Mastering SQS & Spring: Solving Message Duplication
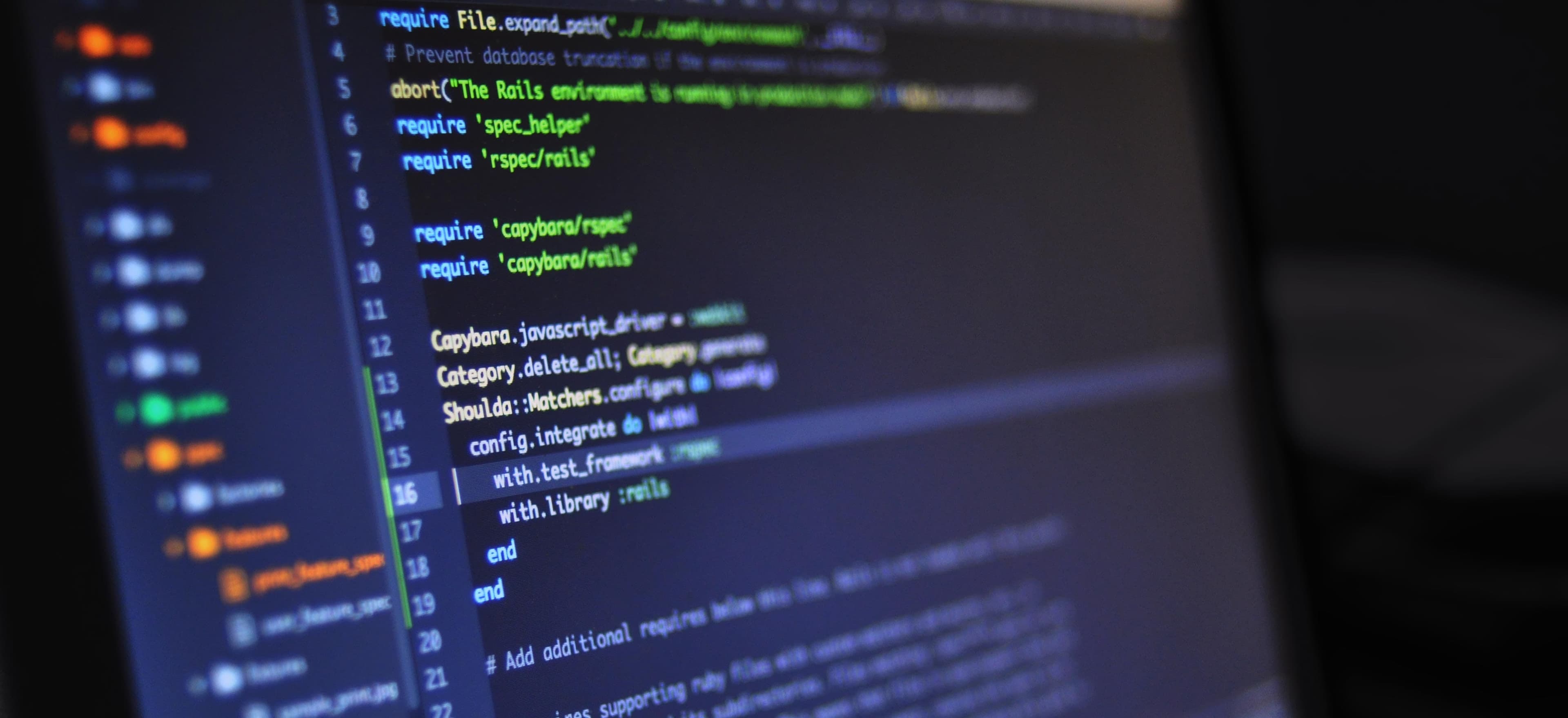
- Published on
Mastering SQS & Spring: Solving Message Duplication
In a distributed system, message queues play a critical role in ensuring seamless communication between various microservices. Amazon Simple Queue Service (SQS) is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications.
