Spring into Action: The Secret Sauce Every Developer Should Know
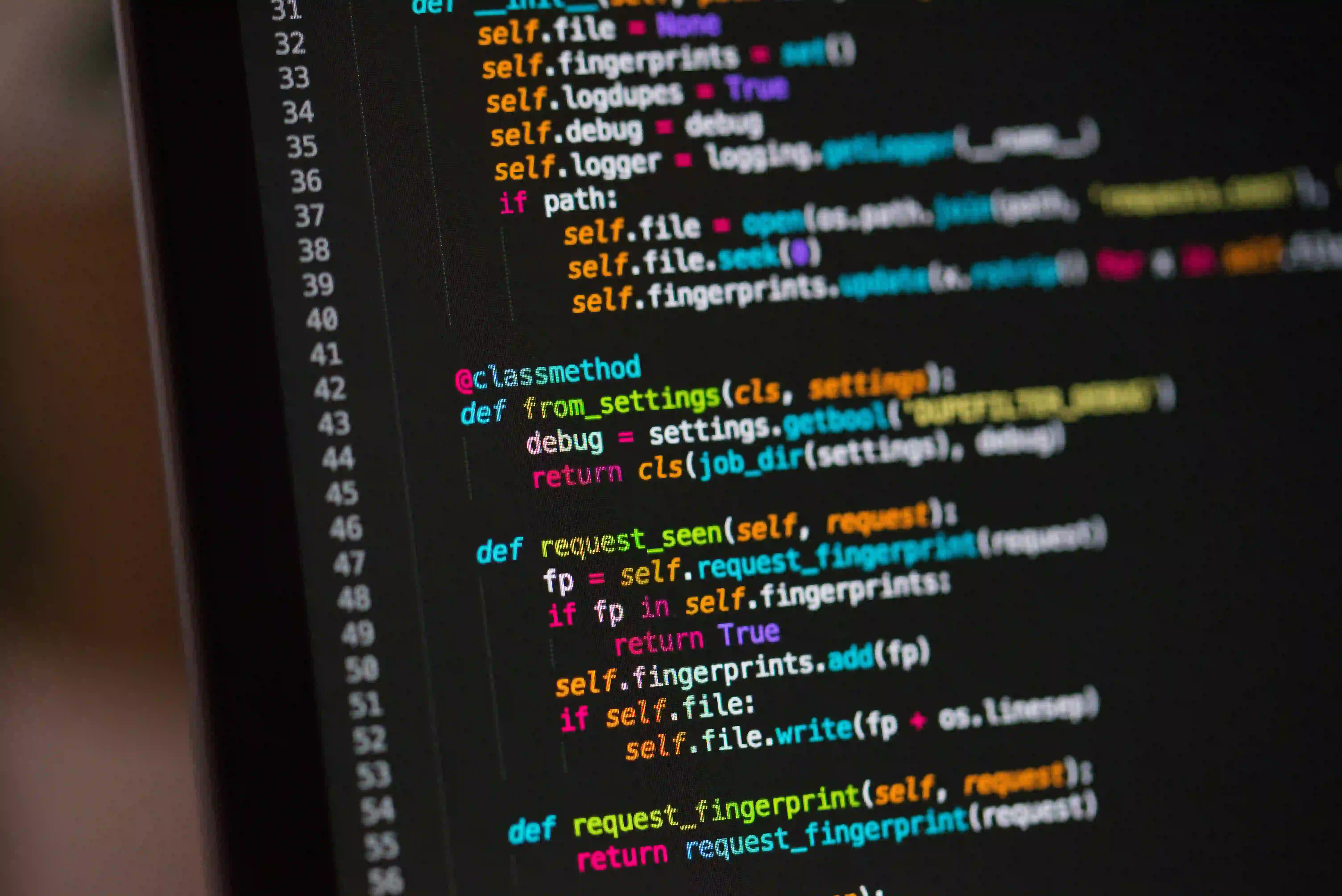
Spring into Action: The Secret Sauce Every Developer Should Know
Are you a Java developer looking to level up your skills and build better, more efficient applications? Look no further than Spring, the lightweight, powerful framework that has become a staple in the Java ecosystem. In this post, we'll explore the key concepts of Spring and how it can revolutionize the way you approach Java development.
