Boost Your Akka App: Mastering Java Tests Effortlessly
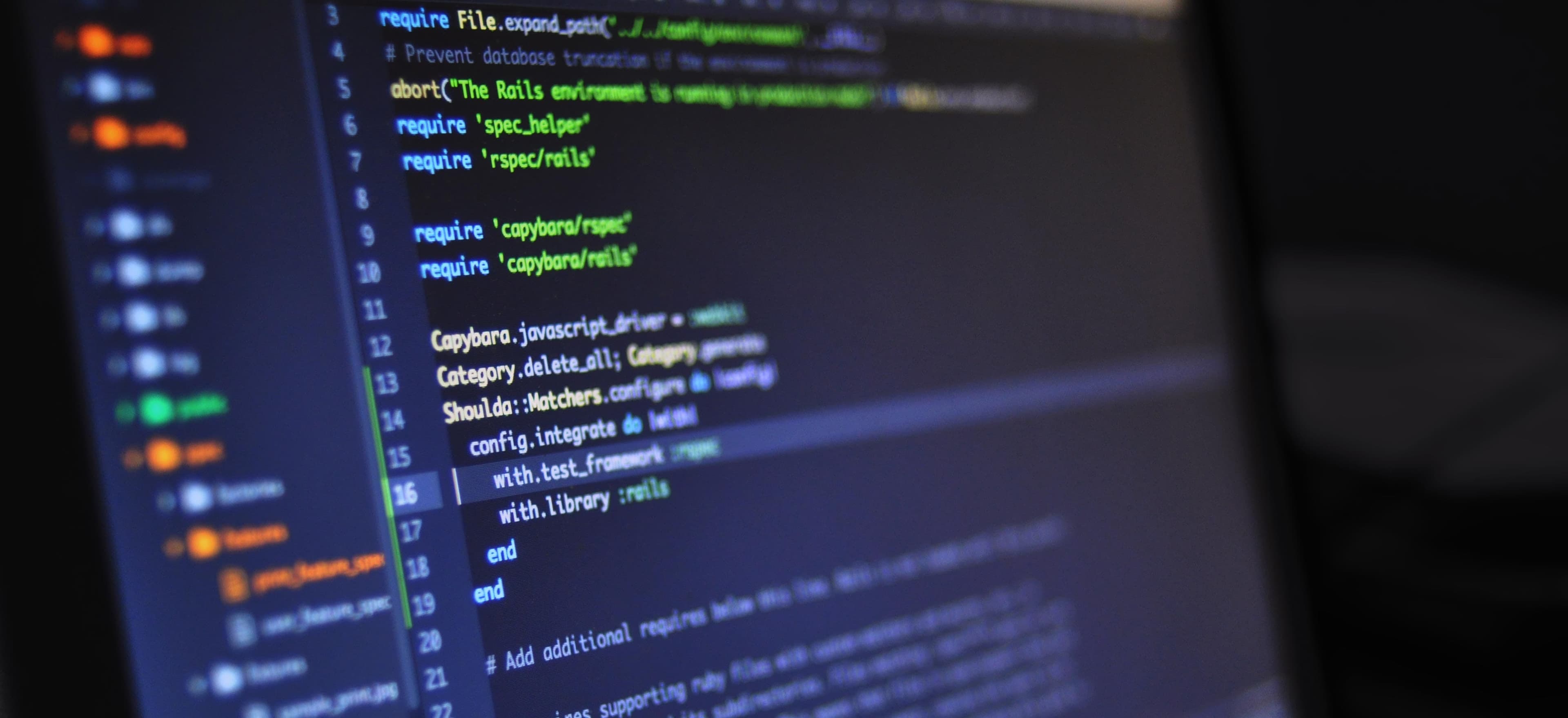
- Published on
Boost Your Akka App: Mastering Java Tests Effortlessly
Testing Java applications, especially those that involve complex asynchronous and concurrent behavior, can be a daunting task. When it comes to Akka, a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications on the JVM, testing becomes even more challenging. In this blog post, we will explore how to master Java tests effortlessly in Akka applications, ensuring the reliability and stability of your system.
