Tackling Slow Requests: Optimizing Android Volley
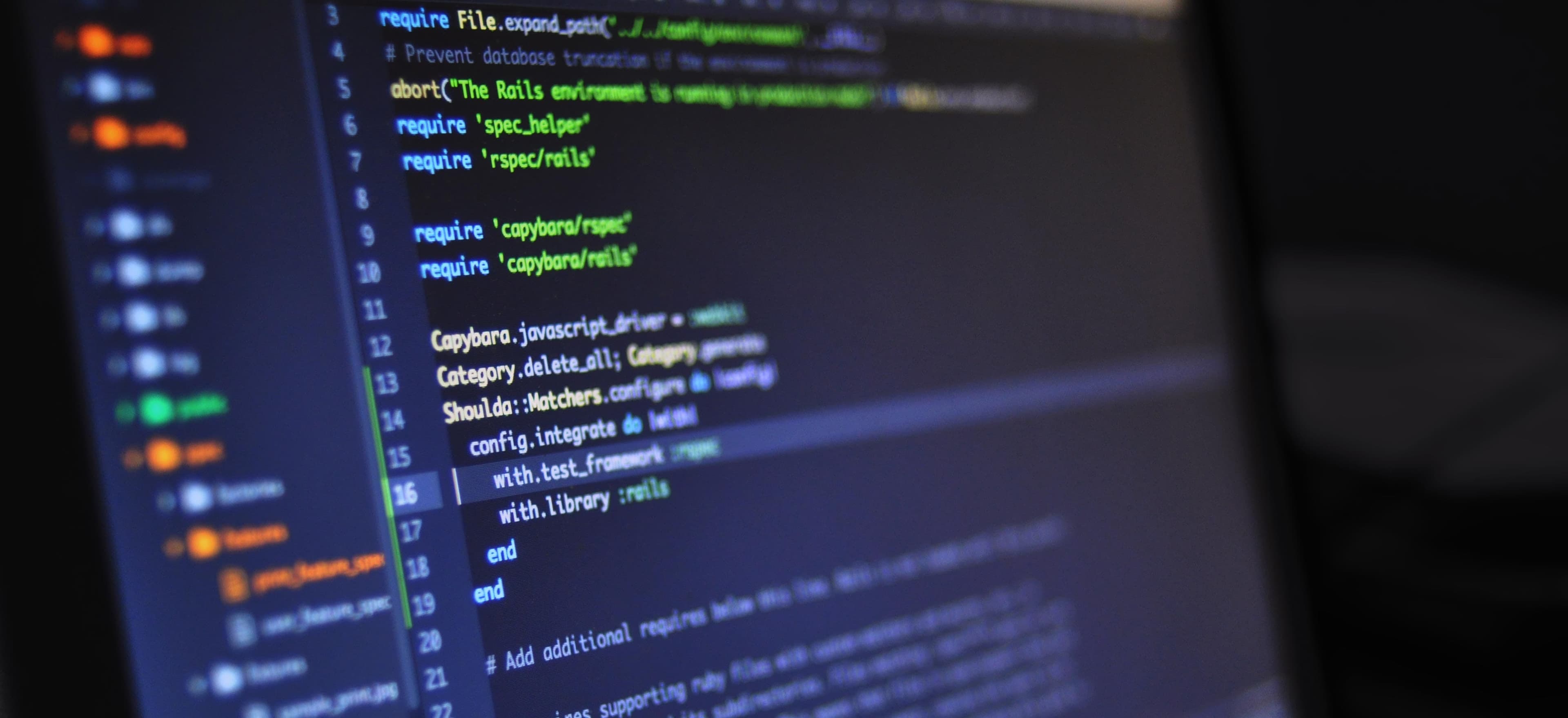
- Published on
Optimizing Android Volley for Faster Network Requests
In the world of Android development, efficient network requests are crucial for a seamless user experience. Poorly optimized network calls can lead to slow response times, increased battery consumption, and overall frustration for the end user. One popular library for handling network requests in Android is Volley – a powerful tool for managing HTTP requests. However, to ensure optimal performance, developers need to familiarize themselves with the techniques to optimize Volley for faster network requests.