What is Spring?
At its core, Spring is a comprehensive framework that provides support for building robust Java applications. It offers a wide range of features, including inversion of control (IoC), aspect-oriented programming (AOP), and integration with other Java technologies such as Hibernate and JPA.
Inversion of Control (IoC) Container
One of the fundamental principles of Spring is the IoC container, which manages the creation and configuration of objects. Instead of relying on the application to create instances of classes directly, the IoC container takes control of object creation and lifecycle management.
Let's take a look at a simple example of how the IoC container works in Spring:
public class MyApp {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
MessageService service = context.getBean(MessageService.class);
System.out.println(service.getMessage());
}
}
In this example, the ClassPathXmlApplicationContext
is the IoC container that loads the configuration from the beans.xml
file and creates the necessary objects. This allows for loose coupling and easier management of dependencies.
Aspect-Oriented Programming (AOP)
AOP is a programming paradigm that allows developers to modularize cross-cutting concerns, such as logging, security, and transaction management. Spring provides robust support for AOP, allowing developers to define aspects and apply them to the codebase without cluttering the business logic.
Here's a simplified example of how AOP works in Spring:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature());
}
}
In this example, the LoggingAspect
defines a pointcut that targets all methods within the com.example.service
package. When a method is invoked within that package, the logBefore
advice is triggered before the method execution.
The Spring Ecosystem
Spring is more than just a framework; it's a robust ecosystem that offers a wide array of projects and extensions to address various aspects of enterprise application development. Some key components of the Spring ecosystem include:
Spring Boot
Spring Boot is a game-changer for Java developers, providing a streamlined way to create stand-alone, production-grade Spring-based applications. By embracing convention over configuration, Spring Boot eliminates much of the boilerplate code typically associated with Java development.
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
With just a few lines of code, you can create a fully functional Spring application with embedded servers and simplified deployment.
Spring Data
Spring Data aims to provide a consistent, high-level programming model for data access, while still retaining the special traits of the underlying data store. It offers support for relational databases, NoSQL data stores, and more.
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByLastName(String lastName);
}
By extending the JpaRepository
interface, developers can leverage powerful features such as query methods, automatic CRUD operations, and pagination support with minimal effort.
Why Spring?
Now that we've covered the basics of Spring, you might be wondering why you should invest your time and effort into learning this framework. Here are a few compelling reasons:
-
Simplicity and Productivity: Spring's core philosophy is to simplify the development of enterprise applications. With its dependency injection and AOP support, developers can focus on business logic without getting bogged down by infrastructure concerns.
-
Modularity and Extensibility: Spring's modular design allows developers to cherry-pick the components they need, making it easy to adapt to evolving project requirements. Additionally, the extensibility of Spring enables seamless integration with other libraries and frameworks.
-
Robust Community and Support: With a vibrant community and extensive documentation, getting help and staying updated with the latest best practices is a breeze. Whether it's on Stack Overflow, forums, or official documentation, you're never alone in your Spring journey.
-
Industry Adoption and Job Opportunities: Spring is widely adopted across industries, making it a valuable skill for developers seeking new opportunities. Many organizations look for Java developers with Spring expertise due to its proven track record in building scalable, maintainable applications.
Getting Started with Spring
If you're ready to dive into the world of Spring development, there are plenty of resources available to help you get started. You can begin by exploring the official Spring documentation and familiarizing yourself with the core concepts and best practices.
For hands-on experience, consider working on sample projects, taking online courses, or participating in open-source contributions. By immersing yourself in practical scenarios, you'll gain a deeper understanding of Spring's capabilities and how to leverage them effectively in real-world scenarios.
Final Thoughts
In conclusion, Spring is a versatile, powerful framework that has solidified its place as a cornerstone of Java development. By mastering the key concepts and components of Spring, developers can streamline their workflow, build scalable applications, and stay ahead in the ever-evolving software landscape.
So, whether you're a seasoned Java developer or just starting your journey, embracing Spring can elevate your skills and open up new possibilities in the world of enterprise application development.
So, are you ready to spring into action with Spring? The secret sauce awaits!