Simplifying Code: Mastering Factory Method Design Pattern
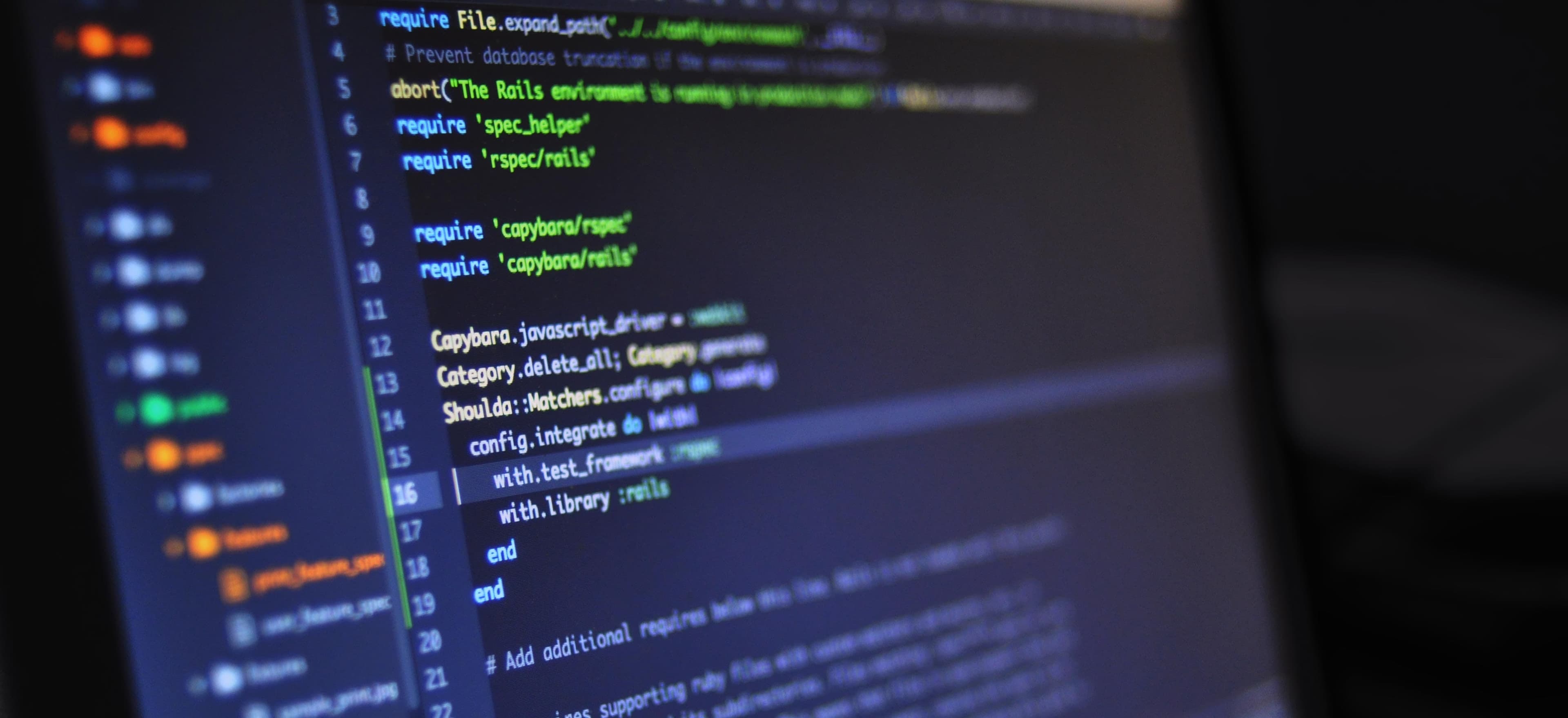
- Published on
Simplifying Code: Mastering Factory Method Design Pattern
In the world of Java programming, the Factory Method Design Pattern plays a crucial role in simplifying code and creating objects. It provides a way to delegate the instantiation logic to child classes, thus promoting loose coupling and enhancing the overall maintainability of the codebase. In this blog post, we will delve into the intricacies of the Factory Method pattern, understand its usage, and explore how it simplifies code in Java applications.
Understanding the Factory Method Design Pattern
The Factory Method pattern is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. This pattern encapsulates the creation of objects and decouples the client code from the actual implementation of the created objects.
Why Use the Factory Method Pattern?
The Factory Method pattern is particularly useful in scenarios where the client code needs to create objects without knowing the exact class or type of the object it will use. By delegating the object creation to subclasses, the pattern allows for easy extensibility and maintenance, enabling the addition of new subclasses without affecting the client code.
Implementing the Factory Method Pattern in Java
Let's dive into a practical example to understand the implementation of the Factory Method pattern in Java. Consider an application that involves creating different types of shapes, such as circles and rectangles. We can define a Shape
interface and concrete classes Circle
and Rectangle
that implement this interface. Next, we'll create a ShapeFactory
interface with a method for creating shapes.
Example Code:
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Inside Rectangle::draw() method.");
}
}
public interface ShapeFactory {
Shape createShape();
}
public class CircleFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Circle();
}
}
public class RectangleFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Rectangle();
}
}
In the above example, we define the Shape
interface and concrete implementations for Circle
and Rectangle
. We also create a ShapeFactory
interface with concrete implementations CircleFactory
and RectangleFactory
that produce instances of the respective shapes.
Why It Works:
The Factory Method pattern allows us to delegate the instantiation of Circle
and Rectangle
objects to their respective factories. This not only encapsulates the object creation logic but also allows us to easily add new shape types by creating additional concrete factory implementations without modifying the client code.
Advantages of Using the Factory Method Pattern
1. Encapsulating Object Creation Logic
By centralizing the object creation process within factory classes, the pattern encapsulates the object creation logic and keeps it separate from the client code. This promotes a cleaner, more maintainable codebase.
2. Reusability and Extensibility
The Factory Method pattern promotes reusability by allowing multiple client classes to use the same factory to create objects. Additionally, it facilitates easy extensibility by supporting the addition of new product types and corresponding factory implementations.
3. Decoupling the Client Code
Since the client code interacts with the factory interface rather than directly with the concrete product classes, the Factory Method pattern decouples the client from the implementation details of the created objects. This decoupling enhances flexibility and scalability.
My Closing Thoughts on the Matter
In the world of Java development, mastering design patterns such as the Factory Method pattern can significantly simplify code, enhance maintainability, and promote flexibility and extensibility. By seamlessly delegating object creation to subclasses, the Factory Method pattern encapsulates the instantiation logic, fosters loose coupling, and enables the addition of new subclasses without impacting the existing codebase. As you continue to refine your Java programming skills, consider adopting the Factory Method pattern to simplify complex object creation scenarios and embrace the benefits of a more streamlined and maintainable codebase.
In conclusion, the Factory Method pattern stands as a valuable tool for simplifying object creation and enhancing the overall structure of Java applications.
Remember, mastering design patterns is an ongoing journey, and incorporating the Factory Method pattern into your Java programming arsenal can be a pivotal step towards writing cleaner, more maintainable code.
By leveraging the power of design patterns, such as the Factory Method pattern, Java developers can streamline their code, improve maintainability, and pave the way for future scalability and extensibility.
So, embrace the Factory Method pattern and unlock the potential to simplify and elevate your Java code!
Checkout our other articles