Maximizing Efficiency with Story Points
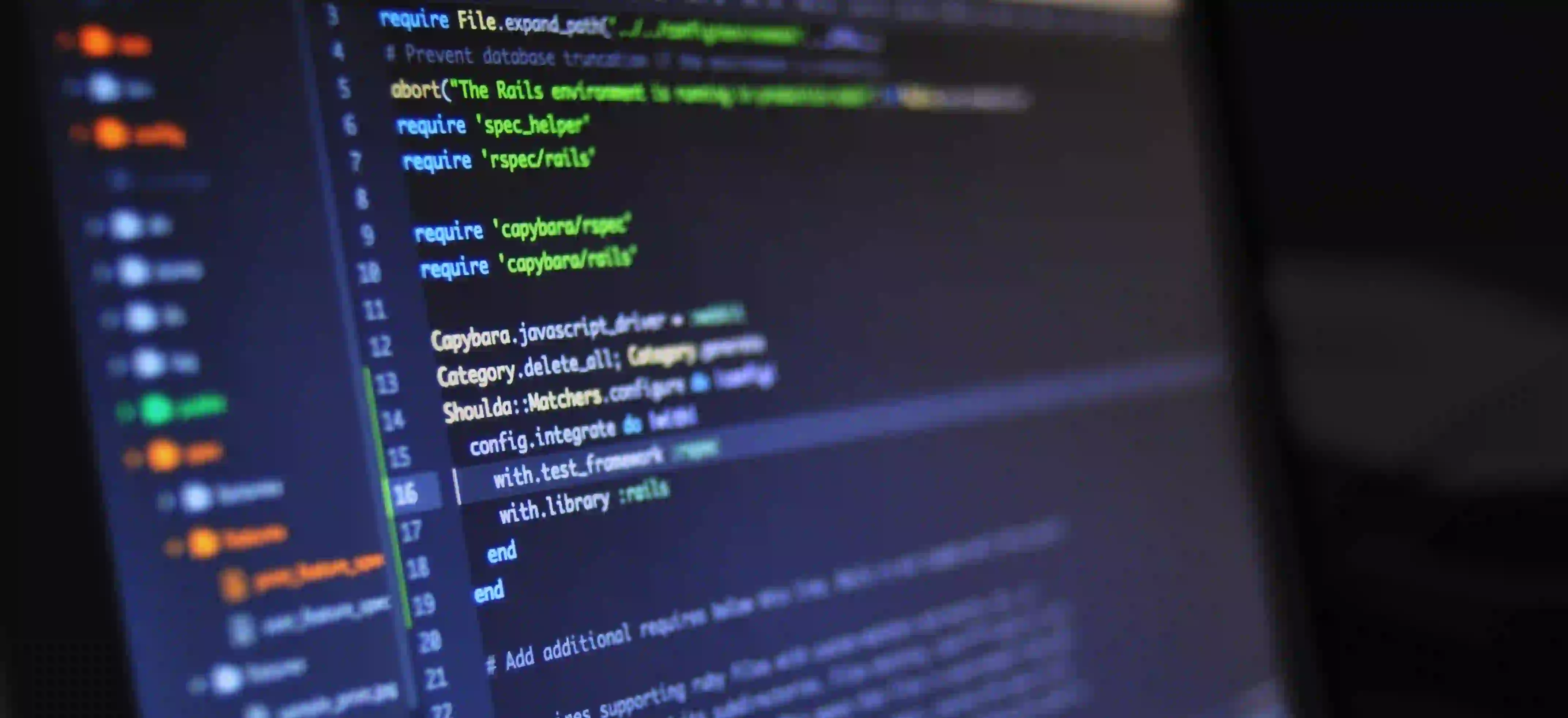
Maximizing Efficiency with Story Points
As a fundamental component of Agile development, Story Points help development teams estimate the effort and complexity of user stories. By using Story Points effectively, teams can maximize efficiency, improve planning accuracy, and enhance overall productivity. In this post, we will explore the concept of Story Points in Java development, discuss best practices for their implementation, and delve into how they can contribute to streamlined project delivery.
Understanding Story Points
Story Points are a unit of measure used in Agile methodologies to estimate the amount of effort required to implement a user story. Unlike traditional time-based estimation, Story Points focus on the relative complexity and the overall effort involved in completing a particular task. This approach enables teams to make more accurate forecasts and minimize the impact of individual variances in team members' capabilities and skill sets.
