Maximizing Performance with Hazelcast Distributed Execution
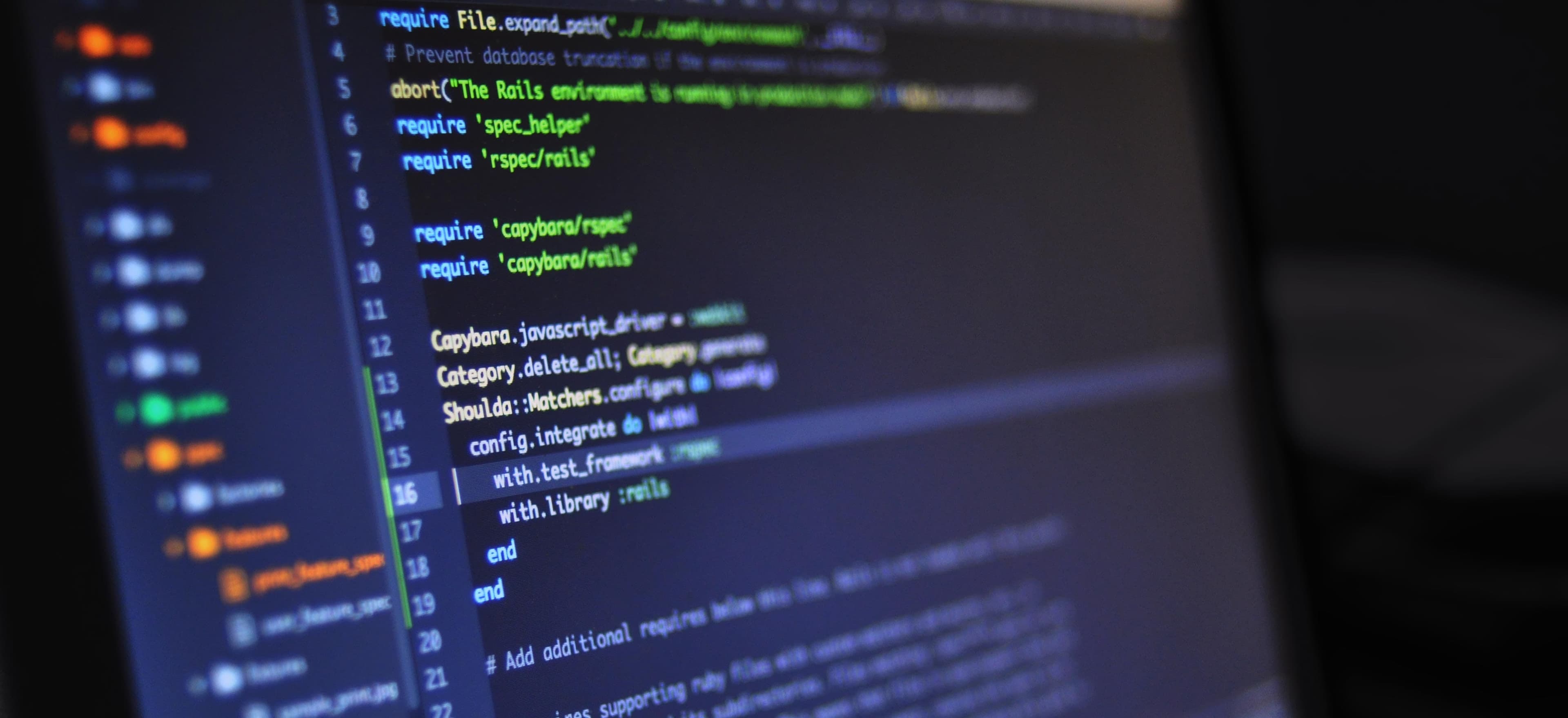
- Published on
Getting Started
In a world where data is growing at an exponential rate, processing large datasets efficiently is crucial for the success of any application. Java developers often face the challenge of optimizing their code to achieve maximum performance, especially when dealing with big data. Fortunately, there are tools like Hazelcast that provide a distributed computing platform to help tackle these challenges. In this article, we will explore how to leverage Hazelcast's distributed execution to maximize the performance of your Java applications.
