Mastering Java: Why equals() and hashCode() Must Align
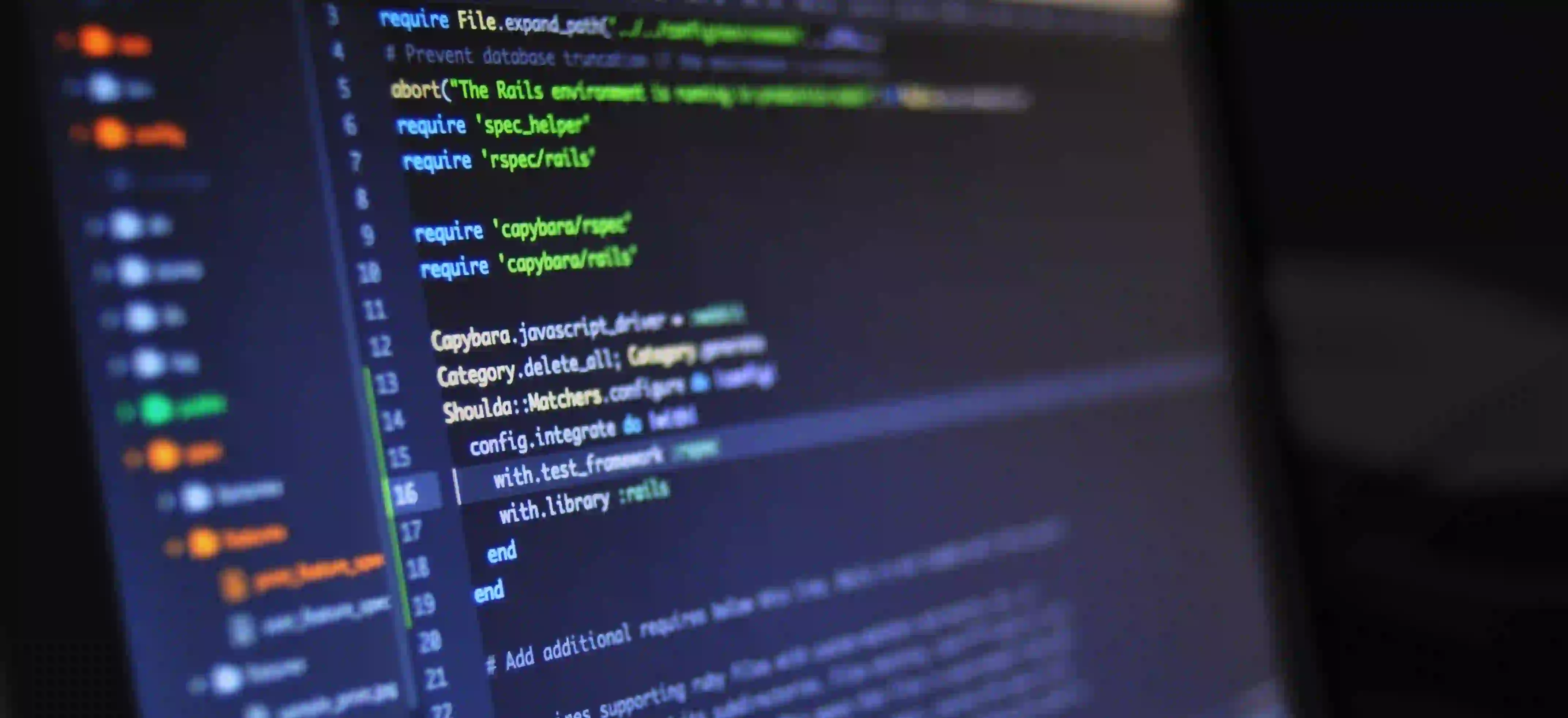
Mastering Java: Why equals() and hashCode() Must Align
When working with Java, you will often encounter situations where you need to compare objects for equality or put them into collections like Set, Map, or any data structure that relies on hashing. To ensure the proper functioning of these operations, it is crucial that the equals()
and hashCode()
methods are implemented correctly and that they align with each other. In this blog post, we will delve into the significance of aligning equals()
and hashCode()
in Java, understand how they interact with each other, and learn the best practices for implementing them.
