Mastering Java EE and jOOQ Integration: A Beginner's Guide
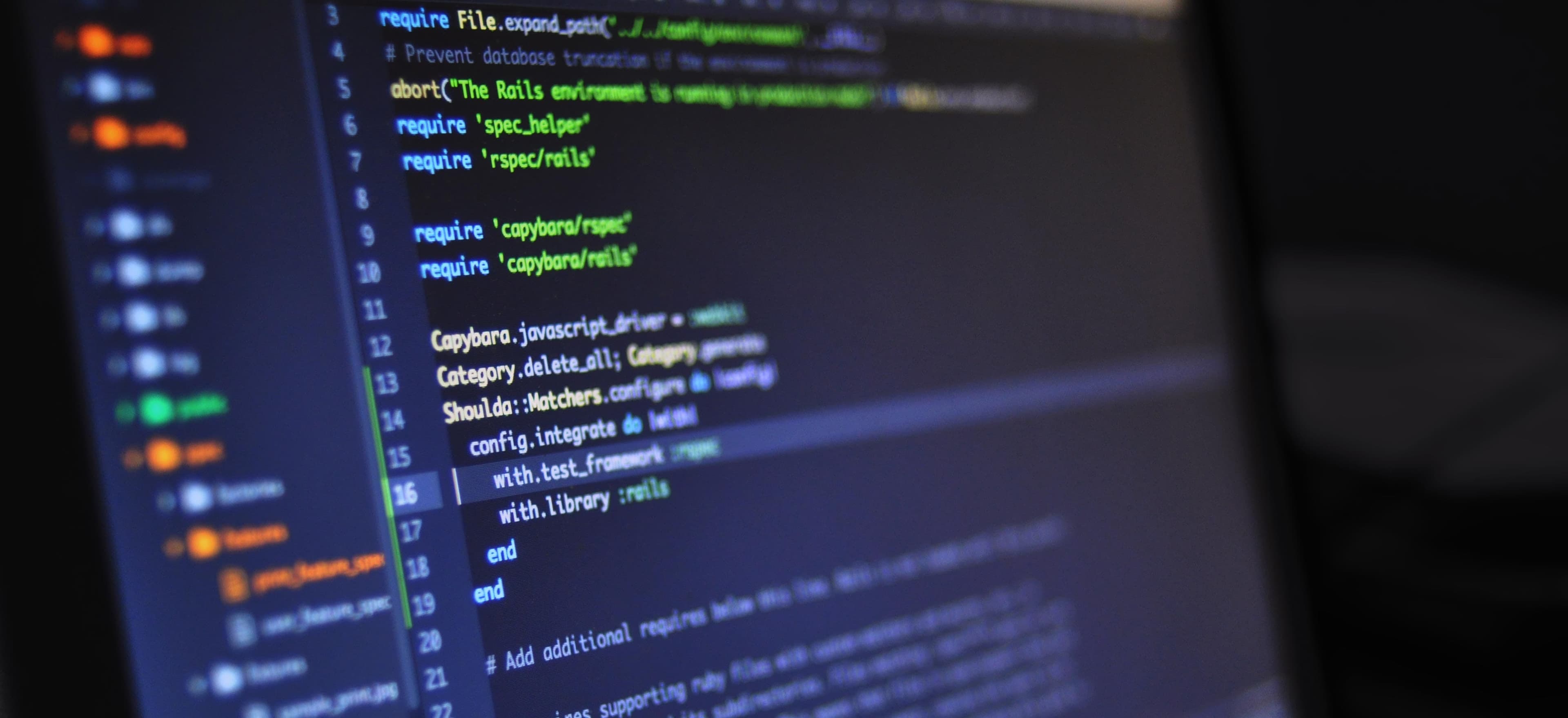
- Published on
Mastering Java EE and jOOQ Integration: A Beginner's Guide
As a Java developer, you understand the power and flexibility that comes with the Java EE platform. However, integrating SQL data manipulation into your Java applications can be challenging. This is where jOOQ (Java Object Oriented Querying) comes in. jOOQ is a powerful library that provides a fluent API for SQL query generation and execution, making it an excellent choice for integrating SQL into your Java EE applications.
