Overcoming Akka HTTP Challenges in Scala Web Development
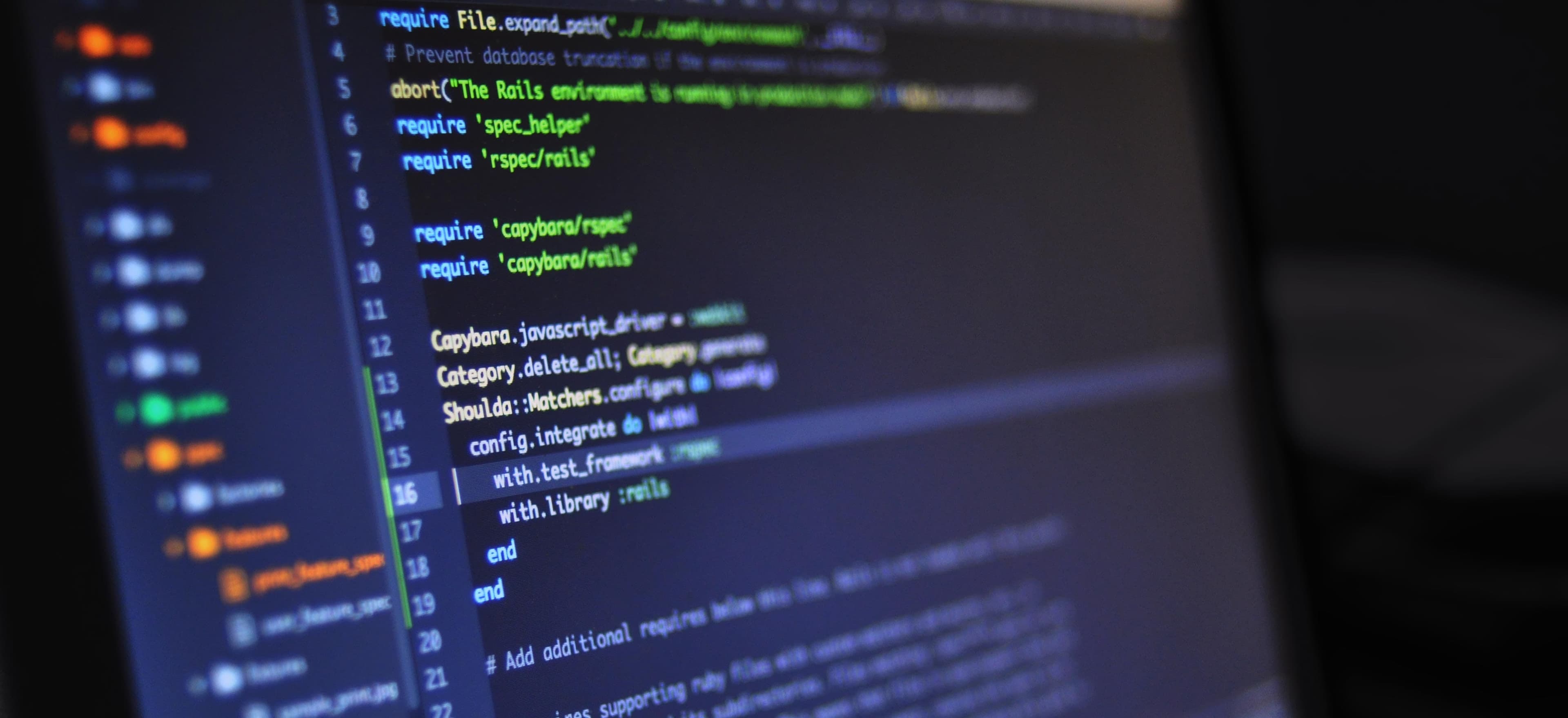
- Published on
Overcoming Akka HTTP Challenges in Scala Web Development
Scala has gained a substantial presence in the web development landscape, largely due to its concise syntax, functional programming capabilities, and seamless integration with Java. One of the key frameworks for building robust and scalable web applications in Scala is Akka HTTP. However, like any powerful tool, Akka HTTP comes with its own set of challenges that developers need to navigate.
In this post, we will delve into some common challenges faced when using Akka HTTP in Scala web development and explore effective strategies to overcome them.
Challenge 1: Complex Routing Logic
Creating a complex routing logic that effectively handles various endpoints, request methods, and query parameters can be a daunting task. Akka HTTP offers a flexible routing DSL, but as the application grows, managing and testing the routes can become increasingly challenging.