Setting the Stage with Akka
Akka is known for its powerful actor-based model, allowing developers to create concurrent and distributed applications with ease. But with great power comes great responsibility, and testing such applications to ensure they function correctly under all conditions is non-negotiable. In the Akka ecosystem, effective testing is essential for building resilient and robust systems.
Leveraging Akka TestKit
To master testing in Akka, it's crucial to leverage the Akka TestKit. This toolkit provides the necessary utilities for testing actor systems and asynchronous code while asserting and mocking interactions between actors.
Creating Tests with Akka TestKit
Let's begin with a basic example of testing an Akka actor using Akka TestKit.
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.testkit.javadsl.TestKit;
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
import scala.concurrent.duration.Duration;
import java.util.concurrent.TimeUnit;
public class MyActorTest {
static ActorSystem system;
@BeforeClass
public static void setup() {
system = ActorSystem.create();
}
@AfterClass
public static void teardown() {
TestKit.shutdownActorSystem(system, Duration.create(5, TimeUnit.SECONDS), true);
system = null;
}
@Test
public void testActorBehavior() {
new TestKit(system) {{
final Props props = Props.create(MyActor.class);
final ActorRef subject = system.actorOf(props);
// Test the actor's behavior
subject.tell("Hello", getRef());
expectMsgEquals("Hello, World!");
}};
}
}
In this example, we create a simple test for an Akka actor using the Akka TestKit. We first initialize the ActorSystem
in the setup()
method and shut it down in the teardown()
method. Within the testActorBehavior()
method, we utilize the TestKit
to create and interact with the actor. We then send a message to the actor and assert the expected response using expectMsgEquals()
.
Explaining the Code
- The
TestKit
provides a test-friendly environment for interacting with actors and capturing their responses. Props.create(MyActor.class)
is used to create the properties for the actor we want to test.system.actorOf(props)
creates an instance of the actor within the test environment.expectMsgEquals("Hello, World!")
ensures that the actor's response matches the expected value.
Asynchronous Testing with Akka TestKit
Asynchronous testing is a common scenario in Akka applications, and Akka TestKit provides powerful tools to handle such cases gracefully.
Handling Future-Based Results
In Akka, it's common to work with Future
as a way to represent asynchronous computations. Let's see how Akka TestKit can handle scenarios involving Future
in testing.
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.dispatch.OnComplete;
import akka.pattern.Patterns;
import akka.testkit.javadsl.TestKit;
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
import scala.concurrent.Future;
import java.util.concurrent.TimeUnit;
import static akka.dispatch.Futures.future;
public class MyFutureActorTest {
static ActorSystem system;
@BeforeClass
public static void setup() {
system = ActorSystem.create();
}
@AfterClass
public static void teardown() {
TestKit.shutdownActorSystem(system, java.time.Duration.ofSeconds(5), true);
system = null;
}
@Test
public void testFutureActor() {
new TestKit(system) {{
final Props props = Props.create(MyFutureActor.class);
final ActorRef subject = system.actorOf(props);
// Test the actor's future-based behavior
Future<String> futureResult = Patterns.ask(subject, "Ping", 1000).mapTo(classTag(String.class));
futureResult.onComplete(new OnComplete<String>() {
@Override
public void onComplete(Throwable failure, String success) {
if (failure != null) {
throw new AssertionError("Future failed with " + failure.getMessage());
} else {
if (!success.equals("Pong")) {
throw new AssertionError("Unexpected response");
}
}
}
}, system.dispatcher());
subject.tell("Ping", ActorRef.noSender());
}};
}
}
Here, we are testing an actor that responds with a Future
. We use Patterns.ask()
to send a message to the actor and expect a Future
response. We then use onComplete()
to handle the result of the Future
, asserting the expected outcome based on the response.
