Unlayering the Truth: Why Layered Architecture Fails
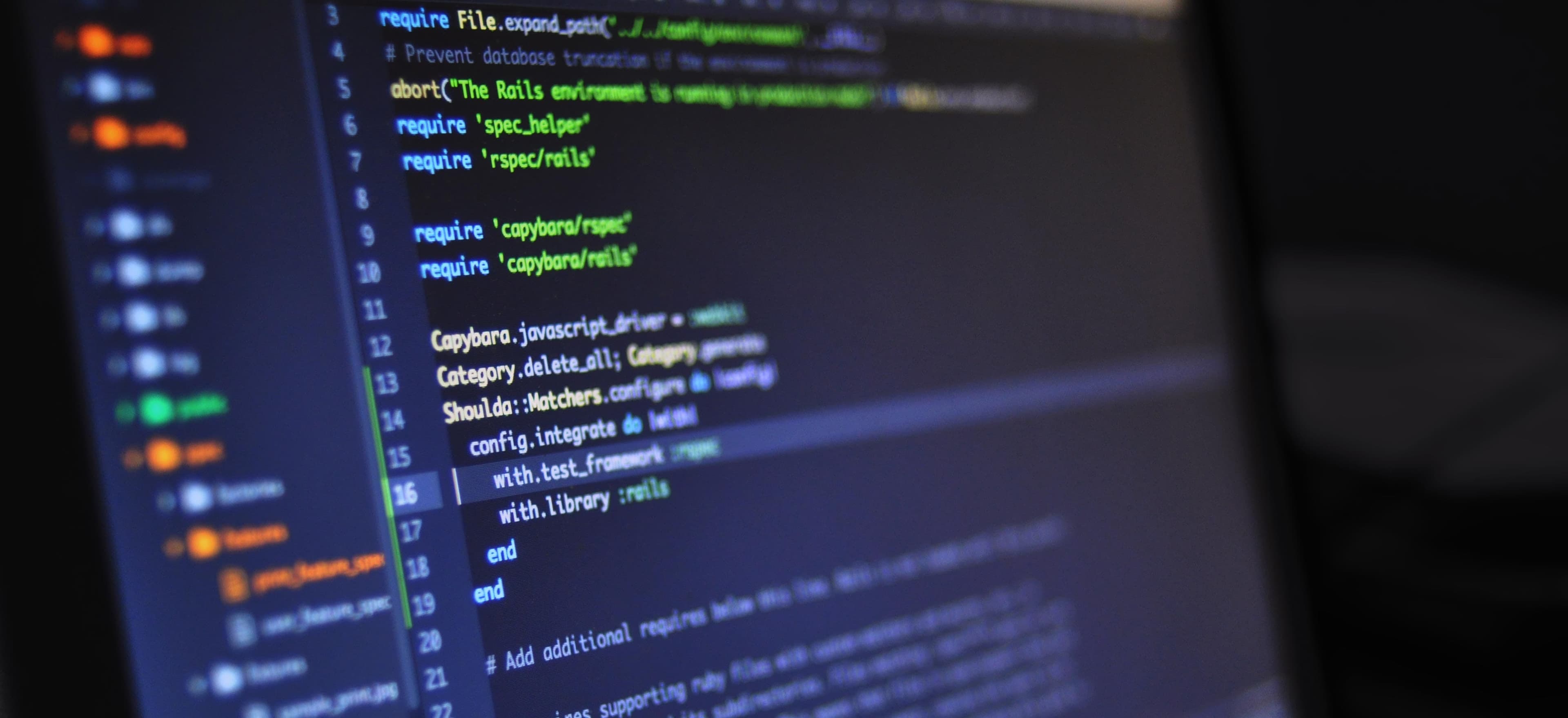
- Published on
Unlayering the Truth: Why Layered Architecture Fails
When it comes to designing and developing robust, maintainable, and scalable software applications, architecture plays a pivotal role. The choice of architecture can greatly impact the overall success of a software project. In the context of Java development, the debate around the effectiveness of layered architecture has been ongoing. While layered architecture has long been touted as a best practice for structuring applications, it's crucial to unlayer the truth and understand why this approach can fall short.