In this guide, we will explore the fundamentals of integrating jOOQ into a Java EE application. We'll cover the basics of jOOQ, how to set it up in a Java EE project, and how to use it to perform SQL queries. By the end of this guide, you'll have a solid understanding of how to leverage jOOQ to bring the power of SQL into your Java EE applications.
What is jOOQ?
jOOQ is a library that allows you to write SQL queries in Java, using a fluent and typesafe API. It provides a domain-specific language (DSL) for writing SQL, making it easier to write and maintain complex SQL queries in your Java code. jOOQ supports various SQL dialects and provides great integration with Java, making it a popular choice for developers who want to leverage the power of SQL in their Java applications.
Setting Up jOOQ in a Java EE Project
To start using jOOQ in your Java EE project, you'll need to add the jOOQ library as a dependency. You can do this by including the jOOQ dependency in your project's build file. Here's an example using Maven:
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.14.4</version>
</dependency>
Once you have added the jOOQ dependency, you'll also need to include the JDBC driver for your database of choice (e.g., MySQL, PostgreSQL, SQL Server) as a dependency. This is necessary for jOOQ to communicate with your database.
Generating jOOQ Classes
After setting up the dependencies, the next step is to generate jOOQ classes. jOOQ provides a code generation tool that takes your database schema and generates Java classes that represent your database tables, records, and queries. This allows you to interact with your database using a type-safe API, making it easier to write and maintain database-related code.
To generate jOOQ classes, you'll need to configure the jOOQ code generation tool to connect to your database and generate the necessary classes. You can do this using a configuration file, where you specify the database connection details and other code generation options.
Here's an example of a jOOQ code generation configuration file:
<configuration xmlns="http://www.jooq.org/xsd/jooq-codegen-3.14.0.xsd">
<jdbc>
<driver>com.mysql.jdbc.Driver</driver>
<url>jdbc:mysql://localhost:3306/your_database</url>
<user>your_username</user>
<password>your_password</password>
</jdbc>
<generator>
<database>
<name>org.jooq.meta.mysql.MySQLDatabase</name>
<inputSchema>public</inputSchema>
</database>
<target>
<packageName>com.yourcompany.generated.jooq</packageName>
<directory>target/generated-sources/jooq</directory>
</target>
</generator>
</configuration>
Once you have configured the jOOQ code generation tool, you can run it to generate the jOOQ classes for your database. This will create Java classes that represent your database tables, records, and queries, which you can then use in your Java EE application to interact with the database.
Using jOOQ to Perform SQL Queries
Now that you have the jOOQ classes generated, you can start using them to perform SQL queries in your Java EE application. jOOQ provides a fluent API for building and executing SQL queries, which makes it easy to work with SQL in your Java code.
Here's an example of how you can use jOOQ to perform a simple SQL query to retrieve data from a database table:
DSLContext dsl = DSL.using(conn, SQLDialect.MYSQL);
Result<Record> result = dsl.select()
.from(Tables.AUTHOR)
.where(Tables.AUTHOR.ID.eq(1))
.fetch();
In this example, we're using the DSLContext
to create a SQL query that selects all columns from the AUTHOR
table where the ID
column is equal to 1. We then execute the query and retrieve the result as a Result
object.
The fluent API provided by jOOQ makes it easy to build complex SQL queries in a typesafe manner, ensuring that your queries are syntactically correct and safe from SQL injection attacks.
Closing Remarks
In this beginner's guide, we've covered the basics of integrating jOOQ into a Java EE application. We've discussed what jOOQ is, how to set it up in a Java EE project, and how to use it to perform SQL queries. By following the steps outlined in this guide, you'll be well on your way to mastering the integration of jOOQ in your Java EE applications.
Integrating jOOQ into your Java EE projects can bring the power and flexibility of SQL into your applications, allowing you to write complex and efficient database queries in a typesafe and maintainable manner. If you're looking to take your Java EE applications to the next level in terms of SQL integration, jOOQ is definitely worth exploring.
So, why wait? Dive into the world of jOOQ and take your Java EE applications to new heights by mastering the integration of SQL with jOOQ!