Explaining the Code
Patterns.ask()
is used to send a message to the actor and expect aFuture
response within a specified timeout..mapTo(classTag(String.class))
ensures that the received result is of typeString
.futureResult.onComplete()
is used to handle the result of theFuture
, allowing us to make assertions based on the success or failure of the computation.
Mocking External Dependencies
In real-world applications, Akka actors often interact with external dependencies such as databases, external services, or other actors. To isolate the behavior of an actor under test and mock such dependencies, Akka TestKit provides the ability to create test probes.
Creating Test Probes
Let's take a look at how we can use test probes to mock the behavior of external dependencies in our tests.
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.testkit.javadsl.TestKit;
import akka.testkit.javadsl.TestProbe;
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
import java.util.Optional;
public class MyActorWithDependencyTest {
static ActorSystem system;
@BeforeClass
public static void setup() {
system = ActorSystem.create();
}
@AfterClass
public static void teardown() {
TestKit.shutdownActorSystem(system);
system = null;
}
@Test
public void testActorWithDependency() {
new TestKit(system) {{
final Props props = Props.create(MyActorWithDependency.class);
// Create a test probe to mock the dependency
TestProbe dependencyProbe = new TestProbe(system);
final ActorRef subject = system.actorOf(props);
// Inject the test probe as the dependency
subject.tell(new MyActorWithDependency.UseDependency(dependencyProbe.ref()), getRef());
// Verify interactions with the dependency
dependencyProbe.expectMsgClass(MyDependency.Perform.class);
// Simulate the response from the dependency
dependencyProbe.reply(new MyDependency.Response("Mocked response"));
// Verify the behavior of the actor based on the dependency's response
expectMsgEquals(Optional.of("Mocked response"));
}};
}
}
In this example, we create a test for an actor that depends on an external component, MyActorWithDependency
. We use a TestProbe
to mock the dependency and verify the interactions between the actor and the dependency. By injecting the test probe into the actor and simulating the response from the dependency, we can test the behavior of the actor under various conditions.
Explaining the Code
TestProbe
is used to create a mock for the external dependency, allowing us to verify interactions and simulate responses.dependencyProbe.expectMsgClass(MyDependency.Perform.class)
ensures that the actor interacts with the dependency by sending the expected message.dependencyProbe.reply(new MyDependency.Response("Mocked response"))
simulates the response from the dependency, enabling us to test the actor's behavior based on different responses.
Closing the Chapter
In this blog post, we've explored how to master Java tests effortlessly in Akka applications using the Akka TestKit. We've seen how to create tests for actors, handle asynchronous testing with futures, and mock external dependencies using test probes. By leveraging these techniques, you can ensure the reliability and resilience of your Akka applications through comprehensive and effective testing.
Mastering testing in Akka is essential for building robust and maintainable systems, and the Akka TestKit provides the necessary tools to achieve this. Embracing a test-driven mindset and incorporating thorough testing practices into your Akka development workflow will undoubtedly lead to more stable and resilient applications in the long run.
So, next time you dive into building or enhancing an Akka application, remember to equip yourself with the invaluable skills of mastering Java tests effortlessly using the Akka TestKit.
Start mastering Akka testing today and elevate your application's reliability and stability to new heights!
To delve deeper into Akka testing and explore additional resources, check out the official Akka documentation, which provides comprehensive insights into testing best practices and strategies for Akka applications.
Now go forth and test with confidence in your Akka universe!
By incorporating advanced testing techniques using the Akka TestKit in Java, developers can ensure the reliability and stability of their Akka applications. Through this comprehensive guide, you've learned how to master Java testing effortlessly, paving the way for resilient and robust systems. If you're interested in exploring further, don't hesitate to dive into the Akka documentation for a deeper understanding of testing best practices and strategies. Happy testing!
Is there anything specific about Akka testing you'd like to explore further? Let us know in the comments below!
Checkout our other articles