Solving the Dreaded OutOfMemoryError: A Deep Dive
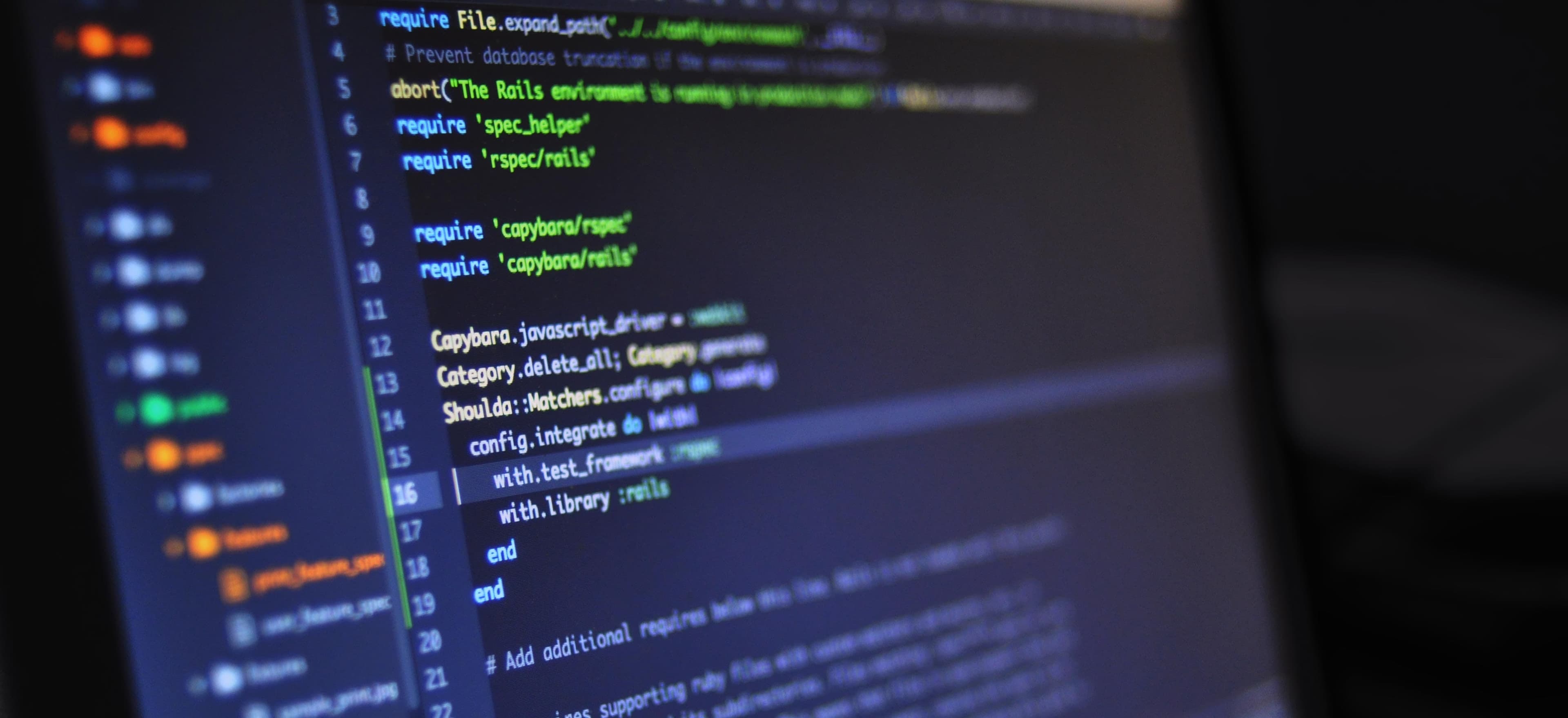
- Published on
Solving the Dreaded OutOfMemoryError: A Deep Dive
As a Java developer, encountering the dreaded OutOfMemoryError
can be a frustrating and time-consuming experience. This error occurs when the Java Virtual Machine (JVM) cannot allocate enough memory to fulfill an operation, resulting in the termination of the application. In this blog post, we will explore the causes of OutOfMemoryError
and discuss various strategies to mitigate and resolve this issue.
