Conquering Complexities: A Beginner's Guide to Dynamic Programming
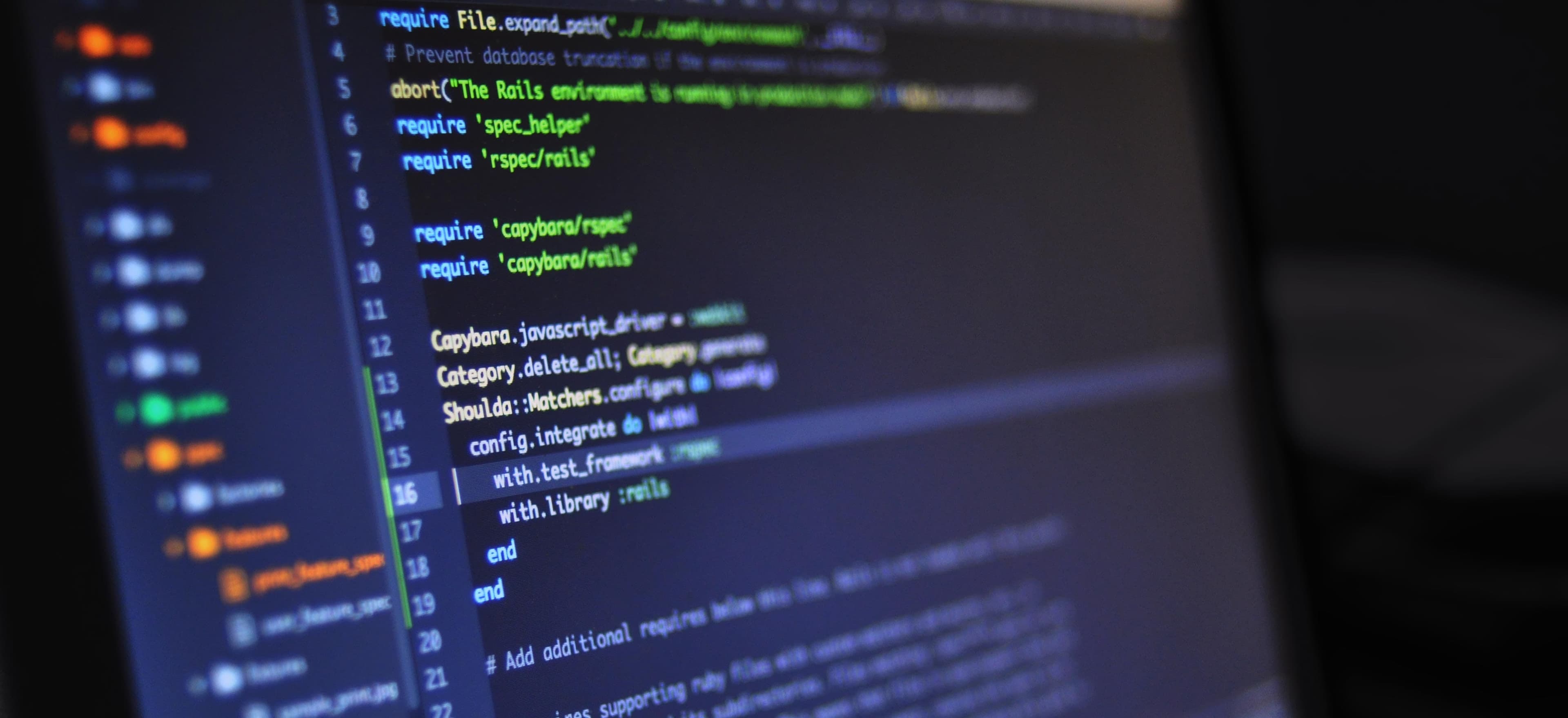
- Published on
Conquering Complexities: A Beginner's Guide to Dynamic Programming
Dynamic programming is a fundamental concept in computer science and a crucial tool in a programmer's arsenal. It is a technique used to solve complex problems by breaking them down into simpler subproblems. In this blog post, we will delve into the world of dynamic programming, exploring its core principles, understanding its application, and implementing it in Java. Whether you are a novice programmer or a seasoned developer looking to refresh your knowledge, this guide will walk you through the essential aspects of dynamic programming in Java.
Understanding Dynamic Programming
At its core, dynamic programming is all about simplifying complex problems by breaking them down into smaller, more manageable subproblems. Unlike its name suggests, dynamic programming has little to do with the "dynamic" aspect of programming; instead, it revolves around breaking down problems into simpler recursive subproblems. This approach allows us to solve each subproblem only once and store its solution, thereby avoiding redundant computations and significantly improving the efficiency of our programs.