In Java development, Story Points are especially valuable when working on iterative and incremental projects, as they provide a consistent and adaptable framework for estimation and planning. By assigning Story Points to user stories, teams can prioritize tasks effectively and create a shared understanding of the work involved.
Benefits of Story Points in Java Development
1. Flexibility in Estimation
Story Points allow for a more flexible and versatile estimation process. Instead of fixating on precise time-based estimates, teams can focus on the relative size and complexity of tasks. In Java development, this approach accommodates the wide range of factors that can influence the time required to complete a user story, such as technical debt, unfamiliar libraries, or unexpected challenges with third-party integrations.
2. Improved Planning Accuracy
By leveraging Story Points, Java development teams can achieve greater accuracy in release and sprint planning. The use of Story Points encourages a holistic view of the work involved, leading to more reliable projections of when specific features or functionalities will be ready for deployment. This level of planning accuracy fosters transparency and predictability within the development process.
3. Team Collaboration and Empowerment
Story Points promote collaboration and empower development teams to collectively assess and estimate the work at hand. When using Story Points, Java developers can engage in in-depth discussions about the complexity of user stories, drawing upon their collective expertise to arrive at consensual estimations. This collaborative approach fosters a sense of ownership and accountability within the team.
4. Adaptability and Continual Improvement
The use of Story Points in Java development encourages adaptability and continual improvement. As teams gain more experience in estimating Story Points, they can refine their approach and calibrate their understanding of task complexity. This iterative process contributes to ongoing enhancement of estimation practices and overall efficiency.
Best Practices for Utilizing Story Points in Java Development
1. Establish a Baseline
When introducing Story Points to a Java development team, it is crucial to establish a baseline by collectively assigning Story Points to a set of reference user stories. This exercise serves as the foundation for subsequent estimations, providing a common frame of reference for the team.
2. Focus on Relative Sizing
Emphasize the comparative nature of Story Points when estimating user stories in Java development. Encourage team members to consider the relative complexity of tasks rather than attempting to quantify them in absolute terms. This shift in perspective fosters a more nuanced and accurate estimation process.
3. Leverage Planning Poker
Utilize the planning poker technique during estimation sessions to harness the collective wisdom of the development team. Through open discussion and comparative assessment, team members can converge on unified Story Point estimates for user stories, leveraging diverse perspectives to arrive at well-informed decisions.
4. Incorporate Historical Data
Draw insights from historical data on completed user stories to refine Story Point estimations in Java development. Analyzing past performance and outcomes enables teams to adjust their estimation approach, identify trends, and enhance the accuracy of future estimations.
5. Regularly Review and Refine
Regularly review the Story Point assignments for completed user stories and use these insights to refine future estimations. This practice facilitates a continual improvement cycle, guiding the team towards increasingly precise and insightful estimation outcomes in Java development.
Code Example: Implementing Story Points in Java
public class UserStory {
private String title;
private int storyPoints;
public UserStory(String title, int storyPoints) {
this.title = title;
this.storyPoints = storyPoints;
}
// Getters and setters for 'title' and 'storyPoints'
public static void main(String[] args) {
UserStory feature1 = new UserStory("Implement user authentication", 5);
UserStory feature2 = new UserStory("Integrate payment gateway", 8);
// Utilizing Story Points for prioritization and planning
// ...
}
}
In this Java code example, the 'UserStory' class demonstrates the implementation of Story Points within a development context. Each 'UserStory' object includes a title and an assigned number of Story Points, enabling teams to prioritize and plan their work effectively.
By integrating Story Points into the Java development process, teams can leverage this approach to streamline their workflow, enhance planning accuracy, and foster a culture of collaborative estimation and continual improvement.
Closing Remarks
Story Points serve as a powerful tool for enhancing efficiency and optimizing planning in Java development. By embracing the relative complexity-based estimation approach facilitated by Story Points, teams can achieve greater accuracy, foster collaboration, and adapt to evolving project dynamics more effectively.
Implementing Story Points in Java development empowers teams to transcend the limitations of traditional time-based estimation, leading to improved planning accuracy and a more cohesive, forward-looking development process. Embracing best practices and leveraging the flexibility of Story Points can propel Java development teams towards enhanced productivity and success.
Incorporating Story Points into the fabric of Java development not only streamlines the planning process but also cultivates a shared understanding of the intricacies of the work at hand, bolstering the team's ability to deliver high-quality solutions in a dynamic and competitive landscape.