What is Hazelcast?
Hazelcast is an in-memory data grid platform that provides distributed data structures and distributed computing capabilities. It allows you to distribute data and computation across multiple nodes, which can lead to significant performance improvements, especially when dealing with large datasets.
One of the key features of Hazelcast is its support for distributed execution, which enables you to distribute computational tasks across the nodes in a Hazelcast cluster. This can be immensely beneficial for parallelizing computations and improving the overall performance of your applications.
Setting Up Hazelcast
Before we can dive into distributed execution, we need to set up a Hazelcast cluster. Setting up a basic Hazelcast cluster is quite straightforward. You just need to add the Hazelcast dependency to your project and configure the cluster members.
Config config = new Config();
NetworkConfig networkConfig = config.getNetworkConfig();
networkConfig.setPortAutoIncrement(true);
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance(config);
In this example, we create a Hazelcast Config
object, which represents the configuration of our Hazelcast cluster. We then enable the PortAutoIncrement
feature, which allows the cluster to assign ports automatically, and finally, we create a new instance of Hazelcast using this configuration.
Distributed Execution with Hazelcast
Once we have our Hazelcast cluster set up, we can start leveraging its distributed execution capabilities. Hazelcast provides an ExecutorService
that allows you to distribute tasks across the nodes in the cluster.
Let's consider a scenario where we need to perform a computationally intensive task on a large collection of data. Traditionally, we might iterate through the collection and process each element sequentially, which can be time-consuming, especially for large datasets. With Hazelcast's distributed execution, we can parallelize this task and distribute the workload across the cluster, significantly improving the processing time.
ExecutorService executorService = hazelcastInstance.getExecutorService("executor");
Map<Integer, Integer> data = ... // Initialize data
List<Future<Integer>> futures = new ArrayList<>();
for (Integer value : data.values()) {
Callable<Integer> task = () -> performIntensiveTask(value);
futures.add(executorService.submit(task));
}
int totalResult = 0;
for (Future<Integer> future : futures) {
totalResult += future.get();
}
In this example, we obtain an ExecutorService
from the Hazelcast instance and initialize a collection of data. We then iterate through the data, creating a Callable
task for each element, and submit these tasks to the ExecutorService
. Finally, we wait for the results and combine them to obtain the final result.
By using the distributed execution capabilities of Hazelcast, we are able to parallelize the intensive task and spread the workload across the nodes in the cluster. This can lead to significant improvements in processing time, especially when running on a cluster with multiple nodes.
Monitoring and Managing Distributed Execution
While distributed execution can greatly enhance the performance of your application, it's important to monitor and manage the distributed tasks to ensure optimal resource utilization and prevent performance bottlenecks.
Hazelcast Management Center is a powerful tool that provides real-time monitoring and management of your Hazelcast clusters. It allows you to visualize cluster metrics, track distributed task executions, and identify any potential performance issues.
By monitoring the distributed tasks, you can gain insights into their resource consumption, execution times, and overall performance impact on the cluster. This visibility enables you to optimize the distribution of tasks, tune the cluster configuration, and make informed decisions to maximize the performance of your distributed executions.
The Bottom Line
In conclusion, Hazelcast's distributed execution capabilities provide a powerful way to maximize the performance of your Java applications, especially when dealing with computationally intensive tasks and large datasets. By parallelizing tasks and distributing the workload across a Hazelcast cluster, you can achieve significant improvements in processing time and overall application performance.
When leveraging Hazelcast's distributed execution, be sure to monitor and manage the distributed tasks using tools like Hazelcast Management Center to ensure optimal resource utilization and performance enhancement.
To dive deeper into Hazelcast's distributed execution and explore more advanced features, check out the official Hazelcast Documentation.
Maximizing performance with Hazelcast distributed execution is not only about writing efficient code but also about optimizing resource utilization and making informed decisions to reap the full benefits of distributed computing. With Hazelcast, you have a powerful tool at your disposal to tackle the challenges of big data and maximize the performance of your Java applications.