Overlapping Subproblems and Optimal Substructure
Two key elements characterize problems that can be solved using dynamic programming: overlapping subproblems and optimal substructure.
Overlapping Subproblems
Overlapping subproblems occur when a problem can be broken down into smaller subproblems, which are reused multiple times to derive the solution. By storing the solutions to these subproblems, we can avoid recalculating them, leading to a more efficient solution.
Optimal Substructure
A problem exhibits optimal substructure if an optimal solution can be constructed from optimal solutions of its subproblems. This property enables us to recursively build our solution, ensuring that we are always moving towards the best possible outcome.
The Essence of Memoization
Memoization, a core concept in dynamic programming, involves storing the results of expensive function calls and reusing them when the same inputs occur again. In Java, memoization can be achieved through various means, such as using arrays or maps to store the results of subproblems. By memoizing the solutions to overlapping subproblems, we can effectively minimize redundant computations and enhance the efficiency of our dynamic programming algorithms.
Implementing Dynamic Programming in Java
Let's illustrate the concepts of dynamic programming through a classic example: the Fibonacci sequence. The Fibonacci sequence is a series of numbers in which each number (after the first two) is the sum of the two preceding ones. We can implement a dynamic programming solution to efficiently compute the Fibonacci sequence in Java.
Tabulation vs. Memoization
There are two primary approaches to dynamic programming: tabulation and memoization. Tabulation involves solving the problem "bottom-up," starting with the simplest subproblems and iteratively building up to the larger problem. On the other hand, memoization approaches the problem "top-down," starting with the original problem and breaking it down into smaller subproblems.
Let's consider the tabulation approach for computing the Fibonacci sequence in Java:
public class Fibonacci {
public int fibonacci(int n) {
int[] dp = new int[n + 1];
dp[0] = 0;
dp[1] = 1;
for (int i = 2; i <= n; i++) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
}
In this implementation, we use an array dp
to store the solutions to subproblems, starting from the base cases of the Fibonacci sequence and iterating up to n
. By iteratively computing and storing the results, we avoid redundant computations and achieve an efficient solution.
On the other hand, let's explore the memoization approach for the same problem:
import java.util.HashMap;
import java.util.Map;
public class Fibonacci {
private Map<Integer, Integer> memo = new HashMap<>();
public int fibonacci(int n) {
if (memo.containsKey(n)) {
return memo.get(n);
}
if (n <= 1) {
return n;
}
int result = fibonacci(n - 1) + fibonacci(n - 2);
memo.put(n, result);
return result;
}
}
In this memoization-based implementation, we utilize a map memo
to store the results of subproblems. Before computing a subproblem, we check if its solution is already present in the memoization table, and if so, we retrieve it directly. This prevents redundant computations and optimizes the recursive approach for computing the Fibonacci sequence.
Advantages and Considerations
While both tabulation and memoization are effective approaches to dynamic programming, each has its strengths and considerations. Tabulation is often more straightforward to implement and is well-suited for problems with clear optimal substructure and overlapping subproblems. Conversely, memoization excels in handling recursive solutions and is particularly useful for problems with complex tree-like structures.
Key Takeaways
Dynamic programming is a powerful technique that enables us to solve complex problems by decomposing them into simpler subproblems. By leveraging concepts such as memoization, tabulation, and the identification of optimal substructure and overlapping subproblems, we can design efficient and scalable solutions to a wide range of computational challenges.
In Java, dynamic programming can be implemented using various strategies, including tabulation and memoization. Understanding the principles and techniques of dynamic programming equips developers with the tools to tackle complex problems while optimizing the performance of their programs.
As you continue your journey in mastering dynamic programming, consider exploring the concept further by solving classic dynamic programming problems and familiarizing yourself with advanced algorithms and data structures. Embracing dynamic programming opens doors to elegant solutions and a deeper understanding of algorithmic problem-solving.
Dynamic programming, with its blend of recursion, optimization, and efficient problem-solving, stands as an indispensable skill for any programmer seeking to conquer complexities and unravel the beauty of algorithmic intricacies.
So, dive into the world of dynamic programming, and let the intricacies of code come to life as you conquer complexities with the power of Java.
Remember, the journey of a thousand lines of code begins with a single algorithmic step!
(To read more on advanced dynamic programming techniques, delve into GeeksforGeeks for comprehensive resources.)
Checkout our other articles