Cracking the Code: Avoiding Cheats in N-Queens Puzzles
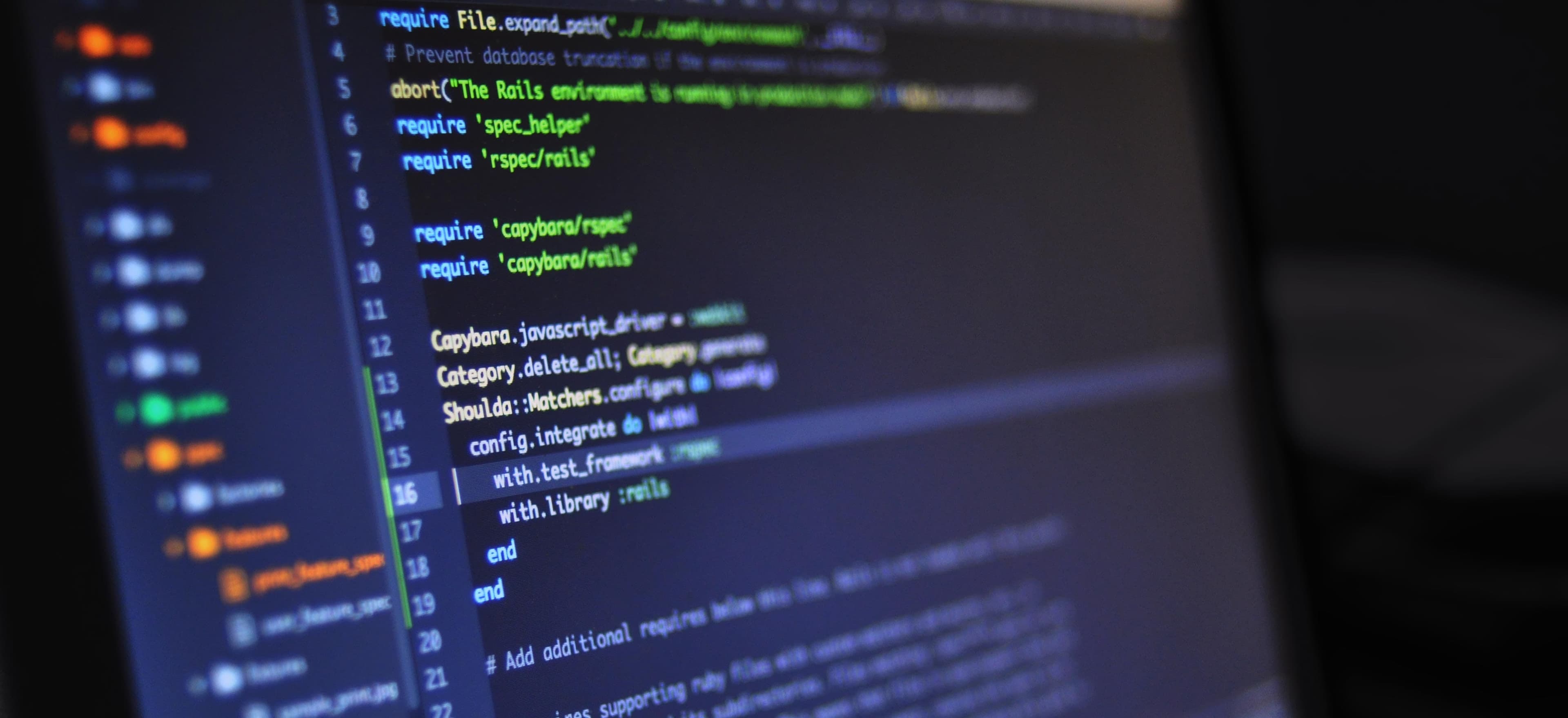
- Published on
Cracking the Code: Avoiding Cheats in N-Queens Puzzles
If you've ever delved into the fascinating world of problem-solving and computer science, you've likely encountered the N-Queens problem. It's a classic chess-based conundrum that challenges you to place N queens on an NxN chessboard without any two queens attacking each other. In this blog post, we'll explore how to solve the N-Queens problem using Java and discuss how to avoid cheating in the process.
