Microservices vs. SOA: Navigating the Architectural Maze
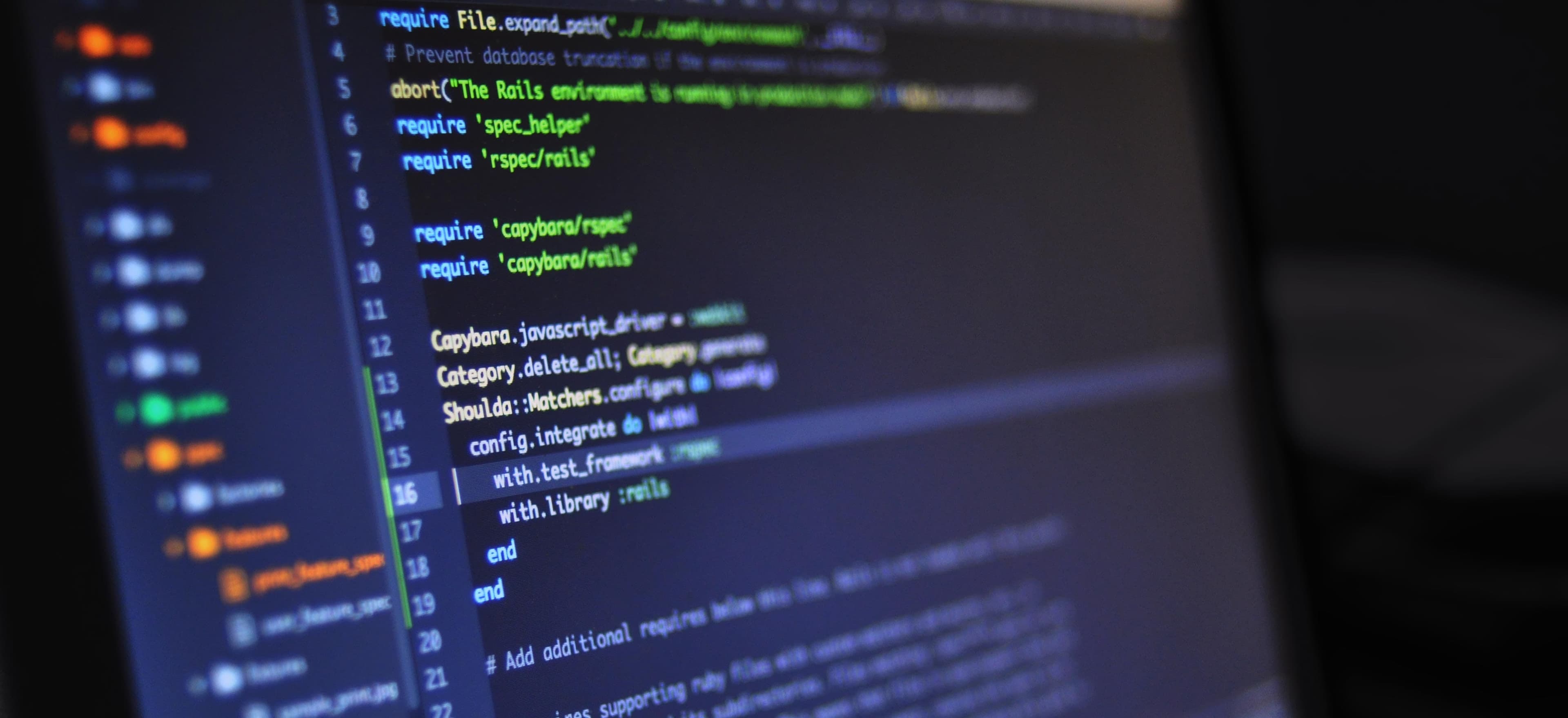
- Published on
Microservices vs. Service-Oriented Architecture (SOA): Navigating the Architectural Maze
In the realm of software architecture, two prominent paradigms have been vying for dominance in recent years: Microservices and Service-Oriented Architecture (SOA). Both have their strengths and weaknesses, and understanding the differences between the two is crucial for architects and developers looking to build scalable, resilient, and maintainable systems.