When integrating SQS with Spring, it's essential to address the issue of message duplication. In this blog post, we'll explore the common causes of message duplication in SQS and how to solve this problem effectively using Spring.
Understanding Message Duplication in SQS
Message duplication can occur in SQS due to various factors, such as network glitches, client timeouts, and retries by the SQS client. When an application publishes a message to an SQS queue, the message may get delivered to a consumer multiple times, leading to unintended consequences and potential data corruption.
Implementing Idempotent Consumption
One of the fundamental techniques to combat message duplication is to ensure idempotent message consumption. Idempotent consumption means that processing a message multiple times has the same effect as processing it once.
Leveraging Deduplication IDs
SQS provides a feature called content-based deduplication to avoid the processing of the same message multiple times within a specified time frame. When enabling content-based deduplication, SQS uses the MessageDeduplicationId
attribute to identify and eliminate duplicate messages.
Configuring Deduplication in Spring
Let's dive into a hands-on example of how to configure content-based deduplication in a Spring application using aws-java-sdk
and spring-cloud-aws
. First, add the necessary dependencies to your pom.xml
:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-sqs</artifactId>
<version>1.11.986</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-aws</artifactId>
<version>2.2.4.RELEASE</version>
</dependency>
Now, configure the SQS client in your Spring application:
@Configuration
public class SqsConfig {
@Value("${cloud.aws.region.static}")
private String region;
@Bean
public AmazonSQSAsync amazonSQS() {
return AmazonSQSAsyncClientBuilder.standard()
.withRegion(Regions.fromName(region))
.build();
}
}
In this configuration, we're creating a bean for AmazonSQSAsync
that connects to SQS using the specified region.
Handling Message Processing Errors
Another aspect to consider is how to handle message processing errors to prevent message duplication. When an error occurs during message processing, it's crucial to ensure that the message doesn't get lost and is processed again. This is where employing a dead-letter queue (DLQ) can be immensely beneficial.
Setting Up a Dead-Letter Queue in SQS
A dead-letter queue is a special type of queue where messages are sent if they cannot be processed successfully after a certain number of attempts. This allows you to isolate problematic messages for further analysis and debugging.
Configuring DLQ in Spring
Let's see how to configure a dead-letter queue for an SQS queue in a Spring application:
@Bean
public QueueMessageHandlerFactory queueMessageHandlerFactory(AmazonSQSAsync amazonSqs) {
QueueMessageHandlerFactory factory = new QueueMessageHandlerFactory();
ErrorHandler errorHandler = new SeekToCurrentErrorHandler(new DeadLetterPublishingRecoverer(amazonSqs, DLQ_ARN));
factory.setAmazonSqs(amazonSqs);
factory.setArgumentResolvers(Collections.singletonList(new PayloadArgumentResolver()));
factory.setErrorHandler(errorHandler);
return factory;
}
In this configuration, we're setting up a QueueMessageHandlerFactory
with an error handler that utilizes a dead-letter publishing recoverer. This ensures that any messages failing processing are redirected to the specified dead-letter queue.
Bringing It All Together
In this article, we've explored the causes of message duplication in SQS and discussed effective strategies for solving this issue in a Spring application. By ensuring idempotent consumption, leveraging content-based deduplication, and setting up a dead-letter queue, you can significantly mitigate the impact of message duplication and build a robust messaging system.
Combating message duplication is crucial for maintaining data integrity and system reliability in microservices architectures. With the combination of AWS SQS and Spring, you can address this challenge effectively and streamline your message processing workflows.
Remember, mastering SQS and Spring is an ongoing journey, and continually refining your approach to handling message duplication will contribute to the resilience and stability of your distributed systems.
To deepen your understanding of SQS, Spring, and related concepts, consider exploring the official documentation and case studies provided by AWS and Spring.
Now that you have a strong understanding of addressing message duplication in SQS using Spring, it's time to apply these practices to your own projects and elevate the efficiency and reliability of your message-driven applications.
Checkout our other articles