Unraveling Dropwizard Metrics for Reactive Apps Success
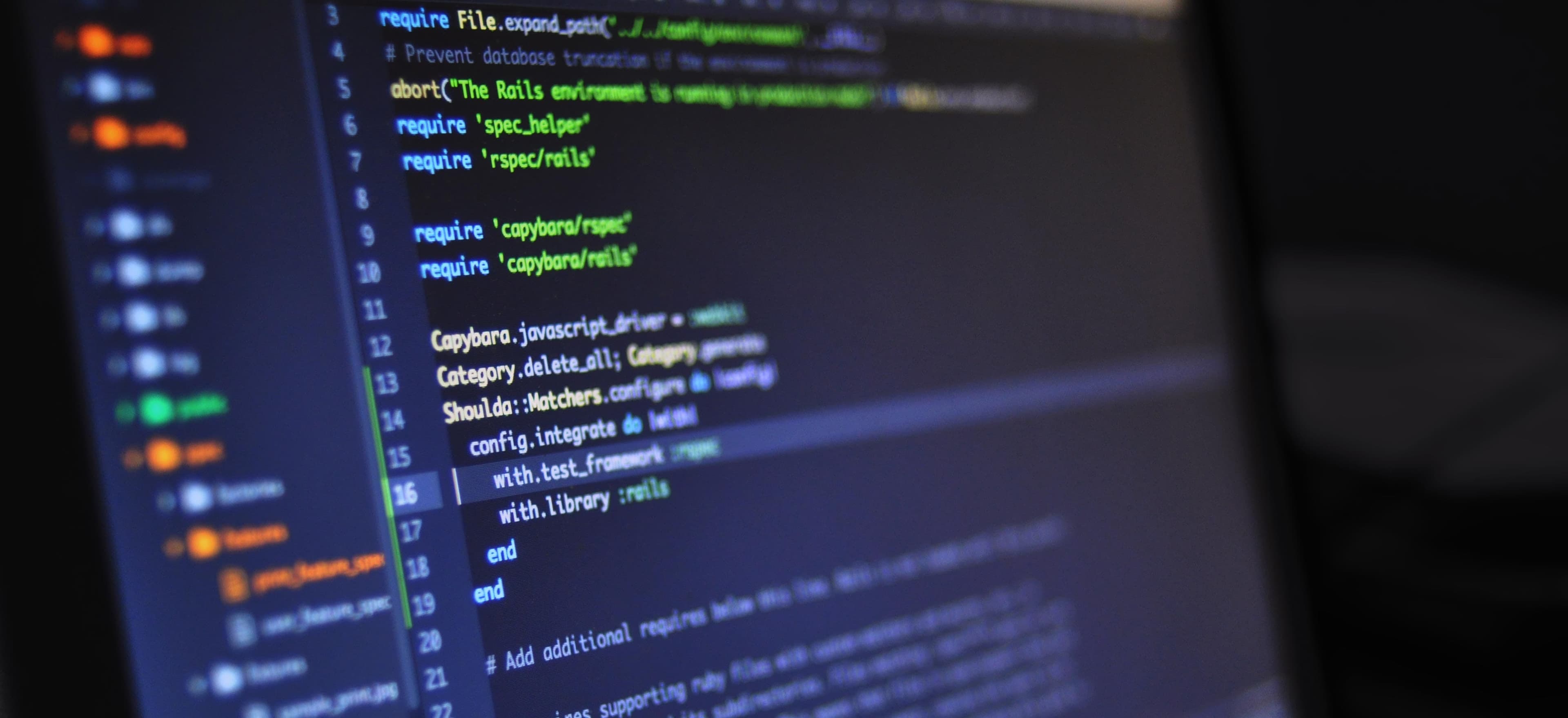
- Published on
Unraveling Dropwizard Metrics for Reactive Apps Success
In the realm of modern software development, reactive programming has gained significant traction due to its ability to handle asynchronous and event-driven scenarios. Java developers have embraced this paradigm shift to build highly responsive and scalable applications. As applications become more complex, the need for monitoring and understanding their behavior becomes critical. This is where Dropwizard Metrics comes into play, offering a robust solution for capturing, recording, and exposing the vital statistics of your application.
The Significance of Metrics in Reactive Apps
Reactive applications are designed to efficiently handle a large number of concurrent requests and data streams. With the constantly changing state and influx of asynchronous events, understanding your application's performance becomes an intricate task. Metrics play a pivotal role in providing insights into the internal workings of your application, enabling you to identify potential bottlenecks, optimize resource utilization, and troubleshoot issues effectively.
Introducing Dropwizard Metrics
Dropwizard Metrics is a powerful library that provides support for capturing various metrics, such as counts, rates, gauges, and histograms, while offering integrations with popular monitoring systems. Its lightweight and easy-to-use nature make it a preferred choice for monitoring the health and behavior of applications, including reactive ones. By incorporating Dropwizard Metrics, developers can gain valuable visibility into the inner workings of their applications and make informed decisions based on data-driven insights.
