Solving EAI Challenges with Spring Integration
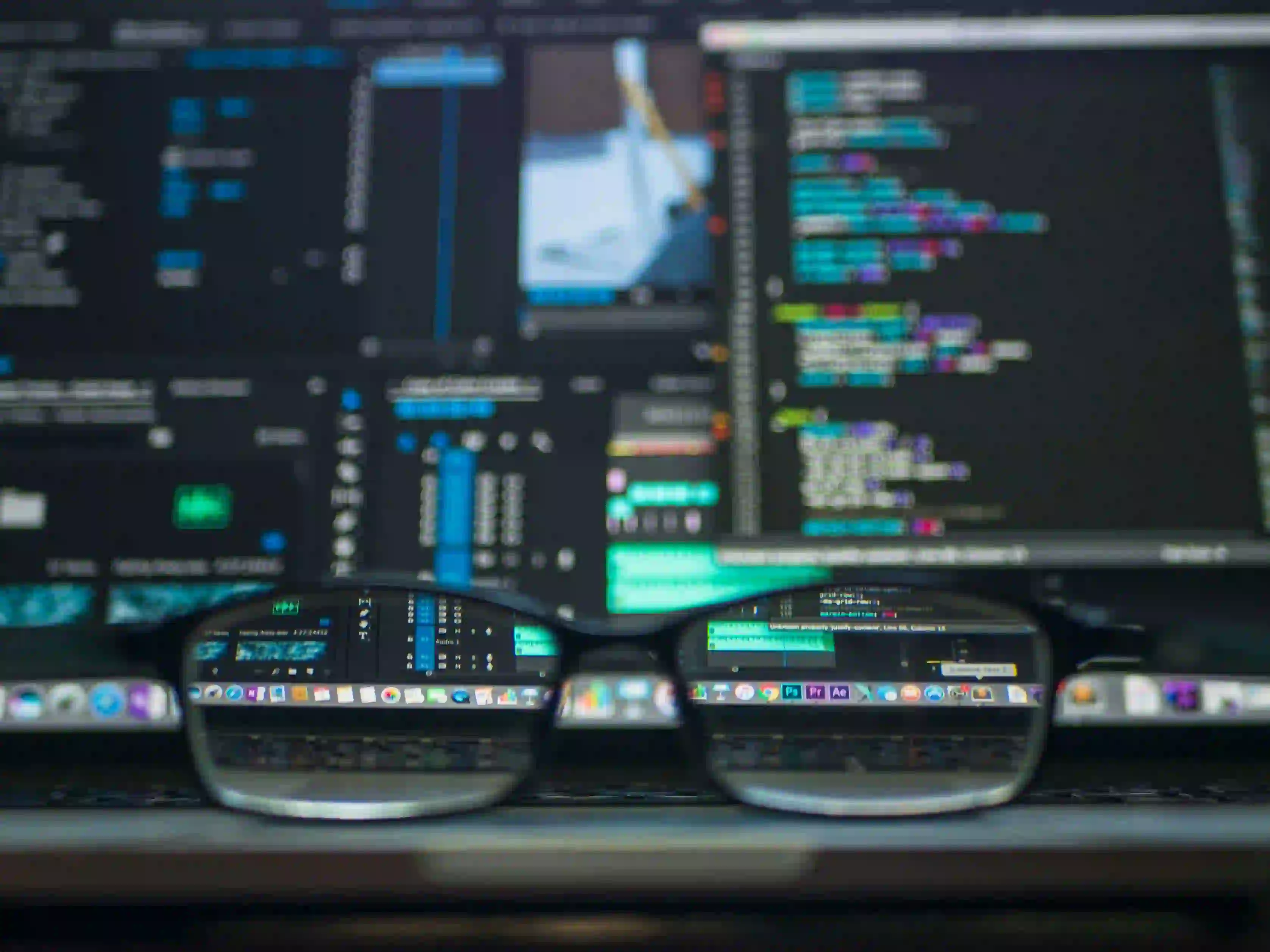
Solving EAI Challenges with Spring Integration
The Opening Bytes
In today's interconnected world, businesses rely on a variety of applications and systems to manage their operations. However, integrating these diverse systems can be a significant challenge. Enterprise Application Integration (EAI) aims to enable seamless communication and data exchange between different software applications within an organization.
One of the most popular tools for implementing EAI is Spring Integration, which provides a lightweight messaging framework to handle these integration challenges. In this article, we will explore how Spring Integration can be used to solve EAI challenges and provide seamless communication between different systems.