Understanding OutOfMemoryError
The OutOfMemoryError
is a runtime exception that indicates that the JVM has run out of memory. This can happen for a variety of reasons, including:
- Memory Leaks: Objects not being properly garbage collected, leading to excessive memory consumption.
- Excessive Memory Allocation: Large objects or data structures being created without proper memory management.
- Inadequate Heap Size: The JVM's heap size is not large enough to accommodate the application's memory requirements.
Causes of OutOfMemoryError
Memory Leaks
Memory leaks occur when objects are no longer needed by the application but are still held in memory, preventing the garbage collector from reclaiming the memory they occupy. This often happens due to inadvertent strong references that prevent objects from being garbage collected. Common causes of memory leaks include:
- Unclosed Resources: Not properly releasing resources such as files, streams, or database connections.
- Static Collections: Holding references to objects in static collections that outlive their usefulness.
- Thread Local Storage: Storing objects in thread-local variables that remain in memory even after the thread has completed its execution.
Excessive Memory Allocation
Another common cause of OutOfMemoryError
is the allocation of large objects or data structures that exceed the available heap space. This can occur when processing large datasets, handling large files, or inefficiently managing memory-intensive operations.
Inadequate Heap Size
The default heap size allocated to a Java application might not be sufficient to handle its memory requirements. This can result in OutOfMemoryError
when the application attempts to allocate more memory than the JVM can provide.
Mitigating OutOfMemoryError
Monitoring and Profiling
Before diving into code changes, it's important to monitor and profile the application to understand its memory usage patterns. Tools like VisualVM, Java Mission Control, and YourKit can provide insights into memory allocation, live objects, and garbage collection behavior.
Efficient Memory Management
Java provides several features and best practices for efficient memory management, such as:
- Proper Resource Management: Ensure that resources are released using try-with-resources or finally blocks to prevent resource leaks.
- Garbage Collection Tuning: Explore different garbage collection strategies and options to optimize memory usage and alleviate the pressure on the heap.
Identifying Memory Hotspots
Profiling the application can help identify memory hotspots—areas of the code where excessive memory is being allocated or retained. By pinpointing these hotspots, developers can focus on optimizing memory usage in specific areas of the application.
Memory-efficient Data Structures
Consider using memory-efficient data structures and algorithms to minimize memory overhead. For example, using primitive data types instead of their object counterparts can significantly reduce memory consumption.
Increase Heap Size
If the application's memory requirements exceed the default heap size, consider increasing the heap size using the -Xmx
flag. However, this should be done cautiously, as allocating too much memory can lead to longer garbage collection pauses and potential performance degradation.
Resolving OutOfMemoryError
Analyzing Heap Dumps
In cases of frequent OutOfMemoryError
, analyzing heap dumps can provide valuable insights into the memory usage of the application. Tools like Eclipse MAT (Memory Analyzer Tool) can help identify memory leaks, large object instances, and memory-retaining paths.
Leverage Soft, Weak, and Phantom References
Java provides soft, weak, and phantom references as part of its reference object mechanism. Utilizing these reference types can help manage memory more efficiently, especially for caching or temporary object storage scenarios.
Optimizing Object Reuse
In scenarios where creating new objects is a major contributor to memory consumption, consider implementing object pooling or reusing existing objects to minimize the overhead of object creation and garbage collection.
Using Memory Profiling Tools
Memory profiling tools can aid in identifying memory consumption patterns and identifying areas for optimization. By analyzing memory allocation and retention, developers can make informed decisions about memory optimization strategies.
Optimize Large Data Operations
When dealing with large datasets or files, consider optimizing data processing operations by reading and processing data in smaller, manageable chunks rather than loading the entire dataset into memory at once.
Bringing It All Together
The OutOfMemoryError
is a common yet challenging issue faced by Java developers. By understanding the causes, mitigation strategies, and resolution techniques outlined in this post, developers can effectively address and prevent OutOfMemoryError
occurrences in their applications.
Remember, proactive monitoring, efficient memory management, and targeted optimization are key to combating OutOfMemoryError
and ensuring the overall stability and performance of Java applications.
For further reference on memory management and troubleshooting OutOfMemoryError
, you can explore the Java Memory Management guide by Oracle and the Memory Analysis blog post by YourKit.
Happy coding and may your Java applications be free from memory woes!