The Rise of Microservices
Microservices have gained immense popularity in the last decade, largely due to their ability to enable continuous delivery and deployment, foster a culture of DevOps, and scale applications more effectively. By breaking down large, monolithic systems into independently deployable services, microservices provide a range of benefits, such as fault isolation, improved agility, and better support for diverse technologies and data management systems.
Service-Oriented Architecture: A Legacy Approach
On the other hand, Service-Oriented Architecture (SOA) has been around for much longer, and it represents a more traditional, monolithic approach to building and integrating software systems. SOA emphasizes the development of loosely coupled services that can be orchestrated to create complex business processes. While it has been widely successful in many enterprise environments, it has also faced criticism for issues such as complexity, performance bottlenecks, and difficulty in implementing changes.
Understanding the Differences
To comprehend the distinctions between microservices and SOA, it’s important to dive into their key characteristics, integration strategies, and the impact they have on the development and operation of software systems.
Granularity of Services
One of the fundamental variations lies in the granularity of the services. Microservices advocates for very fine-grained services, each responsible for a specific business capability. SOA, meanwhile, often leads to coarser-grained services due to its focus on integrating large, enterprise-scale applications. Microservices promote small, single-purpose services that are aligned with specific business domains, allowing for more granular control over scaling and maintenance.
Autonomy and Decentralization
In the context of autonomy, microservices are designed to be fully autonomous, with each service owning its own data and business logic. This is a significant departure from the centralized approach of SOA, where a central ESB (Enterprise Service Bus) typically governs and manages the communication between services. The decentralized nature of microservices enables independent development and deployment, reducing the blast radius of changes and enhancing fault isolation.
Technology Heterogeneity
Microservices are built on the premise of technology heterogeneity, allowing each microservice to be developed using the most appropriate technology stack for its purpose. SOA, by contrast, often leans towards standardization to facilitate a more uniform governance structure and promote interoperability among services. While standardization can bring simplicity in some cases, it may also hinder innovation and the adoption of new, more suitable technologies.
Code-Level Comparison
Let’s take a look at a code-level comparison to illustrate the differences in the implementation of a simple “Order Management” service using both microservices and SOA principles.
Microservices Implementation
// Order Service
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping
public ResponseEntity<?> createOrder(@RequestBody OrderRequest orderRequest) {
// Validate and process the order
Order order = orderService.createOrder(orderRequest);
return ResponseEntity.ok(order);
}
// Other CRUD operations and business logic
}
In the microservices implementation, we have a standalone OrderService
responsible for handling the Order
domain. The service is exposed through its own RESTful API, following the single responsibility principle and allowing for independent scaling and deployment.
SOA Implementation
// Order Service Interface
public interface OrderService {
Order createOrder(OrderRequest orderRequest);
// Other service methods
}
// Order Service Implementation
@Service
public class OrderServiceImpl implements OrderService {
// Collaborations with other services or components
@Override
public Order createOrder(OrderRequest orderRequest) {
// Validate and process the order
// Collaboration with other services using ESB or orchestration
// Return the order
}
// Other service methods
}
In the SOA implementation, the OrderService
is defined as an interface, and its implementation is managed within a larger, centralized application context. The service methods are designed to integrate with other services or components via an ESB or orchestration, promoting a more coupled, holistic approach to service deployment and management.
A Final Look
In conclusion, both microservices and SOA offer distinct approaches to building and integrating software systems, each with its own set of trade-offs and benefits. While microservices provide agility, scalability, and technology diversity, SOA emphasizes standardization, legacy integration, and centralized governance.
Understanding the nuances of each approach is essential for architects and developers as they navigate the architectural maze and make informed decisions about the best fit for their specific use cases and organizational contexts.
For further reading on this topic, check out the following resources:
- Martin Fowler's Blog on Microservices: martinfowler.com/microservices
- InfoQ's Articles on Service-Oriented Architecture: infoq.com/service-oriented-architecture
Happy architecting!