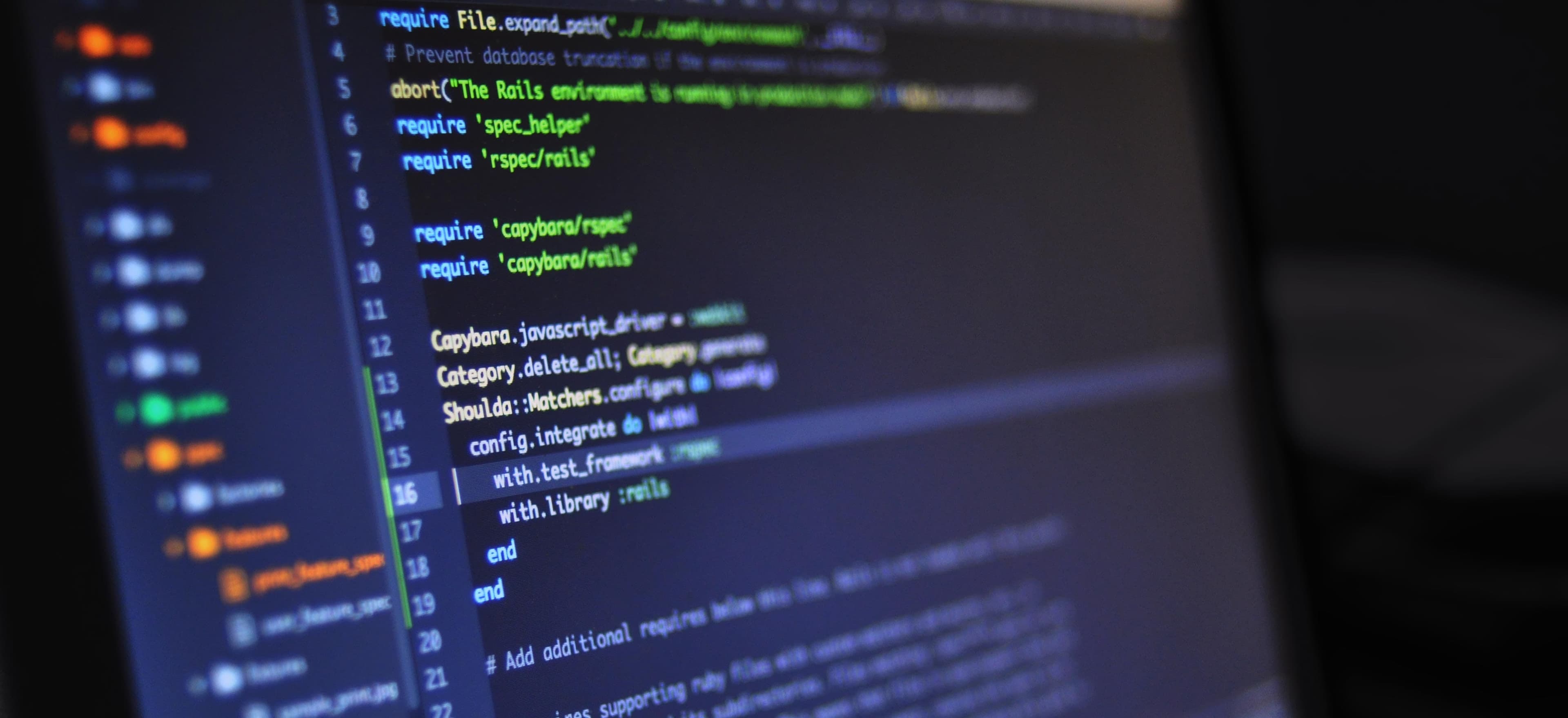
- Published on
Mastering Spring: Solving JSON IdentityInfo Hurdles
As a Java developer working with Spring, you’ve likely encountered the challenge of handling JSON serialization and deserialization. One common issue is dealing with circular references when serializing entities with bi-directional relationships. This often results in StackOverflowErrors or infinite loops. Fortunately, Spring provides a straightforward solution to this problem through the use of @JsonIdentityInfo
. In this blog post, we will delve into this annotation, explore its functionality, and demonstrate how it can be effectively used to overcome JSON identity-related hurdles in your Spring applications.