Understanding the Problem
When it comes to slow network requests in Android using Volley, there could be several factors at play. It could be inefficient handling of the request queue, improper use of caching, or even overlooked aspects of response parsing. By pinpointing the specific areas that need optimization, developers can significantly enhance the responsiveness of their network calls.
Leveraging Request Prioritization
One of the ways to optimize network requests in Android Volley is by leveraging request prioritization. By default, Volley processes requests in a first-come, first-served basis. However, in scenarios where certain requests are more critical than others, developers can prioritize them accordingly.
RequestQueue requestQueue = Volley.newRequestQueue(context);
Request<T> request = new CustomRequest<>( /* request parameters */ );
// Set the priority for the request
request.setPriority(Request.Priority.IMMEDIATE);
requestQueue.add(request);
The above code snippet showcases how to prioritize a request as immediate. This can be useful for crucial operations such as login or real-time updates where responsiveness is paramount.
Efficient Cache Management
Another area where developers can optimize Android Volley is by implementing efficient cache management. Volley comes with built-in caching mechanisms, and understanding how to utilize them effectively can lead to significant performance improvements.
// Creating a custom request with caching enabled
RequestQueue requestQueue = Volley.newRequestQueue(context);
Request<T> request = new CustomRequest<>( /* request parameters */ );
// Enable caching for the request
request.setShouldCache(true);
requestQueue.add(request);
Enabling caching for requests that are suitable for it helps in reducing redundant network calls and speeds up the overall response time.
Throttling Retry Policies
Retry policies play a crucial role in handling network instability. However, excessive retries can exacerbate slow requests. By implementing a well-thought-out retry policy, developers can prevent unnecessary retries that contribute to delays in network responses.
// Setting a custom retry policy for the request
RequestQueue requestQueue = Volley.newRequestQueue(context);
Request<T> request = new CustomRequest<>( /* request parameters */ );
// Set a custom retry policy
request.setRetryPolicy(new DefaultRetryPolicy(
MY_SOCKET_TIMEOUT_MS,
DefaultRetryPolicy.DEFAULT_MAX_RETRIES,
DefaultRetryPolicy.DEFAULT_BACKOFF_MULT));
requestQueue.add(request);
In the code snippet above, a custom retry policy is set to handle socket timeouts, maximum retries, and backoff multiplier. These parameters can be fine-tuned based on the specific network requirements to prevent unnecessary retries that slow down the request.
Implementing Delivery Mechanism
Another important factor in optimizing Android Volley is the implementation of a proper delivery mechanism for handling responses. Utilizing a custom delivery mechanism allows developers to fine-tune the threading model for response delivery, which can have a significant impact on the overall responsiveness of network requests.
// Implementing a custom delivery mechanism
RequestQueue requestQueue = Volley.newRequestQueue(context);
Request<T> request = new CustomRequest<>( /* request parameters */ );
// Set a custom delivery mechanism
request.setDelivery(new ExecutorDelivery(new Handler(Looper.getMainLooper())));
requestQueue.add(request);
In the example above, a custom delivery mechanism is employed using an ExecutorDelivery
with a Handler
attached to the main looper. This ensures that responses are delivered on the main thread, which is crucial for UI updates and maintaining a responsive user interface.
Key Takeaways
Optimizing network requests in Android using Volley is essential for delivering a smooth and efficient user experience. By understanding the nuances of request prioritization, cache management, retry policies, and delivery mechanisms, developers can significantly enhance the performance of their network calls. It's important to remember that the effectiveness of these optimizations may vary based on the specific use case and network conditions. Experimenting with different approaches and measuring the impact on real-world scenarios is crucial for identifying the most effective optimization strategies.
By implementing these optimization techniques, developers can ensure that their Android apps deliver fast and responsive network requests, thereby improving overall user satisfaction and retention.
Remember, efficient networking in Android development is not only about writing good code but also about understanding the intricacies of the underlying network operations.
Checkout our other articles