Setting Up Dropwizard Metrics
To integrate Dropwizard Metrics into a reactive Java application, you need to include the dropwizard-metrics
dependency in your project. You can do this by adding the following Maven dependency:
<dependency>
<groupId>io.dropwizard.metrics</groupId>
<artifactId>metrics-core</artifactId>
<version>4.1.13</version> <!-- Replace with the latest version -->
</dependency>
Once the dependency is added and the project is built, you can start leveraging the capabilities of Dropwizard Metrics within your application.
Recording Metrics
Dropwizard Metrics provides a straightforward way to record various types of metrics. Let's delve into some common types of metrics and how to record them within a reactive context.
Counters
Counters are used to measure the frequency of an event. They are particularly useful for tracking the number of incoming requests or occurrences of specific actions within an application. Here's how you can create and use a counter in Dropwizard Metrics:
import com.codahale.metrics.Counter;
Counter requestCounter = new Counter();
// Increment the counter for each incoming request
requestCounter.inc();
Gauges
Gauges provide a way to capture instantaneous values. They are suitable for metrics such as the current size of a queue or the memory utilization of a component. Let's create a gauge to monitor the CPU load:
import com.codahale.metrics.Gauge;
Gauge<Double> cpuLoadGauge = () -> getCpuLoad(); // Implement the method to retrieve CPU load
Timers
Timers are instrumental in tracking the duration of a particular operation or task. In a reactive application, measuring the latency of asynchronous calls or event processing can be crucial. Here's how you can use a timer to measure the execution time of a code block:
import com.codahale.metrics.Timer;
Timer requestTimer = new Timer();
Timer.Context timerContext = requestTimer.time();
try {
// Perform the operation to be measured
} finally {
timerContext.stop();
}
Meters
Meters are ideal for measuring the rate of an event over time. They can be employed to monitor the throughput of incoming requests or the frequency of specific operations. Let's create a meter to track the request rate:
import com.codahale.metrics.Meter;
Meter requestMeter = new Meter();
// Mark the occurrence of an event
requestMeter.mark();
Exposing Metrics
Once the metrics are being recorded, the next step is to expose them for monitoring and analysis. Dropwizard Metrics provides several reporting options and integrations with monitoring tools such as Graphite, Prometheus, and JMX. Let's explore how to set up a basic HTTP server for exposing metrics via an endpoint.
Dropwizard Metrics JMX
JMX (Java Management Extensions) provides a standard way of managing and monitoring Java applications. Dropwizard Metrics seamlessly integrates with JMX, allowing you to expose the recorded metrics through JMX. Adding JMX reporting is as simple as registering the JmxReporter:
import com.codahale.metrics.JmxReporter;
JmxReporter jmxReporter = JmxReporter.forRegistry(metricRegistry).build();
jmxReporter.start();
HTTP Server for Metrics
Dropwizard Metrics includes a built-in HTTP server for exposing the captured metrics. This feature proves to be immensely valuable when you need to integrate with monitoring systems or visualize the metrics. Setting up the HTTP server is straightforward:
import com.codahale.metrics.MetricRegistry;
import com.codahale.metrics.servlets.MetricsServlet;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletContextHandler;
import org.eclipse.jetty.servlet.ServletHolder;
public class MetricsServer {
public static void main(String[] args) throws Exception {
MetricRegistry metricRegistry = new MetricRegistry();
// Populate the metrics registry with the recorded metrics
Server server = new Server(8080);
ServletContextHandler context = new ServletContextHandler();
context.setContextPath("/");
server.setHandler(context);
context.addServlet(new ServletHolder(new MetricsServlet()), "/metrics");
server.start();
server.join();
}
}
The Closing Argument
In the realm of reactive applications, understanding the behavior and performance of your system is pivotal for delivering a robust and responsive user experience. Dropwizard Metrics equips Java developers with the necessary tools to capture, record, and expose the crucial statistics of their applications. By incorporating Dropwizard Metrics into your reactive app, you can gain valuable insights into its internal dynamics and steer towards proactive performance optimization.
Embracing the power of Dropwizard Metrics empowers developers to elevate the reliability and efficiency of their reactive Java applications, paving the way for seamless scalability and enhanced user satisfaction.
With the ability to effortlessly record metrics such as counters, gauges, timers, and meters, and leveraging the seamless integration options for metrics exposure, Dropwizard Metrics stands as a formidable ally in the pursuit of reactive apps success.
In conclusion, leveraging the capabilities of Dropwizard Metrics propels your reactive Java application towards a realm of informed decision-making, proactive issue resolution, and optimized resource utilization, thereby laying the foundation for unparalleled user experiences in the world of modern software development.