Mastering Java EE Interceptors: Overcoming Common Pitfalls
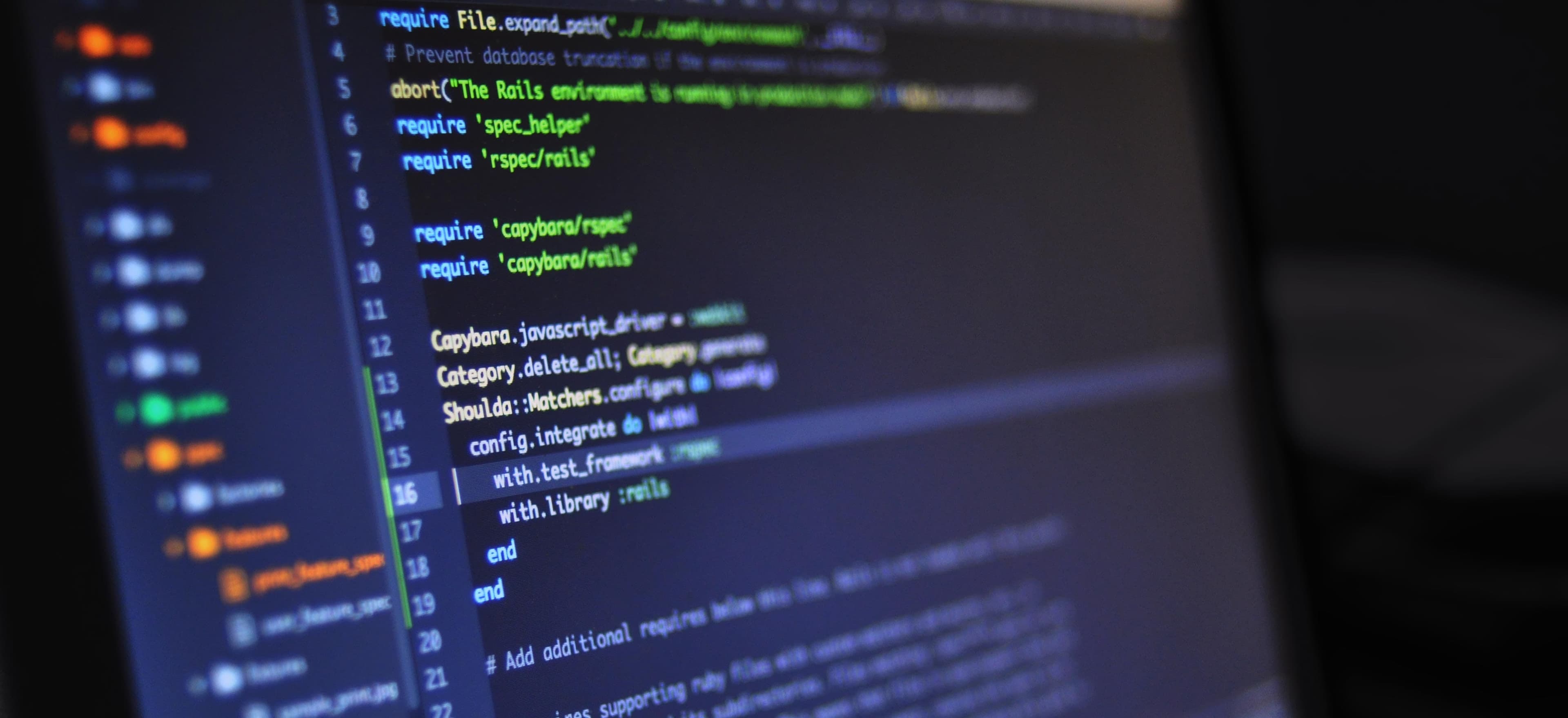
- Published on
Mastering Java EE Interceptors: Overcoming Common Pitfalls
Java EE (Jakarta EE) has long been revered for its robust capabilities, especially in enterprise-level application development. One of its lesser-discussed but incredibly powerful features is Interceptors. They provide a way to add additional behavior to existing components, making your code cleaner and more maintainable. However, understanding how to leverage this feature effectively can be challenging. In this post, we will explore common pitfalls when working with Java EE Interceptors and offer insights on how to avoid them.
What Are Java EE Interceptors?
Interceptors allow developers to define behaviors that can be dynamically applied to different components, such as EJBs (Enterprise JavaBeans) or managed beans. They work by intercepting method calls or lifecycle events and can be used for logging, security checks, transaction management, and more.
Why Use Interceptors?
- Separation of Concerns: Interceptors help in separating concerns by encapsulating different behaviors outside of the business logic code.
- Code Reusability: Interceptor logic can be reused across multiple classes.
