Avoiding Eternal Bugs: The Cardinal Sin of Coding
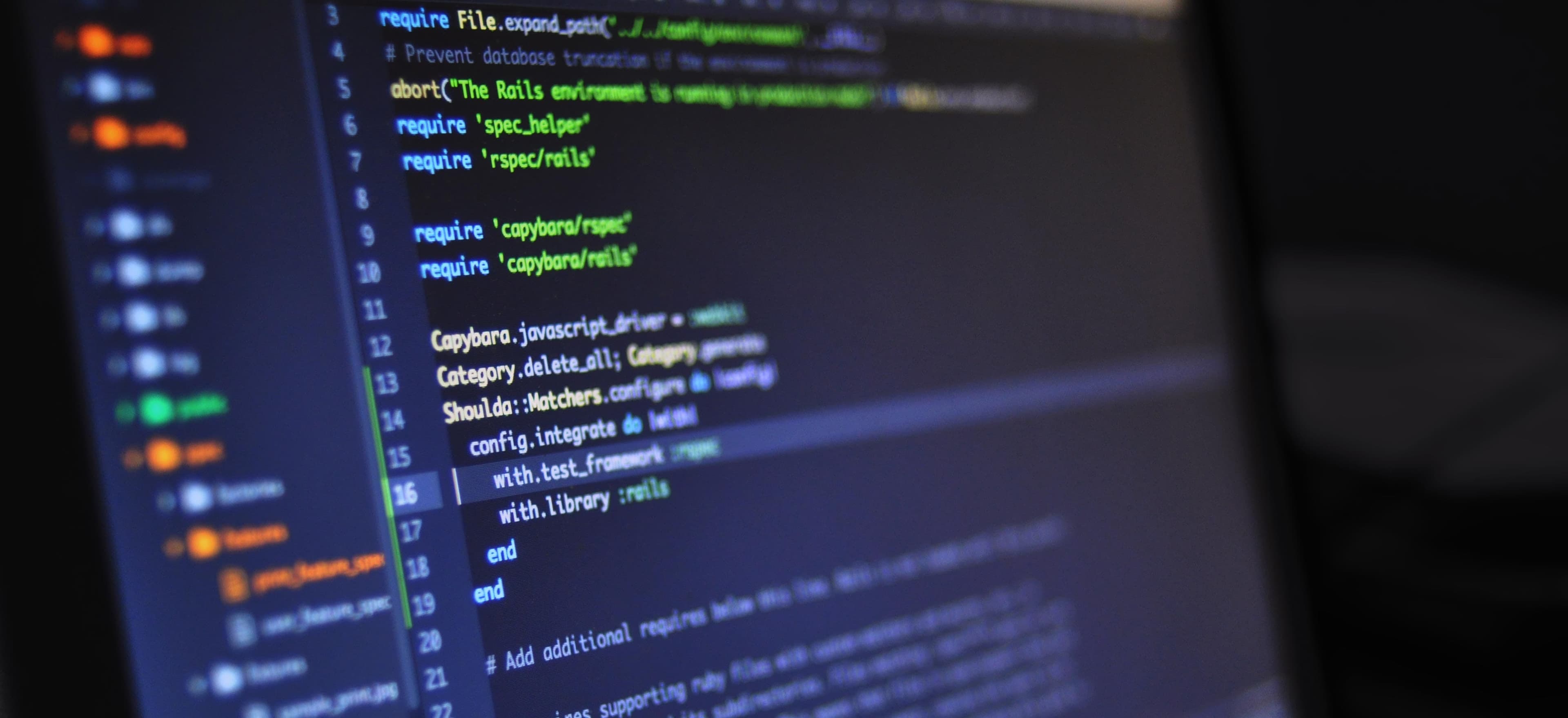
- Published on
The Cardinal Sin of Coding: Ignoring Exception Handling in Java
In the world of Java programming, one cardinal sin that many developers commit is ignoring or bypassing exception handling. Exception handling is crucial for writing robust and reliable code, yet it is often overlooked or relegated to an afterthought. This negligence can result in code that is prone to bugs, crashes, and unexpected behavior, leading to a myriad of issues for both developers and end-users.
The Consequences of Ignoring Exception Handling
When exception handling is neglected, a Java program becomes vulnerable to unpredictable errors. It's like driving a car without brakes – a disaster waiting to happen. Unhandled exceptions can cause the program to crash, leave resources unclosed, or even compromise data integrity. In the worst-case scenario, unhandled exceptions can lead to security vulnerabilities, making the application susceptible to exploitation.