The N-Queens Problem
Before we dive into the Java implementation, let's briefly revisit the basics of the N-Queens problem. The objective is to place N queens on an NxN chessboard in such a way that no two queens threaten each other. This means that no two queens share the same row, column, or diagonal.
A Brute Force Solution
A brute force approach to solving the N-Queens problem involves generating all possible configurations of queens on the board and checking each configuration to see if it's a valid solution. While this approach may work for smaller board sizes, it becomes increasingly inefficient as the board size grows.
Let's take a look at a simple brute force Java implementation for the N-Queens problem:
public class NQueens {
private static int[] queens;
private static int boardSize;
public static boolean isSafe(int row, int col) {
for (int i = 0; i < row; i++) {
if (queens[i] == col || queens[i] - i == col - row || queens[i] + i == col + row) {
return false;
}
}
return true;
}
public static void solveNQueens(int n) {
boardSize = n;
queens = new int[n];
solve(0);
}
private static void solve(int row) {
if (row == boardSize) {
// Found a solution, do something with it
return;
}
for (int col = 0; col < boardSize; col++) {
if (isSafe(row, col)) {
queens[row] = col;
solve(row + 1);
}
}
}
public static void main(String[] args) {
solveNQueens(4); // Solve for a 4x4 board
}
}
In this code snippet, we define a solveNQueens
method that initializes the board and calls the solve
method to find a solution. The isSafe
method checks whether it's safe to place a queen in a particular position on the board.
Optimizing the Solution
While the brute force approach can solve the N-Queens problem, it's not the most efficient solution, especially for larger board sizes. To avoid cheating in the puzzle-solving process, we can optimize the solution using backtracking and pruning techniques.
By employing these techniques, we can significantly reduce the number of unnecessary computations and avoid exploring unfeasible branches of the problem space.
Let's take a look at an optimized Java implementation for the N-Queens problem using backtracking:
public class NQueens {
private static int[] queens;
private static int boardSize;
private static List<List<Integer>> solutions;
public static boolean isSafe(int row, int col) {
for (int i = 0; i < row; i++) {
if (queens[i] == col || queens[i] - i == col - row || queens[i] + i == col + row) {
return false;
}
}
return true;
}
public static void solveNQueens(int n) {
boardSize = n;
queens = new int[n];
solutions = new ArrayList<>();
solve(0);
}
private static void solve(int row) {
if (row == boardSize) {
List<Integer> solution = new ArrayList<>();
for (int col : queens) {
solution.add(col);
}
solutions.add(solution);
return;
}
for (int col = 0; col < boardSize; col++) {
if (isSafe(row, col)) {
queens[row] = col;
solve(row + 1);
}
}
}
public static void main(String[] args) {
solveNQueens(4); // Solve for a 4x4 board
for (List<Integer> solution : solutions) {
System.out.println(solution);
}
}
}
In this optimized code snippet, we maintain a list of solutions and store valid solutions as they are found. This approach helps us avoid redundant computation and only explore viable paths in the problem space.
By implementing backtracking and pruning techniques, we can solve the N-Queens problem efficiently while ensuring that we don't cheat by taking shortcuts or missing potential solutions.
Closing the Chapter
In this blog post, we explored how to solve the N-Queens problem using Java and discussed how to avoid cheating in the process. We started by reviewing the basics of the N-Queens problem and then examined a brute force solution in Java. We then optimized the solution using backtracking and pruning techniques to improve efficiency and maintain integrity in the puzzle-solving process.
By understanding and implementing these techniques, you can tackle complex problem-solving tasks with confidence, knowing that you've arrived at a solution through legitimate means.
So go ahead, put your Java skills to the test, and crack the code of the N-Queens problem using efficient and cheat-free techniques!
For further reading on backtracking algorithms, you can explore this in-depth guide to backtracking.
Happy coding!
Checkout our other articles