Master Gatling: Overcome Your First Load Test Hurdles
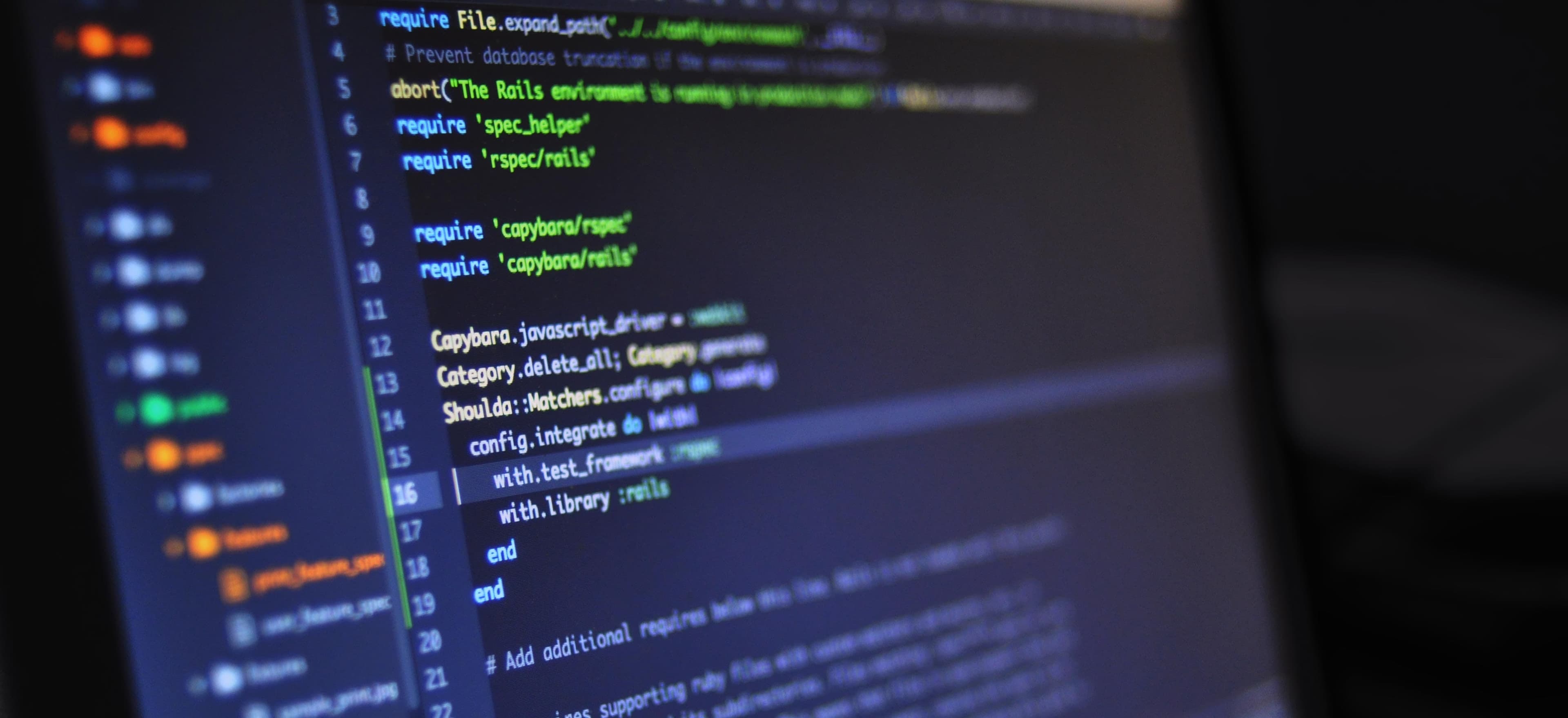
- Published on
Master Gatling: Overcome Your First Load Test Hurdles
If you are in the realm of software development, you are no stranger to the importance of load testing. It's a critical aspect of ensuring that your application can handle the expected load and perform consistently under pressure. When it comes to load testing in the Java ecosystem, Gatling is a name that frequently surfaces. This blog post is your guide to mastering Gatling and overcoming those initial hurdles in your load testing journey.
What is Gatling?
Gatling is an open-source load testing tool designed for ease of use, high performance, and scalability. Written in Scala, Gatling provides a domain-specific language for writing load tests, making it an excellent choice for developers familiar with Scala or Java. It's known for its expressive and intuitive DSL, which allows you to describe scenarios in a way that closely resembles how users interact with your application.
Setting the Stage: The Gatling Basics
Before diving into advanced Gatling features, let's cover the basics. It's essential to set up your environment correctly and understand the fundamental concepts of Gatling.