- Centralized Control: Common behaviors like logging or validation can be managed in one place, enhancing maintainability.
Common Pitfalls When Using Java EE Interceptors
While the benefits of using Java EE Interceptors are clear, developers often encounter several pitfalls. Let's dive into some of these challenges and discuss best practices to overcome them.
1. Misunderstanding the Lifecycle of Interceptors
One of the most common pitfalls is misunderstanding how and when interceptors are executed. Interceptor methods are called according to the lifecycle of the bean they are attached to.
Solution:
Make sure to familiarize yourself with the context in which your interceptors are running. For instance, interceptors can be applied to:
- EJB Lifecycle
- Method invocation
- Lifecycle callbacks
Here's a simple interceptor example that logs method calls:
import javax.interceptor.AroundInvoke;
import javax.interceptor.Interceptor;
import javax.interceptor.InvocationContext;
@Interceptor
public class LoggingInterceptor {
@AroundInvoke
public Object logMethodEntry(InvocationContext ctx) throws Exception {
System.out.println("Entering method: " + ctx.getMethod().getName());
try {
return ctx.proceed();
} finally {
System.out.println("Exiting method: " + ctx.getMethod().getName());
}
}
}
In this code snippet, the logMethodEntry
method intercepts calls to methods of beans to log entry and exit points. Understanding the ctx.proceed()
function is critical, as this allows the original method execution to continue (the "proceed" is a must; otherwise, the method won't execute).
2. Not Handling Exceptions Properly
Another frequent issue arises when exceptions are not appropriately handled in interceptors. Neglecting exception handling can lead to unexpected application behavior.
Solution:
Interceptors should always include error handling. In the previous example, you can add a try-catch block to deal with exceptions gracefully. Here’s a modified version of our interceptor:
@AroundInvoke
public Object logMethodEntry(InvocationContext ctx) throws Exception {
System.out.println("Entering method: " + ctx.getMethod().getName());
try {
return ctx.proceed();
} catch (Exception e) {
System.err.println("Exception in method: " + ctx.getMethod().getName() + " - " + e.getMessage());
throw e; // Rethrow to preserve exception
} finally {
System.out.println("Exiting method: " + ctx.getMethod().getName());
}
}
This approach ensures that if an exception occurs, it's logged, but still appropriately thrown to prevent hiding issues.
3. Overusing Interceptors
It's easy to become overly reliant on interceptors to add behavior to all your beans. However, interceptors should be used judiciously, as excessive usage can lead to code that is difficult to follow.
Solution:
Use interceptors for cross-cutting concerns—areas of functionality that are commonly needed across multiple points in your application, like logging, security, or auditing. Avoid using interceptors for business logic.
4. Wrongly Applying Interceptor Bindings
Interceptor bindings can sometimes lead to unexpected behavior if they are misapplied. Perhaps you meant to apply an interceptor at the class level but mistakenly applied it at the method level, or vice versa.
Solution:
Explicitly define the scope of your interceptors. Here’s how you can specify it at the class level:
import javax.ejb.Stateless;
import javax.interceptor.Interceptors;
@Stateless
@Interceptors(LoggingInterceptor.class)
public class UserService {
public void createUser() {
// Logic to create user
}
}
When analyzing your usage of interceptors, make sure to double-check where and how they are applied. Refer to the official Jakarta EE documentation for guidance on best practices and nuances.
5. Missing @Interceptors Inheritance
Java EE supports interceptor inheritance, but developers often overlook this functionality. If a superclass has interceptors, a subclass does not automatically inherit them.
Solution:
Make sure to annotate superclass methods with the @Interceptors
annotation, so subclasses automatically inherit the intended behavior.
import javax.ejb.Stateless;
import javax.interceptor.Interceptors;
@Stateless
public class BaseService {
@Interceptors(LoggingInterceptor.class)
public void performOperation() {
// Logic for operations
}
}
@Stateless
public class ExtendedService extends BaseService {
// Inherits Logging Interceptor from BaseService
@Override
public void performOperation() {
super.performOperation();
}
}
In summary, interception is powerful, but failing to navigate the nuances of inheritance can lead to missed functionality.
The Last Word
Interceptors add a powerful layer of cross-cutting behavior to your Java EE applications, but they should be used wisely. By understanding the lifecycle events, exception handling, and the proper way to apply and inherit interceptors, you can prevent common pitfalls that might otherwise compromise your code quality.
For further reading on Java EE features, consider checking the official Jakarta EE documentation. It provides a wealth of information that can further enhance your understanding and usage of Interceptors and other features.
When used appropriately, interceptors can transform your enterprise applications, making them cleaner, more maintainable, and adhering to the principles of good design. Happy coding!