The Java try-catch
Block: A Shield Against Chaos
Java provides the try-catch
block for handling exceptions. It allows developers to encapsulate error-prone code within a try
block and provide corresponding handling or recovery code within the catch
block. This mechanism shields the program from crashing and enables graceful error recovery.
Example of try-catch
Block
try {
// Code that may throw an exception
Files.readAllLines(Paths.get("file.txt"));
} catch (IOException e) {
// Handle the exception, log the error, or perform recovery actions
System.err.println("An error occurred: " + e.getMessage());
}
In this example, the try
block encapsulates the code that attempts to read lines from a file. If an IOException
is thrown, the catch
block handles the exception by printing an error message. This prevents the program from crashing and allows for appropriate action to be taken.
The 'Why' Behind the Code
The try-catch
block is not just a syntactical requirement; it's a fundamental aspect of writing stable and reliable code. It forces developers to explicitly acknowledge and address potential failure points, promoting a defensive programming mindset.
Throwing and Catching Custom Exceptions
In addition to handling built-in exceptions, Java allows developers to create and throw custom exceptions tailored to their specific application logic. This empowers developers to convey precise error information and propagate exceptions to higher layers of the application for central handling.
Example of Custom Exception
class CustomException extends Exception {
CustomException(String message) {
super(message);
}
}
class CustomExceptionExample {
void performTask() throws CustomException {
// Condition that requires throwing a custom exception
throw new CustomException("An error occurred in performTask");
}
}
public class Main {
public static void main(String[] args) {
CustomExceptionExample example = new CustomExceptionExample();
try {
example.performTask();
} catch (CustomException e) {
// Handle the custom exception
System.err.println("Custom Exception: " + e.getMessage());
}
}
}
In this example, a custom exception CustomException
is created by extending the base Exception
class. The performTask
method throws the custom exception when a specific condition is met. In the main
method, the custom exception is caught and handled appropriately.
The 'Why' Behind the Code
Custom exceptions enable developers to classify and communicate application-specific errors effectively. By throwing and catching custom exceptions, developers can maintain a consistent error-handling strategy throughout the application, contributing to codebase coherency.
Utilizing finally
Block for Resource Cleanup
Beyond handling exceptions, proper resource management is essential in Java programming. The finally
block ensures that resources such as open files, database connections, or network sockets are reliably released, regardless of whether an exception occurs or not.
Example of finally
Block
FileWriter fileWriter = null;
try {
fileWriter = new FileWriter("output.txt");
fileWriter.write("Hello, World!");
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
} finally {
// Ensure the file writer is closed, even in the presence of an exception
if (fileWriter != null) {
try {
fileWriter.close();
} catch (IOException e) {
System.err.println("Error closing file writer: " + e.getMessage());
}
}
}
In this example, the finally
block guarantees that the fileWriter
is closed, regardless of whether an exception is thrown during the write operation. This prevents resource leaks and ensures proper cleanup.
The 'Why' Behind the Code
The finally
block is a critical component of resource management. It upholds the principle of releasing resources in a timely manner, preventing potential resource exhaustion and promoting efficient utilization of system resources.
The Advantages of Proper Exception Handling in Java
Adhering to sound exception handling practices in Java yields numerous benefits. It leads to more resilient and stable software, reduces debugging efforts, enhances code maintainability, and fortifies the overall quality of the codebase. By acknowledging and addressing potential failure scenarios, developers can instill confidence in their code's reliability and create a positive user experience.
Wrapping Up
Exception handling is not just a formality – it's a cornerstone of robust and dependable Java programming. By embracing the try-catch
block, custom exceptions, and proper resource management with the finally
block, developers can fortify their code against catastrophe and steer clear of the cardinal sin of coding. Remember, in the realm of Java development, neglecting exception handling is a costly oversight that no developer can afford.
In conclusion, prioritizing exception handling is not only a best practice but a fundamental responsibility that distinguishes great code from mediocre code. Ignoring this cardinal rule can lead to dire consequences, while embracing it ensures the resilience and reliability of Java applications.
Want to delve deeper into Java exception handling? Check out the official Java documentation for comprehensive insights.
Remember, handle exceptions with care, and your code will thank you.
Checkout our other articles