Installing Gatling
Gatling is easy to install and run, with minimal prerequisites. You can download the latest distribution from the official website and extract it to your preferred location.
Writing Your First Gatling Test
Creating a basic Gatling test involves defining a scenario and injecting some virtual users to simulate real-world traffic. Let's look at a simple example of a Gatling test that sends an HTTP GET request to a specified endpoint and asserts the response status.
import io.gatling.core.Predef._
import io.gatling.http.Predef._
class BasicSimulation extends Simulation {
val httpConf = http.baseURL("http://your.api.endpoint")
val scn = scenario("BasicSimulation")
.exec(http("request").get("/your/resource"))
setUp(scn.inject(atOnceUsers(10))).protocols(httpConf)
}
In the above code snippet, we define a scenario that makes an HTTP GET request to /your/resource
and inject 10 virtual users to execute this scenario.
Running Your Gatling Test
After writing your test, you can run it using the Gatling command-line interface provided in the distribution. Simply navigate to the Gatling directory and run the ./bin/gatling.sh
(for Unix-based systems) or ./bin/gatling.bat
(for Windows) script. Select your simulation and let Gatling do the rest.
Now that you have a minimal understanding of writing and running a Gatling test, let's move on to dealing with more complex scenarios and understanding some advanced Gatling features.
Advanced Gatling Concepts
Gatling offers a plethora of advanced features and concepts that can empower you to create comprehensive and realistic load tests.
Data Feeding
Real-world applications often rely on various input data. Gatling allows you to use feeders to provide data for your simulations. This can be particularly useful for testing scenarios with multiple data inputs, such as user credentials or API payloads.
val feeder = csv("user_credentials.csv").circular
val scn = scenario("DataFeedingSimulation")
.feed(feeder)
.exec(http("login").post("/login").formParam("username", "${username}").formParam("password", "${password}"))
In this example, we use a CSV feeder to provide user credentials and use them in the HTTP POST request to simulate the login process for multiple users.
Checkpoints and Assertions
Gatling allows you to define checkpoints in your scenarios to verify the responses from the server. These checkpoints serve as assertions, enabling you to ensure that the application behaves as expected under varying load conditions.
val scn = scenario("CheckpointSimulation")
.exec(http("request").get("/resource"))
.check(status.is(200))
.check(jsonPath("$.result").is("success"))
Here, we make a GET request and use checkpoints to verify that the response status is 200 and that the JSON payload contains a specific result.
Simulation Structure
As your load testing needs evolve, organizing and structuring your simulations becomes crucial. Gatling allows you to divide your tests into separate files or components and organize them according to the application's functionalities or use cases.
class Feature1Simulation extends Simulation {
// Define scenario and injection for feature 1
}
class Feature2Simulation extends Simulation {
// Define scenario and injection for feature 2
}
With this approach, you can maintain a modular and scalable structure for your load tests, making it easier to manage and extend them as your application grows.
Gatling Reports and Visualization
Gatling generates comprehensive test reports with rich visualizations, enabling you to analyze the performance metrics and identify potential bottlenecks. These reports include detailed metrics such as response times, throughput, and error rates, presented in intuitive graphs and tables.
Now that you've familiarized yourself with advanced Gatling concepts, it's time to address some common challenges and best practices in load testing with Gatling.
Common Challenges and Best Practices
Correlation and Session Management
When dealing with stateful applications that require maintaining user sessions, you may encounter challenges related to session management and correlation. Gatling provides mechanisms to handle session variables and correlation, ensuring that subsequent requests maintain the necessary state and context.
Dynamic Data and Parameterization
Real-world scenarios often involve dynamic data generation or parameterization. Gatling supports dynamic data generation through built-in functions and custom code, allowing you to create realistic test data and behaviors.
Scalability and Distributed Testing
As your testing requirements grow, you may need to scale your load tests across multiple machines or in a distributed environment. Gatling's architecture supports distributed testing, enabling you to distribute the load across multiple injectors and controllers for increased scalability and performance.
Continuous Integration and Automation
Integrating Gatling tests into your CI/CD pipelines and automating the test execution process is essential for achieving a seamless and efficient testing workflow. Gatling provides plugins for popular CI/CD tools such as Jenkins and TeamCity, allowing you to automate the execution and reporting of your load tests.
The Bottom Line
In conclusion, mastering Gatling for load testing in the Java ecosystem requires a solid understanding of its core concepts, advanced features, common challenges, and best practices. By leveraging Gatling's expressive DSL, advanced capabilities, and comprehensive reporting, you can create effective and realistic load tests to validate the performance of your applications. Embrace Gatling as your go-to tool for load testing, and pave the way for robust and scalable Java applications.