Solution:
One way to address this challenge is by organizing routes into smaller, reusable components using the ~
operator in Akka HTTP. This allows for better modularity and encapsulation of routing logic. Additionally, using the pathPrefix
directive can help in grouping related endpoints, thus improving the overall code organization.
val userRoutes: Route = pathPrefix("users") {
concat(
get {
// Get all users
},
post {
// Create a new user
}
)
}
val itemRoutes: Route = pathPrefix("items") {
concat(
get {
// Get all items
},
post {
// Create a new item
}
)
}
val combinedRoutes: Route = userRoutes ~ itemRoutes
Challenge 2: Asynchronous Request Handling
In a reactive system built with Akka HTTP, it's crucial to handle requests asynchronously to prevent blocking and ensure optimal resource utilization. Managing asynchronous operations and orchestrating responses can be intricate, especially when dealing with multiple concurrent requests.
Solution:
Akka HTTP integrates seamlessly with Akka actors, allowing for a natural way to manage asynchronous operations. By leveraging the onComplete
or flatMap
functions provided by Akka's Future, developers can handle asynchronous requests elegantly while keeping the codebase clean and maintainable.
val route: Route = path("async") {
get {
complete {
// Asynchronous operation using Akka's Future
Future {
// Perform asynchronous operation
}.map { result =>
// Process result and create response
}
}
}
}
Challenge 3: Error Handling and Fault Tolerance
Handling errors and ensuring fault tolerance is paramount in any web application. With Akka HTTP, effectively managing and propagating errors while maintaining the responsiveness of the system can be a challenging task.
Solution:
Akka HTTP provides directives such as handleExceptions
and handleRejections
to elegantly handle exceptions and rejections at the routing level. By defining custom exception handlers and rejection handlers, developers can tailor error responses based on specific use cases, ensuring a consistent and informative experience for the API consumers.
val route: Route = handleExceptions(myExceptionHandler) {
handleRejections(myRejectionHandler) {
// Define routes
}
}
Additionally, leveraging Akka's supervision strategies and actors can enhance fault tolerance within the application. By defining supervision strategies and hierarchical actor structures, developers can build resilient systems that gracefully handle failures.
Challenge 4: Testing Routes and APIs
Testing Akka HTTP routes and APIs presents its own set of challenges, particularly when it comes to setting up a test environment, mocking dependencies, and asserting responses.
Solution:
Akka HTTP provides a testkit that makes it relatively straightforward to write comprehensive tests for routes and APIs. By leveraging the testkit's utilities such as scalatest.RouteTest
, scalatest.HttpsSpec
, and scalatest.Matchers
, developers can simulate HTTP requests, validate responses, and assert the behavior of their routes with ease.
class MyRoutesSpec extends WordSpec with Matchers with ScalatestRouteTest {
val myRoutes = new MyRoutes().route
"MyRoutes" should {
"return a successful response for GET requests" in {
Get("/users") ~> myRoutes ~> check {
status shouldEqual StatusCodes.OK
// Assert other response attributes
}
}
}
}
Challenge 5: Performance Optimization and Caching
In high-performance web applications, optimizing response times and leveraging caching mechanisms play a crucial role. Akka HTTP, being built on top of Akka, offers powerful tools for optimizing performance, but effectively utilizing these features can be a challenge.
Solution:
By incorporating Akka actors and leveraging Akka's ask
pattern for non-blocking request/response interactions, developers can build efficient and responsive APIs. Additionally, integrating caching libraries such as Caffeine or Redis into the Akka HTTP application can significantly improve response times, reduce load on backend services, and enhance overall scalability.
val cache: LoadingCache[String, Future[Response]] = Caffeine.newBuilder().buildAsync((key: String) => {
// Retrieve data from database or external service
// Create and return Future[Response]
})
val route: Route = path("cached") {
get {
parameter("key") { key =>
onSuccess(cache.get(key)) { response =>
complete(response)
}
}
}
}
The Last Word
While Akka HTTP empowers developers to build responsive, scalable, and resilient web applications in Scala, it does come with its own set of challenges. By employing effective strategies such as modular routing, asynchronous request handling, error management, thorough testing, and performance optimization, developers can overcome these challenges and harness the full potential of Akka HTTP in their Scala web development endeavors.
In summary, understanding and embracing the reactive and asynchronous nature of Akka HTTP, along with leveraging Akka's powerful toolkit, are key to effectively addressing the challenges and building robust web applications in Scala.
I hope this post has provided you with valuable insights into overcoming Akka HTTP challenges in Scala web development. Happy coding!
Learn more about Akka HTTP: