Java 8 Collections: Master Element Extraction Easily!
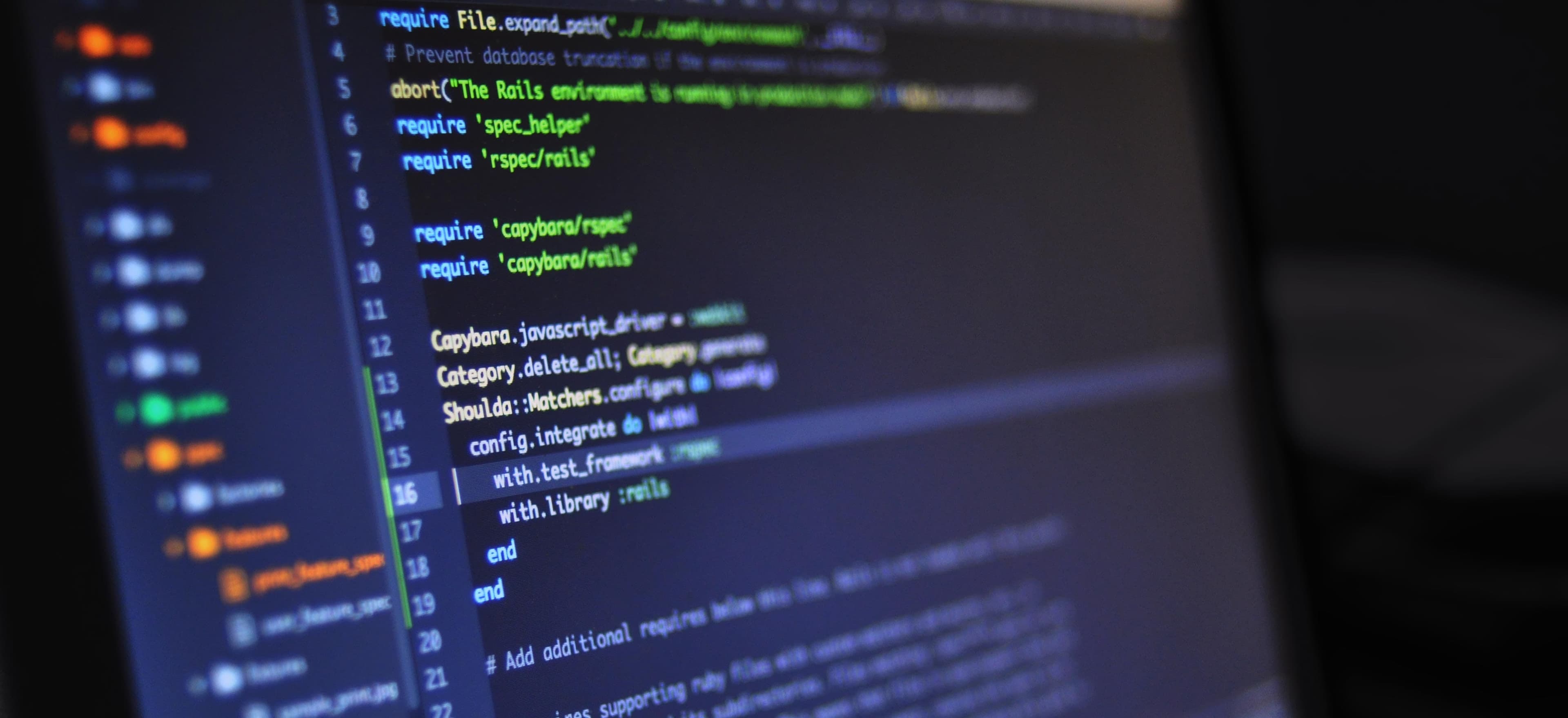
- Published on
Master Element Extraction with Java 8 Collections
In the world of programming, working with collections of data is a common task. Java, being one of the most widely used programming languages, offers a powerful set of tools for working with collections. With the introduction of Java 8, several new features and enhancements were added to the collections framework, making it even more versatile and efficient.
One of the most useful features introduced in Java 8 is the ability to easily extract elements from a collection using lambda expressions and the Stream API. This allows developers to succinctly and elegantly manipulate collections, reducing the need for boilerplate code and iteration loops.
In this post, we will explore how to master element extraction in Java 8 collections, taking advantage of the new features to simplify and streamline our code.
Filtering Elements with Predicate
In Java 8, the java.util.function.Predicate
interface represents a predicate (a boolean-valued function) of one argument. This interface is used to define a condition that can be used to filter elements in a collection.
Let's say we have a list of numbers and we want to extract all the even numbers from it. Using Java 8, we can achieve this with ease using the Predicate
interface and the filter
method from the Stream API.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0) // Using a predicate to filter even numbers
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6, 8, 10]
In this example, we create a stream from the list of numbers and use the filter
method along with a lambda expression that represents the condition for filtering even numbers.
Mapping Elements with Function
Another powerful feature in Java 8 is the ability to transform elements in a collection using the map
method from the Stream API. This is particularly useful when you need to apply a transformation to each element in the collection.
Consider a scenario where we have a list of strings representing numbers, and we want to extract the integer values from them. We can use the map
method to achieve this effortlessly.
List<String> numberStrings = Arrays.asList("1", "2", "3", "4", "5");
List<Integer> numbers = numberStrings.stream()
.map(Integer::parseInt) // Using a function to map strings to integers
.collect(Collectors.toList());
System.out.println(numbers); // Output: [1, 2, 3, 4, 5]
In this example, we use the map
method along with a method reference to Integer::parseInt
to convert each string to its corresponding integer value.
Reducing Elements with BinaryOperator
The reduce
method in Java 8 allows us to perform a reduction on the elements of a stream, using an associative accumulation function, and returns an Optional
describing the reduced value.
Let's say we have a list of numbers and we want to find the sum of all the elements in the list. We can achieve this easily using the reduce
method with a binary operator.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
Optional<Integer> sum = numbers.stream()
.reduce(Integer::sum); // Using a binary operator to reduce elements to a sum
System.out.println(sum.orElse(0)); // Output: 15
In this example, we use the reduce
method with the Integer::sum
binary operator to calculate the sum of all the numbers in the list.
Key Takeaways
In this post, we have explored some of the powerful features introduced in Java 8 for mastering element extraction in collections. By leveraging lambda expressions, the Stream API, and functional interfaces like Predicate
and Function
, we can manipulate collections with ease and conciseness.
Java 8's enhanced collections framework has revolutionized the way we work with data, allowing for more expressive and efficient code. As you continue to explore and master these features, you will find yourself writing cleaner, more readable, and more maintainable code.
So go ahead, dive into Java 8 collections, and unleash the full potential of element extraction in your programs!
To further expand your knowledge of Java 8 collections, you can explore the official Java documentation on Collections and Stream API.
Happy coding!
Checkout our other articles