Overcoming Challenges in Diverse Computational Results
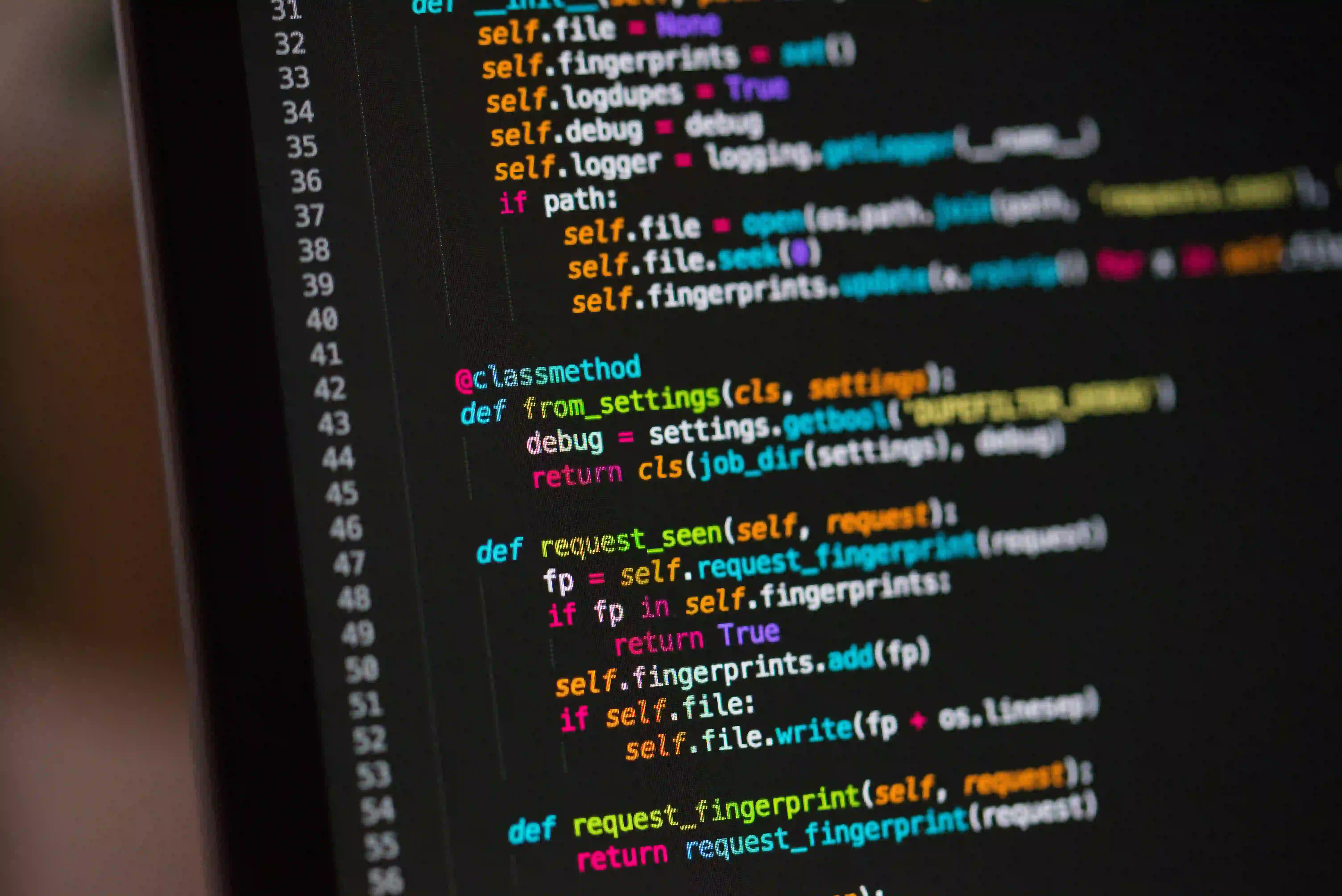
Overcoming Challenges in Diverse Computational Results
In the world of Java development, dealing with diverse computational results is a common challenge that developers often encounter. Whether it's processing complex algorithms, handling a large amount of data, or optimizing performance, the need to overcome these challenges is crucial for building robust and efficient applications.
In this article, we will explore some of the common challenges developers face when dealing with diverse computational results in Java, and discuss strategies and best practices to overcome them.
Understanding the Challenges
When working with diverse computational results, developers often encounter challenges related to performance, memory management, and complex data processing. These challenges can arise from various factors such as inefficient algorithms, suboptimal data structures, or lack of parallel processing.
1. Performance
Optimizing the performance of computational algorithms is crucial, especially when dealing with large datasets or complex computations. Inefficient algorithms can result in slow processing times and poor overall application performance.
2. Memory Management
Managing memory efficiently is vital when working with diverse computational results. Inadequate memory management can lead to memory leaks, excessive garbage collection, and degraded application performance.
3. Complex Data Processing
Processing diverse and complex data requires careful consideration of data structures, algorithms, and parallel processing techniques. Inadequate handling of complex data can lead to errors, inconsistencies, and suboptimal performance.
Strategies for Overcoming Challenges
To address the challenges associated with diverse computational results, developers can employ various strategies and best practices in their Java applications.
1. Algorithm Optimization
One of the most effective ways to improve the performance of computational algorithms is through algorithm optimization. This involves analyzing and refining the algorithms to make them more efficient and reduce computational complexity.
// Example of algorithm optimization
public int linearSearch(int[] arr, int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target) {
return i; // Found the target
}
}
return -1; // Target not found
}
In the above code snippet, the linear search algorithm is optimized for better performance by using a simple loop to iterate through the array and find the target element.
2. Memory Optimization
Efficient memory management is essential for handling diverse computational results. It's important to minimize memory consumption and reduce unnecessary object creation to avoid memory-related issues.
// Example of memory optimization using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Java");
}
String result = sb.toString();
In the above code snippet, using StringBuilder
instead of concatenating strings directly helps in optimizing memory usage by reducing the number of created string objects.
3. Parallel Processing
Utilizing parallel processing techniques can significantly improve the performance of complex data processing tasks. Java provides
concurrency utilities such as ExecutorService
and ForkJoinPool
to facilitate parallel processing.
// Example of parallel processing using ExecutorService
ExecutorService executor = Executors.newFixedThreadPool(4);
List<Future<Integer>> resultList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
Future<Integer> result = executor.submit(new SomeTask(i));
resultList.add(result);
}
// Process the results
for (Future<Integer> future : resultList) {
// Handle the computed result
}
executor.shutdown();
By utilizing parallel processing with ExecutorService
, the computational tasks can be efficiently distributed across multiple threads,
improving overall performance.
Closing Remarks
Dealing with diverse computational results in Java can be challenging, but by employing strategies such as algorithm optimization, memory management, and parallel processing, developers can overcome these challenges and build robust and efficient applications.
Understanding the complexities of computational algorithms, implementing efficient memory management techniques, and leveraging parallel processing capabilities are essential for addressing the challenges associated with diverse computational results.
By adopting these strategies and best practices, developers can ensure that their Java applications are capable of handling diverse computational tasks with optimal performance and reliability.
In conclusion, overcoming challenges in diverse computational results requires a combination of analytical thinking, technical expertise, and a thorough understanding of Java's capabilities for handling complex computations.
For more in-depth knowledge on parallel processing in Java, check out the official Java Concurrency Tutorial. Additionally, to dig deeper into memory management techniques, explore the Java Platform's Memory Management documentation.
Keep exploring, keep optimizing, and keep coding efficiently with Java!