Understanding Layered Architecture
Layered architecture, also known as multitier architecture, is a widely adopted design pattern in which the components of an application are organized into horizontal layers. These typically include presentation, application, domain, and data access layers, each with its specific set of responsibilities. The primary goal is to enforce a separation of concerns and promote modularity, making the codebase more maintainable and scalable.
public class UserController {
private final UserService userService;
public UserController() {
this.userService = new UserService();
}
public User createUser(String username, String email) {
// Input validation and business logic
return userService.createUser(username, email);
}
}
In the above snippet, we can see the UserController invoking the UserService, adhering to the layered architecture principles.
The Pitfalls of Layered Architecture
Tight Coupling
One of the major drawbacks of layered architecture is the potential for tight coupling between layers. As the layers depend on each other, any change in one layer can have cascading effects on other layers, leading to a lack of flexibility and increased complexity.
Inefficient Communication
In a layered architecture, communication between layers often involves passing data through multiple abstraction boundaries. This can lead to performance overhead, especially in scenarios where data transformation and mapping are prevalent.
Limited Flexibility
Layered architecture can hinder flexibility, making it challenging to accommodate changes that do not align with the predefined layer boundaries. This rigidity can impede the evolution of the application, especially in agile environments where adaptability is crucial.
Testing Challenges
Testing components in isolation within a layered architecture can be cumbersome. Mocking dependencies for unit testing becomes intricate due to the interconnected nature of the layers, impacting the overall testability of the system.
Beyond Layered Architecture: Embracing a Modular Approach
In response to the limitations of layered architecture, an alternative approach that has gained traction in the Java ecosystem is modular architecture. Modular architecture emphasizes the creation of self-contained, reusable modules that encapsulate both data and behavior, fostering loose coupling and high cohesion.
public interface UserService {
User createUser(String username, String email);
}
public class DefaultUserService implements UserService {
@Override
public User createUser(String username, String email) {
// Business logic
return new User(username, email);
}
}
In the revised code snippet, we introduce an interface for the UserService, promoting abstraction and enabling flexibility through dependency injection and inversion of control.
Advantages of Modular Architecture
-
Loose Coupling: Modules are decoupled from one another, reducing interdependencies and mitigating the ripple effects of changes.
-
High Cohesion: Modules encapsulate related functionality, promoting better maintainability and reusability.
-
Testability: With clear module boundaries, unit testing becomes more straightforward, as individual modules can be tested in isolation.
-
Flexibility: Modular architecture allows for more fluid adaptation to evolving requirements, facilitating agility in development.
Evolution of Architecture: Microservices and Beyond
As software development paradigms continue to advance, the traditional notions of layered architecture are being challenged by newer concepts such as microservices architecture. Microservices advocate for building applications as a collection of loosely coupled, independently deployable services, each responsible for a specific business capability.
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody UserRequest userRequest) {
// Input validation and delegation to UserService
User user = userService.createUser(userRequest);
return ResponseEntity.ok(user);
}
}
In the above code snippet, the UserController is designed as a part of a microservice, leveraging dependency injection for the UserService.
The Microservices Paradigm
-
Autonomous Services: Microservices promote autonomy, allowing teams to develop and deploy services independently, fostering agility and innovation.
-
Resilience and Scalability: By being distributed and scalable by nature, microservices architecture enables systems to handle varying workloads and recover from failures more effectively.
-
Technological Heterogeneity: Each microservice can be implemented using the most suitable technology stack for its specific functionality, providing flexibility in technology choices.
In Conclusion, Here is What Matters
While the layered architecture has been a staple in software development, its shortcomings have become increasingly evident in today’s dynamic and demanding development landscape. Embracing modular architecture and exploring microservices can offer compelling alternatives that align with the evolving needs of modern software systems. By reassessing architectural paradigms and staying abreast of industry trends, Java developers can navigate the complexities of software design more adeptly, fostering resilient, scalable, and maintainable applications.
In conclusion, the unlayering of the truth behind the limitations of layered architecture underscores the imperative for continual evolution and adaptation in software architecture, ultimately shaping the future of Java development.
For further insight into software architecture, check out the official Java documentation and Spring Framework for comprehensive resources.