What is Spring Integration?
Before delving into how Spring Integration solves EAI challenges, let's briefly discuss what Spring Integration is. Spring Integration is an extension of the Spring programming model, which aims to simplify the building of enterprise integration solutions. It provides a set of lightweight messaging components that can be used to create robust and scalable integration solutions.
The key concept behind Spring Integration is the use of message-driven architecture, where messages are used to facilitate communication between different components of an application. These messages can carry data and be routed through a series of endpoints, allowing for flexible and decoupled integration patterns.
Solving EAI Challenges with Spring Integration
Now that we have a basic understanding of Spring Integration, let's explore how it can be used to address EAI challenges.
1. Protocol Transformation
One common challenge in EAI is the need to transform messages from one protocol to another. For example, while one system might use HTTP for communication, another system might rely on a message queue such as RabbitMQ. Spring Integration provides a range of components, such as Http.inboundGateway
and Amqp.inboundGateway
, that can handle protocol-specific message reception and transformation.
Example:
@Bean
public IntegrationFlow httpToAmqpFlow() {
return IntegrationFlows.from(Http.inboundGateway("/receive")
.requestMapping(m -> m.methods(HttpMethod.POST))
.requestPayloadType(String.class))
.handle(Amqp.outboundGateway(rabbitTemplate)
.routingKey("queue.name"))
.get();
}
In this example, an HTTP inbound gateway is used to receive messages, which are then transformed and sent to an AMQP (Advanced Message Queuing Protocol) queue using the Amqp.outboundGateway
component.
2. Message Routing
Another common EAI challenge is the need to route messages to different destinations based on specific criteria. Spring Integration provides a variety of components, such as Router
, RecipientListRouter
, and HeaderValueRouter
, that can be used to implement complex message routing logic based on message content or metadata.
Example:
@Bean
public IntegrationFlow messageRoutingFlow() {
return IntegrationFlows.from("inputChannel")
.<String, Boolean>route(payload ->
payload.startsWith("Important"),
mapping -> mapping
.subFlowMapping(true, sf ->
sf.handle("importantServiceActivator"))
.subFlowMapping(false, sf ->
sf.handle("ordinaryServiceActivator")))
.get();
}
In this example, a Router
is used to route messages based on whether they start with the word "Important". Messages meeting this criterion are sent to the importantServiceActivator
, while others are routed to the ordinaryServiceActivator
.
3. Error Handling and Retry Mechanisms
EAI solutions often require robust error handling and retry mechanisms to ensure message delivery and processing reliability. Spring Integration provides components such as errorChannel
for handling errors, and MessageRecoverer
for implementing custom retry logic.
Example:
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("input")
.handle("messageHandler")
.wireTap("auditLogChannel")
.get();
}
In this example, a simple error handling flow is demonstrated, where messages are handled by a messageHandler
and a wiretap is applied to route a copy of each message to an auditLogChannel
for error logging and analysis.
4. Content Enrichment and Aggregation
Content enrichment involves enhancing messages with additional data from external sources, while aggregation involves combining data from multiple messages into a single message. Spring Integration provides components such as Enricher
and Aggregator
to facilitate these tasks, improving the integration of systems with disparate data sources.
Example:
@Bean
public IntegrationFlow contentEnrichmentFlow() {
return IntegrationFlows.from("enricherInput")
.enrich(enricherSpec ->
enricherSpec.requestSubFlow(subFlow -> subFlow.handle("externalService"))
.<Object, String>transform(Object::toString))
.handle("finalService")
.get();
}
In this example, an Enricher
is used to enrich messages by invoking an external service and transforming the response before passing it to the finalService
.
To Wrap Things Up
In this article, we've explored how Spring Integration can be used to address EAI challenges such as protocol transformation, message routing, error handling, content enrichment, and aggregation. By leveraging its lightweight messaging components and flexible integration patterns, Spring Integration provides a powerful solution for building robust and scalable enterprise integration solutions.
As organizations continue to seek seamless connectivity between their disparate systems, the role of tools like Spring Integration in EAI will only become more critical. By mastering Spring Integration, developers can ensure that their integration solutions are efficient, reliable, and maintainable in the long term.
To discover more about Spring Integration, you can visit the official Spring Integration website and explore its comprehensive documentation and examples. Start integrating with Spring Integration today and unlock the potential for seamless communication and data exchange within your enterprise applications.