Understanding the Issue
Consider a classic scenario where you have two entities, such as Author
and Book
, with a bi-directional relationship. An author can have multiple books, and a book can have an author. When you attempt to serialize an author object that contains a list of books, and each book in turn references the author, you encounter a circular reference. This circular reference confuses the default JSON serialization process, leading to the errors mentioned earlier.
Introducing @JsonIdentityInfo
The @JsonIdentityInfo
annotation from the Jackson library provides a solution by instructing the JSON serializer to handle object identity. By applying this annotation to the entity classes involved in the circular reference, you can ensure that the serialization process maintains object identity by serializing the object only once, and then using references to that object for subsequent occurrences.
Let’s examine how to utilize @JsonIdentityInfo
in a practical Spring application.
Applying @JsonIdentityInfo
Consider the following Author
and Book
entity classes representing the bi-directional relationship:
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author")
@JsonManagedReference
private List<Book> books;
// Constructors, getters, and setters
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
@JsonBackReference
private Author author;
// Constructors, getters, and setters
}
In the Author
entity, we use @JsonManagedReference
to mark the books
field, indicating that it is the forward part of the reference. Similarly, in the Book
entity, we use @JsonBackReference
to mark the author
field, indicating that it is the back part of the reference.
To enable the use of @JsonIdentityInfo
, we need to add the annotation to the entity classes and specify the generator type, as shown below:
@Entity
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id")
public class Author {
// ...
}
@Entity
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id")
public class Book {
// ...
}
In the above code, we specify ObjectIdGenerators.PropertyGenerator.class
as the generator, and provide the property name using the property
attribute. This configuration ensures that object identities are handled correctly during serialization.
Practical Demonstration
Let’s demonstrate the effect of @JsonIdentityInfo
by serializing an Author
object that has a list of Book
objects.
Author author = new Author("John Doe");
Book book1 = new Book("Spring in Action", author);
Book book2 = new Book("Java Concurrency in Practice", author);
author.setBooks(Arrays.asList(book1, book2));
ObjectMapper objectMapper = new ObjectMapper();
String serializedAuthor = objectMapper.writeValueAsString(author);
System.out.println(serializedAuthor);
When you execute the above code, you will notice that the generated JSON string maintains object identity, using references to the Author
object for the books' author
property, instead of serializing the entire Author
object for each book.
Wrapping Up
In this blog post, we explored the common issue of circular references during JSON serialization in Spring applications and how the @JsonIdentityInfo
annotation can be utilized to overcome this challenge. By following the discussed approach and applying @JsonIdentityInfo
to entity classes with bi-directional relationships, you can ensure that the JSON serialization process handles object identities effectively, thereby preventing circular reference-related errors.
By mastering the usage of @JsonIdentityInfo
, you can enhance the robustness of your Spring applications and deliver efficient and reliable JSON serialization and deserialization. Incorporating this annotation into your development toolkit will undoubtedly empower you to tackle JSON identity-related hurdles with confidence.
In summary, @JsonIdentityInfo
is a valuable tool for Java developers working with Spring, offering a simple yet powerful solution to JSON identity challenges. Embracing this annotation will undoubtedly elevate your proficiency in managing JSON serialization complexities within Spring applications.
Now that you have a solid understanding of @JsonIdentityInfo
, consider exploring other advanced features and best practices for working with JSON in Spring to further enhance your expertise in this domain.
In the dynamic landscape of Java development, continual learning and exploration of advanced concepts are essential to stay ahead. Seize the opportunity to delve into Jackson's documentation, further solidifying your understanding of JSON processing in Spring.
Expand your knowledge by exploring various approaches to optimizing JSON handling in Spring, such as leveraging custom serializers and deserializers, and stay attuned to the evolving best practices within the Java ecosystem.
Level up your Java skills and elevate your Spring prowess by integrating advanced techniques for efficient and resilient JSON processing. Mastering Spring entails embracing the nuances of JSON handling, and with the adept utilization of @JsonIdentityInfo
, you have taken a significant stride towards achieving expertise in this crucial aspect of modern Java development.
Checkout our other articles