Understanding equals() and hashCode()
equals()
The equals()
method in Java is used to check if two objects are equal. When you override the equals()
method in your class, you are essentially providing your own definition of equality for instances of that class. The default implementation of equals()
in the Object
class simply checks for reference equality, which is not suitable for most use cases.
hashCode()
The hashCode()
method is used to generate a unique integer value for an object. This integer value is used by hash-based data structures such as HashMap
and HashSet
. It is crucial that two objects that are equal according to the equals()
method must produce the same hash code.
The Contract Between equals() and hashCode()
According to the Java documentation, the two key points of the contract between equals()
and hashCode()
are:
- If two objects are equal according to the
equals()
method, then they must have the same hash code. - If two objects have the same hash code, they do not have to be equal.
Why Alignment is Important
The reason for aligning equals()
and hashCode()
in Java is to ensure the consistent behavior of objects in hash-based collections. When you add an object to a HashSet
or a HashMap
, the collection uses the object's hash code to determine the bucket to place the object into. Once the object is placed in the bucket, the equals()
method is used to identify the exact location of the object within the bucket. If the equals()
and hashCode()
methods are not aligned, the object may not be found in the collection or the behavior may be unpredictable.
To illustrate the importance of alignment, consider the following scenario where the equals()
and hashCode()
methods are not aligned:
public class Employee {
private String id;
private String name;
public Employee(String id, String name) {
this.id = id;
this.name = name;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Employee employee = (Employee) o;
return Objects.equals(id, employee.id) &&
Objects.equals(name, employee.name);
}
@Override
public int hashCode() {
return Objects.hash(id);
}
}
In this example, the hashCode()
method only considers the id
field, but the equals()
method takes into account both id
and name
. Now, if instances of Employee
are used in a HashSet
or HashMap
, the inconsistent behavior will lead to unexpected results.
Best Practices for Alignment
To ensure the proper alignment between equals()
and hashCode()
, follow these best practices:
-
Equality Criteria: Determine which fields of your class should be used to define equality and ensure they are all used in the
equals()
method. -
Consistency: Fields used in the
equals()
method should also be used in thehashCode()
calculation. -
Immutability: If a class is mutable, and the fields used in
equals()
andhashCode()
calculation can change, then the object's state can become inconsistent within hash-based collections. It is recommended to make the class immutable or use only immutable fields for comparison. -
Efficiency: The hash code calculation should be efficient, as it can impact the performance of hash-based collections.
Following these practices will not only ensure the alignment between equals()
and hashCode()
but also lead to predictable behavior of objects in hash-based collections.
Aligning equals() and hashCode() Example
Let's revisit the Employee
class and align the equals()
and hashCode()
methods:
public class Employee {
private String id;
private String name;
public Employee(String id, String name) {
this.id = id;
this.name = name;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Employee employee = (Employee) o;
return Objects.equals(id, employee.id) &&
Objects.equals(name, employee.name);
}
@Override
public int hashCode() {
return Objects.hash(id, name);
}
}
In this revised example, both the id
and name
fields are used in the hashCode()
method, aligning it with the equals()
method. Now, instances of Employee
can be used reliably in hash-based collections without any unexpected behavior.
Closing the Chapter
Aligning the equals()
and hashCode()
methods is essential for the proper functioning of hash-based collections in Java. By adhering to the contract between the two methods and following best practices for alignment, you can ensure the predictable behavior of objects in collections, avoiding unexpected results and improving the overall integrity of your code.
In Java, mastering the alignment between equals()
and hashCode()
is a fundamental aspect of creating robust and reliable code. Embracing these principles will not only elevate your understanding of the Java language but also set you on the path to becoming a proficient Java developer.
Now that you have a comprehensive understanding of the significance of aligning equals()
and hashCode()
in Java, it's time to put this knowledge into practice and enhance the stability and predictability of your Java applications. Happy coding!
For further reading and deepening your understanding, consider the official Java documentation on Object.hashCode() and Object.equals().